Quick start
Contents
Quick start¶
This section is a quick start guide that will show the basic functionality of McStasScript. It assumes the user is already familiar with McStas itself, if this is not the case, it is recommended to start with the tutorial which can serve as an introduction to both McStas and McStasScript.
Importing the package¶
McStasScript needs to be imported into the users python environment.
import mcstasscript as ms
Creating the first instrument object¶
Now the package can be used. Start with creating a new instrument, just needs a name. For a McXtrace instrument use McXtrace_instr instead.
instrument = ms.McStas_instr("first_instrument")
Finding a component¶
The instrument object loads the available McStas components, so it can show these in order to help the user.
instrument.available_components()
Here are the available component categories:
contrib
misc
monitors
obsolete
optics
samples
sources
union
Call available_components(category_name) to display
instrument.available_components("sources")
Here are all components in the sources category.
Adapt_check Moderator Source_Optimizer Source_gen
ESS_butterfly Monitor_Optimizer Source_adapt Source_simple
ESS_moderator Source_Maxwell_3 Source_div
Adding the first component¶
McStas components can be added to the instrument, here we add a source and ask for help on the parameters.
source = instrument.add_component("source", "Source_simple")
source.show_parameters()
___ Help Source_simple _____________________________________________________________
|optional parameter|required parameter|default value|user specified value|
radius = 0.1 [m] // Radius of circle in (x,y,0) plane where neutrons are
generated.
yheight = 0.0 [m] // Height of rectangle in (x,y,0) plane where neutrons are
generated.
xwidth = 0.0 [m] // Width of rectangle in (x,y,0) plane where neutrons are
generated.
dist = 0.0 [m] // Distance to target along z axis.
focus_xw = 0.045 [m] // Width of target
focus_yh = 0.12 [m] // Height of target
E0 = 0.0 [meV] // Mean energy of neutrons.
dE = 0.0 [meV] // Energy half spread of neutrons (flat or gaussian sigma).
lambda0 = 0.0 [AA] // Mean wavelength of neutrons.
dlambda = 0.0 [AA] // Wavelength half spread of neutrons.
flux = 1.0 [1/(s*cm**2*st*energy unit)] // flux per energy unit, Angs or meV if
flux=0, the source emits 1 in 4*PI whole
space.
gauss = 0.0 [1] // Gaussian (1) or Flat (0) energy/wavelength distribution
target_index = 1 [1] // relative index of component to focus at, e.g. next is
+1 this is used to compute 'dist' automatically.
-------------------------------------------------------------------------------------
Set parameters¶
The parameters of the component object are adjustable directly through the attributes of the object.
source.xwidth = 0.03
source.yheight = 0.03
source.lambda0 = 3
source.dlambda = 2.2
source.dist = 5
source.focus_xw = 0.01
source.focus_yh = 0.01
print(source)
COMPONENT source = Source_simple(
yheight = 0.03, // [m]
xwidth = 0.03, // [m]
dist = 5, // [m]
focus_xw = 0.01, // [m]
focus_yh = 0.01, // [m]
lambda0 = 3, // [AA]
dlambda = 2.2 // [AA]
)
AT (0, 0, 0) ABSOLUTE
Instrument parameters¶
It is possible to add instrument parameters that can be adjusted when running the simulation or adjusted using the widget interface.
wavelength = instrument.add_parameter("wavelength", value=3, comment="Wavelength in AA")
source.lambda0 = wavelength
source.dlambda = "0.1*wavelength"
print(source)
COMPONENT source = Source_simple(
yheight = 0.03, // [m]
xwidth = 0.03, // [m]
dist = 5, // [m]
focus_xw = 0.01, // [m]
focus_yh = 0.01, // [m]
lambda0 = wavelength, // [AA]
dlambda = 0.1*wavelength // [AA]
)
AT (0, 0, 0) ABSOLUTE
Inserting a sample component¶
A sample component is added as any other component, but here we place it relative to the source. A SANS sample is used, it focuses to a detector (chosen with target_index) with a width of focus_xw and height of focus_yh.
sample = instrument.add_component("sans_sample", "Sans_spheres")
sample.set_AT(5, RELATIVE=source)
sample.set_parameters(R=120, xwidth=0.01, yheight=0.01, zdepth=0.01,
target_index=1, focus_xw=0.5, focus_yh=0.5)
print(sample)
COMPONENT sans_sample = Sans_spheres(
R = 120, // [AA]
xwidth = 0.01, // [m]
yheight = 0.01, // [m]
zdepth = 0.01, // [m]
target_index = 1, // [1]
focus_xw = 0.5, // [m]
focus_yh = 0.5 // [m]
)
AT (0, 0, 5) RELATIVE source
Adding a monitor¶
The monitor can be placed relative to the sample, and even use the attributes from the sample to define its size so that the two always match. When setting a filename, it has to be a string also in the generated code, so use double quotation marks as shown here.
PSD = instrument.add_component("PSD", "PSD_monitor")
PSD.set_AT([0, 0, 5], RELATIVE=sample)
PSD.set_parameters(xwidth=sample.focus_xw, yheight=sample.focus_yh, filename='"PSD.dat"')
Setting up the simulation¶
The instrument now contains a source, a sample and a monitor, this is enough for a simple demonstration.
instrument.show_parameters()
wavelength = 3 // Wavelength in AA
instrument.set_parameters(wavelength=4)
instrument.settings(ncount=2E6)
Performing the simulation¶
In order to start the simulation the backengine method is called. If the simulation is successful, the data will be returned, otherwise the method returns None.
data = instrument.backengine()
INFO: Using directory: "/Users/madsbertelsen/PaNOSC/McStasScript/github/McStasScript/docs/source/getting_started/first_instrument_data_31"
INFO: Regenerating c-file: first_instrument.c
CFLAGS=
INFO: Recompiling: ./first_instrument.out
mccode-r.c:2837:3: warning: expression result unused [-Wunused-value]
*t0;
^~~
1 warning generated.
INFO: ===
Detector: PSD_I=2.96498e-31 PSD_ERR=1.93649e-33 PSD_N=1.99994e+06 "PSD.dat"
INFO: Placing instr file copy first_instrument.instr in dataset /Users/madsbertelsen/PaNOSC/McStasScript/github/McStasScript/docs/source/getting_started/first_instrument_data_31
loading system configuration
Plot the data¶
The data can be plotted with the make_sub_plot function from the plotter module.
ms.make_sub_plot(data, log=True)
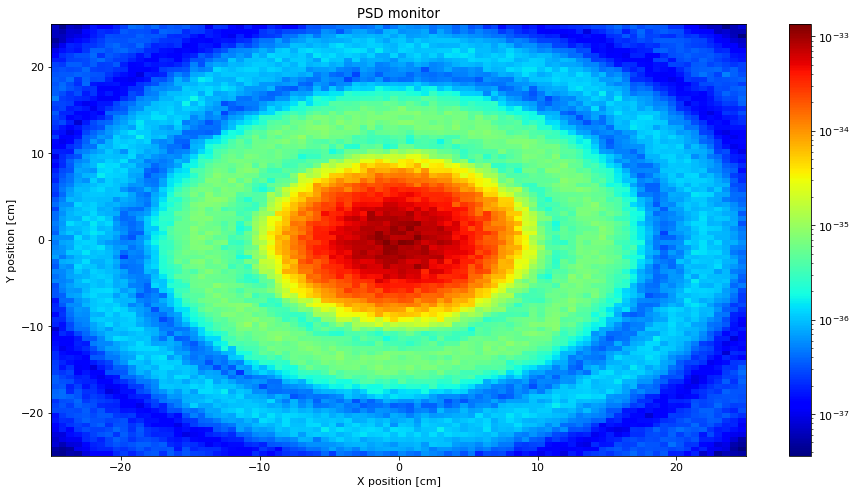
Access the data¶
The data is a list of McStasData objects and can be accessed directly.
print(data)
[
McStasData: PSD type: 2D I:2.96498e-31 E:1.93649e-33 N:1999940.0]
It is possible to search through the data list with the name_search function to retrieve the desired data object.
PSD_data = ms.name_search("PSD", data)
The intensities can then be accessed directly, along with Error and Ncount.
PSD_data.Intensity
array([[4.50464830e-38, 4.36558420e-38, 5.61904357e-38, ...,
6.01111507e-38, 5.45197742e-38, 5.22052232e-38],
[5.18223516e-38, 4.57536406e-38, 5.02234579e-38, ...,
8.62563460e-38, 4.82721955e-38, 3.60415103e-38],
[7.95847547e-38, 6.06906667e-38, 8.33089979e-38, ...,
9.67272539e-38, 5.62381583e-38, 4.66891970e-38],
...,
[6.42325626e-38, 6.59414237e-38, 8.26777785e-38, ...,
8.82298693e-38, 6.18833317e-38, 3.72700668e-38],
[6.66705788e-38, 5.51159631e-38, 8.20056630e-38, ...,
7.80880572e-38, 5.91312336e-38, 5.96067117e-38],
[6.85500254e-38, 4.22729029e-38, 6.69483563e-38, ...,
4.06725312e-38, 6.10111567e-38, 8.14837217e-38]])
Metadata is also available as a dict.
info_dict = PSD_data.metadata.info
for field, info in info_dict.items():
print(field, ":", info)
Date : Tue Apr 25 14:57:53 2023 (1682427473)
type : array_2d(90, 90)
Source : first_instrument (first_instrument.instr)
component : PSD
position : 0 0 10
title : PSD monitor
Ncount : 2000000
filename : PSD.dat
statistics : X0=-0.00405737; dX=4.17141; Y0=0.0344194; dY=4.16099;
signal : Min=3.60415e-38; Max=1.36659e-33; Mean=3.66047e-35;
values : 2.96498e-31 1.93649e-33 1.99994e+06
xvar : X
yvar : Y
xlabel : X position [cm]
ylabel : Y position [cm]
zvar : I
zlabel : Signal per bin
xylimits : -25 25 -25 25
variables : I I_err N
Parameters : {'wavelength': 4.0}