Union tagging system
Contents
Union tagging system¶
The Union_master is capable of recording histories for each neutron in a tree like fashion and add the total intensities for each unique history together. At the end of the simulation these are sorted by intensity and written to file, and the top 20 are shown in the terminal. This system does not work with MPI, if MPI is used only part of the data is written to disk. The system can take up a large amount of memory when used, so it is disabled per default.
First we set up a simple instrument with sample, container and a layer of cryostat.
import mcstasscript as ms
instrument = ms.McStas_instr("python_tutorial", input_path="run_folder")
Al_inc = instrument.add_component("Al_inc", "Incoherent_process")
Al_inc.sigma = 0.0082
Al_inc.unit_cell_volume = 66.4
Al_pow = instrument.add_component("Al_pow", "Powder_process")
Al_pow.reflections = '"Al.laz"'
Al = instrument.add_component("Al", "Union_make_material")
Al.process_string = '"Al_inc,Al_pow"'
Al.my_absorption = 100*0.231/66.4 # barns [m^2 E-28]*Å^3 [m^3 E-30]=[m E-2], factor 100
Sample_inc = instrument.add_component("Sample_inc", "Incoherent_process")
Sample_inc.sigma = 3.4176
Sample_inc.unit_cell_volume = 1079.1
Sample_pow = instrument.add_component("Sample_pow", "Powder_process")
Sample_pow.reflections = '"Na2Ca3Al2F14.laz"'
Sample = instrument.add_component("Sample", "Union_make_material")
Sample.process_string = '"Sample_inc,Sample_pow"'
Sample.my_absorption = 100*2.9464/1079.1
src = instrument.add_component("source", "Source_div")
src.xwidth = 0.01
src.yheight = 0.035
src.focus_aw = 0.01
src.focus_ah = 0.01
src.lambda0 = instrument.add_parameter("wavelength", value=5.0,
comment="Wavelength in [Ang]")
src.dlambda = "0.01*wavelength"
src.flux = 1E13
sample_geometry = instrument.add_component("sample_geometry", "Union_cylinder")
sample_geometry.yheight = 0.03
sample_geometry.radius = 0.0075
sample_geometry.material_string='"Sample"'
sample_geometry.priority = 100
sample_geometry.set_AT([0,0,1], RELATIVE=src)
container = instrument.add_component("sample_container", "Union_cylinder")
container.set_RELATIVE(sample_geometry)
container.yheight = 0.03+0.003 # 1.5 mm top and button
container.radius = 0.0075 + 0.0015 # 1.5 mm sides of container
container.material_string='"Al"'
container.priority = 99
inner_wall = instrument.add_component("cryostat_wall", "Union_cylinder")
inner_wall.set_AT([0,0,0], RELATIVE=sample_geometry)
inner_wall.yheight = 0.12
inner_wall.radius = 0.03
inner_wall.material_string='"Al"'
inner_wall.priority = 80
inner_wall_vac = instrument.add_component("cryostat_wall_vacuum", "Union_cylinder")
inner_wall_vac.set_AT([0,0,0], RELATIVE=sample_geometry)
inner_wall_vac.yheight = 0.12 - 0.008
inner_wall_vac.radius = 0.03 - 0.002
inner_wall_vac.material_string='"Vacuum"'
inner_wall_vac.priority = 81
logger_zx = instrument.add_component("logger_space_zx", "Union_logger_2D_space")
logger_zx.set_RELATIVE(sample_geometry)
logger_zx.D_direction_1 = '"z"'
logger_zx.D1_min = -0.04
logger_zx.D1_max = 0.04
logger_zx.n1 = 300
logger_zx.D_direction_2 = '"x"'
logger_zx.D2_min = -0.04
logger_zx.D2_max = 0.04
logger_zx.n2 = 300
logger_zx.filename = '"logger_zx.dat"'
logger_zy = instrument.add_component("logger_space_zy", "Union_logger_2D_space")
logger_zy.set_RELATIVE(sample_geometry)
logger_zy.D_direction_1 = '"z"'
logger_zy.D1_min = -0.04
logger_zy.D1_max = 0.04
logger_zy.n1 = 300
logger_zy.D_direction_2 = '"y"'
logger_zy.D2_min = -0.06
logger_zy.D2_max = 0.06
logger_zy.n2 = 300
logger_zy.filename = '"logger_zy.dat"'
Adding the Union_master with tagging¶
There are two important parameters to consider when setting a Union_master up for tagging:
enable_tagging [default 0] 0 for disable, 1 for enable
history_limit [default 300000] Limit of unique histories recorded
As the Union_master component records each ray in succession, their unique history is added to the history tree. If a ray takes the same path in the tree, the intensity gets added to that unique history. When the history limit is reached, the tree is not built out further, but if already recorded histories occur, their intensity is still added to the existing tree.
master = instrument.add_component("master", "Union_master")
master.enable_tagging = 1
master.history_limit = 300000
instrument.set_parameters(wavelength=3.0)
instrument.settings(ncount=3E5, output_path="data_folder/union_tagging")
data = instrument.backengine()
INFO: Using directory: "/Users/madsbertelsen/PaNOSC/McStasScript/github/McStasScript/docs/source/tutorial/data_folder/union_tagging_1"
INFO: Regenerating c-file: python_tutorial.c
CFLAGS= -I@MCCODE_LIB@/share/ -I@MCCODE_LIB@/share/
INFO: Recompiling: ./python_tutorial.out
mccode-r.c:2837:3: warning: expression result unused [-Wunused-value]
*t0;
^~~
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1604:105: warning: incompatible function pointer types passing 'int (const struct saved_history_struct *, const struct saved_history_struct *)' to parameter of type 'int (* _Nonnull)(const void *, const void *)' [-Wincompatible-function-pointer-types]
qsort(total_history.saved_histories,total_history.used_elements,sizeof (struct saved_history_struct), Sample_compare_history_intensities);
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/stdlib.h:161:22: note: passing argument to parameter '__compar' here
int (* _Nonnull __compar)(const void *, const void *));
^
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1613:20: warning: incompatible pointer types passing 'struct saved_history_struct *' to parameter of type 'struct dynamic_history_list *' [-Wincompatible-pointer-types]
printf_history(&total_history.saved_histories[history_iterate]);
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1434:50: note: passing argument to parameter 'history' here
void printf_history(struct dynamic_history_list *history) {
^
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:2030:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:839:1: warning: non-void function does not return a value in all control paths [-Wreturn-type]
};
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:883:1: warning: non-void function does not return a value [-Wreturn-type]
};
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3274:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3274:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3276:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3276:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3278:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3278:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3280:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3280:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:12948:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zx_filename
^~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:12948:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zx_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:13191:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zy_filename
^~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:13191:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zy_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:788:15: warning: left operand of comma operator has no effect [-Wunused-value]
if (volume_index_main,Volumes[volume_index_main]->geometry.is_mask_volume == 0 ||
^~~~~~~~~~~~~~~~~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:788:90: warning: left operand of comma operator has no effect [-Wunused-value]
if (volume_index_main,Volumes[volume_index_main]->geometry.is_mask_volume == 0 ||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~^~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:789:92: warning: left operand of comma operator has no effect [-Wunused-value]
volume_index_main,Volumes[volume_index_main]->geometry.is_masked_volume == 0 ||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~^~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
14 warnings generated.
INFO: ===
Opening input file '/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//data/Al.laz' (Table_Read_Offset)
Table from file 'Al.laz' (block 1) is 26 x 18 (x=1:8), constant step. interpolation: linear
'# TITLE *Aluminum-Al-[FM3-M] Miller, H.P.jr.;DuMond, J.W.M.[1942] at 298 K; ...'
PowderN: Al_pow: Reading 26 rows from Al.laz
PowderN: Al_pow: Read 26 reflections from file 'Al.laz'
PowderN: Al_pow: Vc=66.4 [Angs] sigma_abs=0.924 [barn] sigma_inc=0.0328 [barn] reflections=Al.laz
Opening input file '/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//data/Na2Ca3Al2F14.laz' (Table_Read_Offset)
Table from file 'Na2Ca3Al2F14.laz' (block 1) is 841 x 18 (x=1:20), constant step. interpolation: linear
'# TITLE *-Na2Ca3Al2F14-[I213] Courbion, G.;Ferey, G.[1988] Standard NAC cal ...'
PowderN: Sample_pow: Reading 841 rows from Na2Ca3Al2F14.laz
PowderN: Sample_pow: Read 841 reflections from file 'Na2Ca3Al2F14.laz'
PowderN: Sample_pow: Vc=1079.1 [Angs] sigma_abs=11.7856 [barn] sigma_inc=13.6704 [barn] reflections=Na2Ca3Al2F14.laz
---------------------------------------------------------------------
global_process_list.num_elements: 4
name of process [0]: Al_inc
component index [0]: 1
name of process [1]: Al_pow
component index [1]: 2
name of process [2]: Sample_inc
component index [2]: 4
name of process [3]: Sample_pow
component index [3]: 5
---------------------------------------------------------------------
global_material_list.num_elements: 2
name of material [0]: Al
component index [0]: 3
my_absoprtion [0]: 0.347892
number of processes [0]: 2
name of material [1]: Sample
component index [1]: 6
my_absoprtion [1]: 0.273042
number of processes [1]: 2
---------------------------------------------------------------------
global_geometry_list.num_elements: 2
name of geometry [0]: sample_geometry
component index [0]: 8
Volume.name [0]: sample_geometry
Volume.p_physics.is_vacuum [0]: 0
Volume.p_physics.my_absorption [0]: 0.273042
Volume.p_physics.number of processes [0]: 2
Volume.geometry.shape [0]: cylinder
Volume.geometry.center.x [0]: 0.000000
Volume.geometry.center.y [0]: 0.000000
Volume.geometry.center.z [0]: 1.000000
Volume.geometry.rotation_matrix[0] [0]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [0]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [0]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [0]: 0.007500
Volume.geometry.geometry_parameters.height [0]: 0.030000
Volume.geometry.focus_data_array.elements[0].Aim [0]: [0.000000 0.000000 1.000000]
name of geometry [1]: sample_container
component index [1]: 9
Volume.name [1]: sample_container
Volume.p_physics.is_vacuum [1]: 0
Volume.p_physics.my_absorption [1]: 0.347892
Volume.p_physics.number of processes [1]: 2
Volume.geometry.shape [1]: cylinder
Volume.geometry.center.x [1]: 0.000000
Volume.geometry.center.y [1]: 0.000000
Volume.geometry.center.z [1]: 1.000000
Volume.geometry.rotation_matrix[0] [1]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [1]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [1]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [1]: 0.009000
Volume.geometry.geometry_parameters.height [1]: 0.033000
Volume.geometry.focus_data_array.elements[0].Aim [1]: [0.000000 0.000000 1.000000]
name of geometry [2]: cryostat_wall
component index [2]: 10
Volume.name [2]: cryostat_wall
Volume.p_physics.is_vacuum [2]: 0
Volume.p_physics.my_absorption [2]: 0.347892
Volume.p_physics.number of processes [2]: 2
Volume.geometry.shape [2]: cylinder
Volume.geometry.center.x [2]: 0.000000
Volume.geometry.center.y [2]: 0.000000
Volume.geometry.center.z [2]: 1.000000
Volume.geometry.rotation_matrix[0] [2]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [2]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [2]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [2]: 0.030000
Volume.geometry.geometry_parameters.height [2]: 0.120000
Volume.geometry.focus_data_array.elements[0].Aim [2]: [0.000000 0.000000 1.000000]
name of geometry [3]: cryostat_wall_vacuum
component index [3]: 11
Volume.name [3]: cryostat_wall_vacuum
Volume.p_physics.is_vacuum [3]: 1
Volume.p_physics.my_absorption [3]: 0.000000
Volume.p_physics.number of processes [3]: 0
Volume.geometry.shape [3]: cylinder
Volume.geometry.center.x [3]: 0.000000
Volume.geometry.center.y [3]: 0.000000
Volume.geometry.center.z [3]: 1.000000
Volume.geometry.rotation_matrix[0] [3]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [3]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [3]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [3]: 0.028000
Volume.geometry.geometry_parameters.height [3]: 0.112000
Volume.geometry.focus_data_array.elements[0].Aim [3]: [0.000000 0.000000 1.000000]
---------------------------------------------------------------------
number_of_volumes = 5
number_of_masks = 0
number_of_masked_volumes = 0
---- Overview of the lists generated for each volume ----
List overview for surrounding vacuum
LIST: Children for Volume 0 = [1,2,3,4]
LIST: Direct_children for Volume 0 = [3]
LIST: Intersect_check_list for Volume 0 = [3]
LIST: Mask_intersect_list for Volume 0 = []
LIST: Destinations_list for Volume 0 = []
LIST: Reduced_destinations_list for Volume 0 = []
LIST: Next_volume_list for Volume 0 = [3]
LIST: mask_list for Volume 0 = []
LIST: masked_by_list for Volume 0 = []
LIST: masked_by_mask_index_list for Volume 0 = []
mask_mode for Volume 0 = 0
List overview for sample_geometry with cylinder shape made of Sample
LIST: Children for Volume 1 = []
LIST: Direct_children for Volume 1 = []
LIST: Intersect_check_list for Volume 1 = []
LIST: Mask_intersect_list for Volume 1 = []
LIST: Destinations_list for Volume 1 = [2]
LIST: Reduced_destinations_list for Volume 1 = [2]
LIST: Next_volume_list for Volume 1 = [2]
Is_vacuum for Volume 1 = 0
is_mask_volume for Volume 1 = 0
is_masked_volume for Volume 1 = 0
is_exit_volume for Volume 1 = 0
LIST: mask_list for Volume 1 = []
LIST: masked_by_list for Volume 1 = []
LIST: masked_by_mask_index_list for Volume 1 = []
mask_mode for Volume 1 = 0
List overview for sample_container with cylinder shape made of Al
LIST: Children for Volume 2 = [1]
LIST: Direct_children for Volume 2 = [1]
LIST: Intersect_check_list for Volume 2 = [1]
LIST: Mask_intersect_list for Volume 2 = []
LIST: Destinations_list for Volume 2 = [4]
LIST: Reduced_destinations_list for Volume 2 = [4]
LIST: Next_volume_list for Volume 2 = [4,1]
Is_vacuum for Volume 2 = 0
is_mask_volume for Volume 2 = 0
is_masked_volume for Volume 2 = 0
is_exit_volume for Volume 2 = 0
LIST: mask_list for Volume 2 = []
LIST: masked_by_list for Volume 2 = []
LIST: masked_by_mask_index_list for Volume 2 = []
mask_mode for Volume 2 = 0
List overview for cryostat_wall with cylinder shape made of Al
LIST: Children for Volume 3 = [1,2,4]
LIST: Direct_children for Volume 3 = [4]
LIST: Intersect_check_list for Volume 3 = [4]
LIST: Mask_intersect_list for Volume 3 = []
LIST: Destinations_list for Volume 3 = [0]
LIST: Reduced_destinations_list for Volume 3 = []
LIST: Next_volume_list for Volume 3 = [0,4]
Is_vacuum for Volume 3 = 0
is_mask_volume for Volume 3 = 0
is_masked_volume for Volume 3 = 0
is_exit_volume for Volume 3 = 0
LIST: mask_list for Volume 3 = []
LIST: masked_by_list for Volume 3 = []
LIST: masked_by_mask_index_list for Volume 3 = []
mask_mode for Volume 3 = 0
List overview for cryostat_wall_vacuum with cylinder shape made of Vacuum
LIST: Children for Volume 4 = [1,2]
LIST: Direct_children for Volume 4 = [2]
LIST: Intersect_check_list for Volume 4 = [2]
LIST: Mask_intersect_list for Volume 4 = []
LIST: Destinations_list for Volume 4 = [3]
LIST: Reduced_destinations_list for Volume 4 = [3]
LIST: Next_volume_list for Volume 4 = [3,2]
Is_vacuum for Volume 4 = 1
is_mask_volume for Volume 4 = 0
is_masked_volume for Volume 4 = 0
is_exit_volume for Volume 4 = 0
LIST: mask_list for Volume 4 = []
LIST: masked_by_list for Volume 4 = []
LIST: masked_by_mask_index_list for Volume 4 = []
mask_mode for Volume 4 = 0
Union_master component master initialized sucessfully
Detector: logger_space_zx_I=18057 logger_space_zx_ERR=100.838 logger_space_zx_N=87833 "logger_zx.dat"
Detector: logger_space_zy_I=18057 logger_space_zy_ERR=100.838 logger_space_zy_N=87833 "logger_zy.dat"
Top 20 most common histories. Shows the index of volumes entered (VX), and the scattering processes (PX)
185775 N I=3.961321E+04 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> V0
50765 N I=1.053272E+04 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> V4 -> V3 -> V0
22847 N I=4.871716E+03 V0 -> V3 -> V4 -> V2 -> V4 -> V3 -> V0
16658 N I=3.552022E+03 V0 -> V3 -> V4 -> V3 -> V0
6996 N I=1.477955E+03 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> V2 -> V4 -> V3 -> V0
3112 N I=5.817059E+02 V0 -> V3 -> V4 -> V2 -> P1 -> V4 -> V3 -> V0
2365 N I=4.123603E+02 V0 -> V3 -> P1 -> V0
1792 N I=3.681478E+02 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> P1 -> V0
1410 N I=2.816975E+02 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> P1 -> V4 -> V3 -> V0
1248 N I=2.544674E+02 V0 -> V3 -> V4 -> V2 -> P1 -> V1 -> V2 -> V4 -> V3 -> V0
982 N I=2.289662E+02 V0 -> V3 -> P1 -> V4 -> V3 -> V0
1012 N I=1.878656E+02 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> P1 -> V2 -> V4 -> V3 -> V0
787 N I=1.640674E+02 V0 -> V3 -> V4 -> V2 -> V1 -> P0 -> V2 -> V4 -> V3 -> V0
450 N I=9.535642E+01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> P1 -> V4 -> V3 -> V0
520 N I=9.169734E+01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V0
385 N I=5.830942E+01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
245 N I=5.101584E+01 V0 -> V3 -> V4 -> V2 -> P1 -> V1 -> P1 -> V2 -> V4 -> V3 -> V0
277 N I=4.505622E+01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> P1 -> V1 -> V2 -> V4 -> V3 -> V0
184 N I=3.710373E+01 V0 -> V3 -> V4 -> V2 -> V4 -> V3 -> P1 -> V0
225 N I=3.698070E+01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
INFO: Placing instr file copy python_tutorial.instr in dataset /Users/madsbertelsen/PaNOSC/McStasScript/github/McStasScript/docs/source/tutorial/data_folder/union_tagging_1
loading system configuration
ms.name_plot_options("logger_space_zx", data, log=True, orders_of_mag=4)
ms.name_plot_options("logger_space_zy", data, log=True, orders_of_mag=4)
ms.make_sub_plot(data)
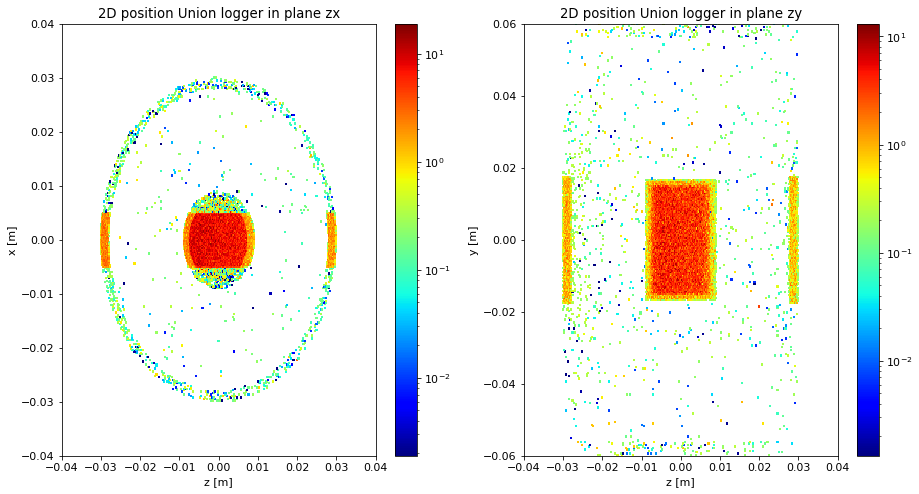
Finding the history file¶
A file called Union_history.dat is written in the run folder with all the unique histories. In some cases a bug happens that causes the file to be unreadable, for now the best fix is to rerun the simulation.
with open("run_folder/union_history.dat", "r", errors="ignore") as file:
instrument_string = file.read()
print(instrument_string)
History file written by the McStas component Union_master
When running with MPI, the results may be from just a single thread, meaning intensities are divided by number of threads
----- Description of the used volumes -----------------------------------------------------------------------------------
V0: Surrounding vacuum
V1: sample_geometry Material: Sample P0: P1: Sample_pow
V2: sample_container Material: Al P0: Al_inc P1: Al_pow
V3: cryostat_wall Material: Al P0: Al_inc P1: Al_pow
V4: cryostat_wall_vacuum Material: Vacuum
----- Histories sorted after intensity ----------------------------------------------------------------------------------
185775 N I=3.961321E+04 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> V0
50765 N I=1.053272E+04 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> V4 -> V3 -> V0
22847 N I=4.871716E+03 V0 -> V3 -> V4 -> V2 -> V4 -> V3 -> V0
16658 N I=3.552022E+03 V0 -> V3 -> V4 -> V3 -> V0
6996 N I=1.477955E+03 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> V2 -> V4 -> V3 -> V0
3112 N I=5.817059E+02 V0 -> V3 -> V4 -> V2 -> P1 -> V4 -> V3 -> V0
2365 N I=4.123603E+02 V0 -> V3 -> P1 -> V0
1792 N I=3.681478E+02 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> P1 -> V0
1410 N I=2.816975E+02 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> P1 -> V4 -> V3 -> V0
1248 N I=2.544674E+02 V0 -> V3 -> V4 -> V2 -> P1 -> V1 -> V2 -> V4 -> V3 -> V0
982 N I=2.289662E+02 V0 -> V3 -> P1 -> V4 -> V3 -> V0
1012 N I=1.878656E+02 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> P1 -> V2 -> V4 -> V3 -> V0
787 N I=1.640674E+02 V0 -> V3 -> V4 -> V2 -> V1 -> P0 -> V2 -> V4 -> V3 -> V0
450 N I=9.535642E+01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> P1 -> V4 -> V3 -> V0
520 N I=9.169734E+01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V0
385 N I=5.830942E+01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
245 N I=5.101584E+01 V0 -> V3 -> V4 -> V2 -> P1 -> V1 -> P1 -> V2 -> V4 -> V3 -> V0
277 N I=4.505622E+01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> P1 -> V1 -> V2 -> V4 -> V3 -> V0
184 N I=3.710373E+01 V0 -> V3 -> V4 -> V2 -> V4 -> V3 -> P1 -> V0
225 N I=3.698070E+01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
149 N I=3.106338E+01 V0 -> V3 -> V4 -> V3 -> P1 -> V0
162 N I=2.922490E+01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> P1 -> V1 -> V2 -> V4 -> V3 -> V0
144 N I=2.738158E+01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> P1 -> P1 -> V2 -> V4 -> V3 -> V0
113 N I=2.442939E+01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P0 -> V2 -> V4 -> V3 -> V0
92 N I=2.285098E+01 V0 -> V3 -> V4 -> V2 -> V1 -> P0 -> P1 -> V2 -> V4 -> V3 -> V0
104 N I=1.939182E+01 V0 -> V3 -> P1 -> P1 -> V0
73 N I=1.500160E+01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V0
63 N I=1.396520E+01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> V2 -> P1 -> V4 -> V3 -> V0
68 N I=1.244012E+01 V0 -> V3 -> P1 -> P1 -> V4 -> V3 -> V0
61 N I=9.330138E+00 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> P1 -> P1 -> V0
55 N I=9.255150E+00 V0 -> V3 -> V4 -> V2 -> P1 -> P1 -> V4 -> V3 -> V0
22 N I=8.055281E+00 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> P1 -> V1 -> P1 -> V2 -> V4 -> V3 -> V0
45 N I=7.116559E+00 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> P1 -> P1 -> V4 -> V3 -> V0
47 N I=6.454225E+00 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> P1 -> V1 -> P1 -> V2 -> V4 -> V3 -> V0
35 N I=6.162031E+00 V0 -> V3 -> V4 -> V2 -> P1 -> V4 -> V3 -> P1 -> V0
39 N I=5.906764E+00 V0 -> V3 -> V4 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
22 N I=5.620351E+00 V0 -> V3 -> V4 -> V2 -> P1 -> V1 -> P1 -> P1 -> V2 -> V4 -> V3 -> V0
36 N I=5.452127E+00 V0 -> V3 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
30 N I=5.275440E+00 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
27 N I=4.569823E+00 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> P1 -> P1 -> V4 -> V3 -> V0
19 N I=4.396805E+00 V0 -> V3 -> P1 -> V4 -> V3 -> P1 -> V0
13 N I=4.178755E+00 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P0 -> P1 -> V2 -> V4 -> V3 -> V0
27 N I=4.086861E+00 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> V2 -> P1 -> V1 -> V2 -> V4 -> V3 -> V0
26 N I=4.083722E+00 V0 -> V3 -> V4 -> V2 -> P1 -> P1 -> V1 -> V2 -> V4 -> V3 -> V0
26 N I=3.877217E+00 V0 -> V3 -> V4 -> V2 -> P1 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
18 N I=3.696686E+00 V0 -> V3 -> P1 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
18 N I=3.611148E+00 V0 -> V3 -> V4 -> V2 -> P1 -> V1 -> V2 -> V4 -> V3 -> P1 -> V0
15 N I=3.455205E+00 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> P0 -> V2 -> V4 -> V3 -> V0
15 N I=3.406859E+00 V0 -> V3 -> V4 -> V2 -> V1 -> P0 -> P1 -> P1 -> V2 -> V4 -> V3 -> V0
13 N I=3.374198E+00 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> P1 -> V2 -> P1 -> V4 -> V3 -> V0
17 N I=3.231731E+00 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> V4 -> V3 -> P1 -> P1 -> V0
16 N I=3.011303E+00 V0 -> V3 -> V4 -> V2 -> P1 -> V1 -> V2 -> P1 -> V4 -> V3 -> V0
16 N I=2.860067E+00 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> P1 -> V4 -> V3 -> P1 -> V0
14 N I=2.296279E+00 V0 -> V3 -> P1 -> P1 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> V0
9 N I=1.891707E+00 V0 -> V3 -> V4 -> V2 -> V1 -> P0 -> V2 -> V4 -> V3 -> P1 -> V0
1 N I=1.856563E+00 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> P1 -> P1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V0
7 N I=1.805719E+00 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> P1 -> V4 -> V3 -> P1 -> V0
9 N I=1.730843E+00 V0 -> V3 -> V4 -> V2 -> P0 -> V4 -> V3 -> V0
25 N I=1.541542E+00 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> P1 -> P1 -> P1 -> V2 -> V4 -> V3 -> V0
10 N I=1.539849E+00 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> P1 -> V0
2 N I=1.513959E+00 V0 -> V3 -> V4 -> V2 -> P1 -> V1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V0
9 N I=1.496076E+00 V0 -> V3 -> V4 -> V2 -> V4 -> V3 -> P1 -> P1 -> V0
8 N I=1.266179E+00 V0 -> V3 -> V4 -> V3 -> P1 -> P1 -> V0
7 N I=1.253851E+00 V0 -> V3 -> V4 -> V2 -> P1 -> V1 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
1 N I=1.240710E+00 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> P1 -> P1 -> P1 -> P1 -> P1 -> V2 -> V4 -> V3 -> V0
8 N I=1.150993E+00 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> P1 -> P1 -> V1 -> V2 -> V4 -> V3 -> V0
6 N I=1.128084E+00 V0 -> V3 -> V4 -> V2 -> V1 -> P0 -> V2 -> P1 -> V4 -> V3 -> V0
5 N I=1.125626E+00 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P0 -> P1 -> P1 -> V2 -> V4 -> V3 -> V0
5 N I=1.025901E+00 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> P1 -> P1 -> V4 -> V3 -> V0
5 N I=9.617506E-01 V0 -> V3 -> P0 -> V0
1 N I=9.214967E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> V2 -> P1 -> P1 -> V1 -> V2 -> V4 -> V3 -> V0
2 N I=8.790519E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> P1 -> V4 -> V3 -> P1 -> P1 -> V0
5 N I=8.603720E-01 V0 -> V3 -> V4 -> V2 -> P1 -> V1 -> V2 -> P1 -> V1 -> V2 -> V4 -> V3 -> V0
4 N I=8.322301E-01 V0 -> V3 -> V4 -> V2 -> P1 -> V1 -> P0 -> V2 -> V4 -> V3 -> V0
6 N I=8.197674E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
6 N I=7.580874E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> V4 -> V3 -> P1 -> P1 -> V4 -> V3 -> V0
1 N I=7.031758E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> P1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V0
3 N I=6.809638E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> P1 -> V0
8 N I=6.709230E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V0
4 N I=6.626793E-01 V0 -> V3 -> V4 -> V2 -> V4 -> V3 -> P1 -> P1 -> V4 -> V3 -> V0
1 N I=6.053246E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> V4 -> V3 -> P1 -> P1 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> V0
4 N I=5.986540E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> P1 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
3 N I=5.858165E-01 V0 -> V3 -> P1 -> V4 -> V3 -> P1 -> V4 -> V2 -> V4 -> V3 -> V0
4 N I=5.464585E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> P1 -> V1 -> V2 -> P1 -> V4 -> V3 -> V0
2 N I=5.368739E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P0 -> P1 -> V2 -> P1 -> V1 -> V2 -> V4 -> V3 -> V0
5 N I=5.343946E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
3 N I=5.323972E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> P1 -> P1 -> V4 -> V2 -> V1 -> P1 -> V2 -> V4 -> V3 -> V0
2 N I=4.953174E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P0 -> V2 -> V4 -> V3 -> P1 -> V0
4 N I=4.888856E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> P1 -> P1 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> V0
3 N I=4.824856E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> P1 -> V1 -> V2 -> V4 -> V3 -> P1 -> V0
4 N I=4.654932E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> P1 -> V2 -> P1 -> V1 -> V2 -> V4 -> V3 -> V0
3 N I=4.390844E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> P1 -> P1 -> V4 -> V2 -> V4 -> V3 -> V0
3 N I=4.389768E-01 V0 -> V3 -> V4 -> V3 -> P1 -> P1 -> V4 -> V3 -> V0
2 N I=4.075698E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P0 -> P0 -> V2 -> V4 -> V3 -> V0
2 N I=3.845838E-01 V0 -> V3 -> V4 -> V2 -> P0 -> V1 -> V2 -> V4 -> V3 -> V0
2 N I=3.845408E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> P0 -> V0
3 N I=3.810607E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> P1 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
3 N I=3.807561E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> P1 -> V1 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
2 N I=3.627377E-01 V0 -> V3 -> V4 -> V2 -> P1 -> V4 -> V3 -> P1 -> V4 -> V3 -> P1 -> V0
2 N I=3.495585E-01 V0 -> V3 -> V4 -> V2 -> P1 -> V1 -> P1 -> P1 -> P1 -> V2 -> V4 -> V3 -> V0
2 N I=3.465043E-01 V0 -> V3 -> P1 -> P0 -> V0
2 N I=3.374544E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> P1 -> V1 -> P1 -> P1 -> V2 -> V4 -> V3 -> V0
4 N I=3.189180E-01 V0 -> V3 -> V4 -> V2 -> P1 -> P1 -> V1 -> P1 -> V2 -> V4 -> V3 -> V0
3 N I=3.019891E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> P1 -> P1 -> V1 -> V2 -> V4 -> V3 -> V0
1 N I=2.815319E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> V4 -> V3 -> P1 -> P1 -> P1 -> V0
2 N I=2.734011E-01 V0 -> V3 -> V4 -> V3 -> P1 -> P1 -> V4 -> V2 -> V4 -> V3 -> V0
1 N I=2.106864E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> P0 -> P1 -> V0
1 N I=2.101911E-01 V0 -> V3 -> P1 -> P0 -> V4 -> V3 -> V0
1 N I=2.099214E-01 V0 -> V3 -> V4 -> V2 -> P1 -> P0 -> V4 -> V3 -> V0
3 N I=2.044396E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> P1 -> P1 -> V1 -> P1 -> V2 -> V4 -> V3 -> V0
1 N I=1.922969E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> P0 -> V4 -> V3 -> V0
1 N I=1.921659E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> P0 -> V4 -> V2 -> V4 -> V3 -> V0
2 N I=1.880022E-01 V0 -> V3 -> V4 -> V2 -> P1 -> V1 -> P1 -> V2 -> P1 -> V4 -> V3 -> V0
1 N I=1.878869E-01 V0 -> V3 -> V4 -> V2 -> P0 -> V1 -> P0 -> V2 -> V4 -> V3 -> V0
1 N I=1.816071E-01 V0 -> V3 -> V4 -> V2 -> P1 -> V4 -> V3 -> P1 -> P1 -> V4 -> V3 -> V0
1 N I=1.815289E-01 V0 -> V3 -> P1 -> P1 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
1 N I=1.815220E-01 V0 -> V3 -> V4 -> V2 -> P1 -> V1 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> P1 -> V0
1 N I=1.813974E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> P1 -> P1 -> V0
1 N I=1.812737E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> P1 -> P1 -> P1 -> V4 -> V3 -> V0
1 N I=1.812557E-01 V0 -> V3 -> V4 -> V2 -> P1 -> V1 -> V2 -> V4 -> V3 -> P1 -> P1 -> V0
1 N I=1.808547E-01 V0 -> V3 -> P1 -> P1 -> V4 -> V2 -> V1 -> V2 -> P1 -> V1 -> V2 -> V4 -> V3 -> V0
1 N I=1.806014E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> P1 -> V1 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
1 N I=1.805651E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> P1 -> P1 -> P1 -> V4 -> V3 -> V0
1 N I=1.655079E-01 V0 -> V3 -> P1 -> V4 -> V3 -> P1 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> V0
1 N I=1.653151E-01 V0 -> V3 -> V4 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
1 N I=1.621750E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> P1 -> V1 -> P0 -> V2 -> V4 -> V3 -> P1 -> V0
1 N I=1.621141E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P0 -> P1 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
1 N I=1.481087E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P0 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
1 N I=1.479883E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P0 -> V2 -> P1 -> V1 -> V2 -> V4 -> V3 -> V0
1 N I=1.444584E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> P1 -> V1 -> V2 -> P1 -> V4 -> V3 -> V0
1 N I=1.366483E-01 V0 -> V3 -> P0 -> V4 -> V3 -> P1 -> V0
1 N I=1.364144E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> P1 -> P0 -> V0
1 N I=1.363769E-01 V0 -> V3 -> P0 -> V4 -> V2 -> P1 -> V4 -> V3 -> V0
1 N I=1.289191E-01 V0 -> V3 -> V4 -> V3 -> P1 -> P1 -> P1 -> V4 -> V3 -> P1 -> V0
1 N I=1.235414E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> P1 -> V1 -> V2 -> P1 -> P1 -> V1 -> P1 -> V2 -> V4 -> V3 -> V0
2 N I=1.191198E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> P1 -> P1 -> P0 -> V2 -> V4 -> V3 -> V0
1 N I=1.179279E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> P1 -> P1 -> V4 -> V3 -> V0
1 N I=1.177898E-01 V0 -> V3 -> P1 -> P1 -> P1 -> V4 -> V3 -> V0
1 N I=1.176309E-01 V0 -> V3 -> V4 -> V2 -> P1 -> P1 -> V4 -> V3 -> P1 -> V0
1 N I=1.175791E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> P1 -> V1 -> V2 -> V4 -> V3 -> P1 -> P1 -> V0
4 N I=1.138856E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> V2 -> P1 -> V1 -> P1 -> V2 -> V4 -> V3 -> V0
2 N I=1.117987E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> P1 -> V1 -> P1 -> P1 -> P1 -> V2 -> V4 -> V3 -> V0
1 N I=1.076425E-01 V0 -> V3 -> V4 -> V3 -> P1 -> V4 -> V3 -> P1 -> V0
1 N I=1.075279E-01 V0 -> V3 -> V4 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> P1 -> V0
1 N I=1.075020E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> P1 -> V1 -> V2 -> V4 -> V3 -> P1 -> V0
3 N I=1.064300E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> V2 -> P1 -> P1 -> V4 -> V3 -> V0
1 N I=1.028607E-01 V0 -> V3 -> V4 -> V2 -> P0 -> V1 -> P1 -> V2 -> V4 -> V3 -> V0
1 N I=9.190556E-02 V0 -> V3 -> V4 -> V2 -> P1 -> P1 -> V1 -> P1 -> P1 -> P1 -> V2 -> V4 -> V3 -> V0
2 N I=8.985451E-02 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> P0 -> P1 -> P1 -> V2 -> V4 -> V3 -> V0
3 N I=7.858364E-02 V0 -> V3 -> P1 -> P1 -> V4 -> V2 -> V1 -> P1 -> V2 -> V4 -> V3 -> V0
1 N I=7.637808E-02 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> P1 -> P1 -> V1 -> V2 -> V4 -> V3 -> P1 -> V0
1 N I=7.623668E-02 V0 -> V3 -> V4 -> V2 -> P1 -> V4 -> V3 -> P1 -> P1 -> V0
2 N I=7.191051E-02 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P0 -> P1 -> P1 -> P1 -> V2 -> V4 -> V3 -> V0
2 N I=6.841978E-02 V0 -> V3 -> V4 -> V2 -> P1 -> V1 -> V2 -> P1 -> V1 -> P1 -> V2 -> V4 -> V3 -> V0
1 N I=6.239289E-02 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> P1 -> V1 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
1 N I=6.137751E-02 V0 -> V3 -> V4 -> V2 -> V1 -> P0 -> V2 -> P1 -> V1 -> P1 -> V2 -> V4 -> V3 -> V0
1 N I=6.131627E-02 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P0 -> V2 -> P1 -> V1 -> V2 -> V4 -> V3 -> V0
1 N I=5.927130E-02 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P0 -> V2 -> P1 -> V4 -> V3 -> V0
2 N I=5.653735E-02 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> P1 -> P1 -> P1 -> P1 -> V2 -> V4 -> V3 -> V0
2 N I=5.491441E-02 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> P1 -> V1 -> P1 -> V2 -> P1 -> V4 -> V3 -> V0
1 N I=5.037154E-02 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> P0 -> V4 -> V3 -> V0
1 N I=4.901831E-02 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> P1 -> V0
1 N I=4.679894E-02 V0 -> V3 -> V4 -> V2 -> V1 -> P0 -> P1 -> V2 -> V4 -> V3 -> P1 -> V0
1 N I=4.616141E-02 V0 -> V3 -> V4 -> V2 -> V1 -> P0 -> P1 -> P0 -> P1 -> V2 -> V4 -> V3 -> V0
2 N I=4.024895E-02 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> V2 -> V4 -> V3 -> P1 -> P1 -> V0
2 N I=3.862931E-02 V0 -> V3 -> V4 -> V2 -> P1 -> V1 -> P1 -> P0 -> V2 -> V4 -> V3 -> V0
1 N I=3.787663E-02 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> P1 -> V1 -> V2 -> P1 -> V1 -> P1 -> V2 -> V4 -> V3 -> V0
1 N I=3.184574E-02 V0 -> V3 -> V4 -> V2 -> P1 -> V1 -> P1 -> P1 -> P1 -> P1 -> V2 -> V4 -> V3 -> V0
2 N I=2.487289E-02 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> P1 -> V1 -> P1 -> P0 -> V2 -> V4 -> V3 -> V0
2 N I=2.045418E-02 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> P1 -> P0 -> V2 -> V4 -> V3 -> V0
2 N I=1.922082E-02 V0 -> V3 -> V4 -> V2 -> P1 -> V1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
1 N I=1.443613E-02 V0 -> V3 -> V4 -> V2 -> V1 -> P0 -> P1 -> V2 -> P1 -> V4 -> V3 -> V0
1 N I=8.234554E-03 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> V2 -> V4 -> V3 -> P1 -> P1 -> V4 -> V3 -> V0
2 N I=6.281062E-03 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> P1 -> V1 -> P1 -> P1 -> V2 -> V4 -> V3 -> V0
2 N I=1.821028E-03 V0 -> V3 -> V4 -> V2 -> V1 -> P0 -> P1 -> P1 -> P1 -> V2 -> V4 -> V3 -> V0
1 N I=9.262404E-04 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> P1 -> V2 -> P1 -> V1 -> V2 -> P1 -> V4 -> V3 -> V0
1 N I=2.178958E-04 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> P1 -> V1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V0
1 N I=1.088269E-04 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P0 -> P1 -> P1 -> P1 -> P1 -> V2 -> V4 -> V3 -> V0
1 N I=1.934077E-05 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> P0 -> P1 -> V2 -> V4 -> V3 -> V0
Interpreting the histories¶
The history with highest intensity in my run is: V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> V0, which means:
Neutron entered Volume0 (Surrounding vacuum)
Neutron entered Volume3 (Cryostat wall)
Neutron entered Volume4 (Cryostat vacuum)
Neutron entered Volume2 (Container)
Neutron entered Volume1 (Sample)
Neutron entered Volume2 (Container)
Neutron entered Volume4 (Cryostat vacuum)
Neutron entered Volume3 (Cryostat wall)
Neutron entered Volume0 (Surrounding vacuum)
So the most likely occurrence is that the ray is propagated through all geometries. The next most likely history is:
V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> V4 -> V3 -> V0
Neutron entered Volume0 (Surrounding vacuum)
Neutron entered Volume3 (Cryostat wall)
Neutron entered Volume4 (Cryostat vacuum)
Neutron entered Volume2 (Container)
Neutron entered Volume1 (Sample)
Neutron scattered on Process1 (Since we are in Volume1, that would be Sample_pow)
Neutron entered Volume2 (Container)
Neutron entered Volume4 (Cryostat vacuum)
Neutron entered Volume3 (Cryostat wall)
Neutron entered Volume0 (Surrounding vacuum)
Use conditional component to filter tagging¶
Just like a logger can be modified by a conditional component to only record events that satisfy that condition, the tagging system of the Union_master component can also be modified by a conditional component. In that case, the tagging system will only record events that satisfy the condition imposed by the conditional component.
This can be useful to explain an unexpected feature, as the conditional components can filter for energy, time, direction or any combination of these. Here we demonstrate this feature by adding a Union_conditional_PSD outside of the direct beam and enabling the master_tagging control parameter, which causes the condition to be applied to the tagging system.
# Set up an arm pointing to the relevant spot
spot_dir = instrument.add_component("spot_dir", "Arm", RELATIVE=sample_geometry,
before="master")
spot_dir.set_ROTATED([0, 60, 0], RELATIVE=sample_geometry)
# Set up a conditional component targeting all our loggers
PSD_conditional = instrument.add_component("space_all_PSD_conditional", "Union_conditional_PSD",
before="master")
PSD_conditional.xwidth = 0.2
PSD_conditional.yheight = 0.2
PSD_conditional.master_tagging = 1
PSD_conditional.set_AT([0, 0, 0.5], RELATIVE=spot_dir)
data = instrument.backengine()
INFO: Using directory: "/Users/madsbertelsen/PaNOSC/McStasScript/github/McStasScript/docs/source/tutorial/data_folder/union_tagging_2"
INFO: Regenerating c-file: python_tutorial.c
CFLAGS= -I@MCCODE_LIB@/share/ -I@MCCODE_LIB@/share/
INFO: Recompiling: ./python_tutorial.out
mccode-r.c:2837:3: warning: expression result unused [-Wunused-value]
*t0;
^~~
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1604:105: warning: incompatible function pointer types passing 'int (const struct saved_history_struct *, const struct saved_history_struct *)' to parameter of type 'int (* _Nonnull)(const void *, const void *)' [-Wincompatible-function-pointer-types]
qsort(total_history.saved_histories,total_history.used_elements,sizeof (struct saved_history_struct), Sample_compare_history_intensities);
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/stdlib.h:161:22: note: passing argument to parameter '__compar' here
int (* _Nonnull __compar)(const void *, const void *));
^
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1613:20: warning: incompatible pointer types passing 'struct saved_history_struct *' to parameter of type 'struct dynamic_history_list *' [-Wincompatible-pointer-types]
printf_history(&total_history.saved_histories[history_iterate]);
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1434:50: note: passing argument to parameter 'history' here
void printf_history(struct dynamic_history_list *history) {
^
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:2030:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:839:1: warning: non-void function does not return a value in all control paths [-Wreturn-type]
};
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:883:1: warning: non-void function does not return a value [-Wreturn-type]
};
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3274:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3274:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3276:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3276:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3278:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3278:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3280:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3280:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:13237:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zx_filename
^~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:13237:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zx_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:13480:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zy_filename
^~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:13480:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zy_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:788:15: warning: left operand of comma operator has no effect [-Wunused-value]
if (volume_index_main,Volumes[volume_index_main]->geometry.is_mask_volume == 0 ||
^~~~~~~~~~~~~~~~~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:788:90: warning: left operand of comma operator has no effect [-Wunused-value]
if (volume_index_main,Volumes[volume_index_main]->geometry.is_mask_volume == 0 ||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~^~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:789:92: warning: left operand of comma operator has no effect [-Wunused-value]
volume_index_main,Volumes[volume_index_main]->geometry.is_masked_volume == 0 ||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~^~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
14 warnings generated.
INFO: ===
Opening input file '/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//data/Al.laz' (Table_Read_Offset)
Table from file 'Al.laz' (block 1) is 26 x 18 (x=1:8), constant step. interpolation: linear
'# TITLE *Aluminum-Al-[FM3-M] Miller, H.P.jr.;DuMond, J.W.M.[1942] at 298 K; ...'
PowderN: Al_pow: Reading 26 rows from Al.laz
PowderN: Al_pow: Read 26 reflections from file 'Al.laz'
PowderN: Al_pow: Vc=66.4 [Angs] sigma_abs=0.924 [barn] sigma_inc=0.0328 [barn] reflections=Al.laz
Opening input file '/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//data/Na2Ca3Al2F14.laz' (Table_Read_Offset)
Table from file 'Na2Ca3Al2F14.laz' (block 1) is 841 x 18 (x=1:20), constant step. interpolation: linear
'# TITLE *-Na2Ca3Al2F14-[I213] Courbion, G.;Ferey, G.[1988] Standard NAC cal ...'
PowderN: Sample_pow: Reading 841 rows from Na2Ca3Al2F14.laz
PowderN: Sample_pow: Read 841 reflections from file 'Na2Ca3Al2F14.laz'
PowderN: Sample_pow: Vc=1079.1 [Angs] sigma_abs=11.7856 [barn] sigma_inc=13.6704 [barn] reflections=Na2Ca3Al2F14.laz
starded conditional initialize
---------------------------------------------------------------------
global_process_list.num_elements: 4
name of process [0]: Al_inc
component index [0]: 1
name of process [1]: Al_pow
component index [1]: 2
name of process [2]: Sample_inc
component index [2]: 4
name of process [3]: Sample_pow
component index [3]: 5
---------------------------------------------------------------------
global_material_list.num_elements: 2
name of material [0]: Al
component index [0]: 3
my_absoprtion [0]: 0.347892
number of processes [0]: 2
name of material [1]: Sample
component index [1]: 6
my_absoprtion [1]: 0.273042
number of processes [1]: 2
---------------------------------------------------------------------
global_geometry_list.num_elements: 2
name of geometry [0]: sample_geometry
component index [0]: 8
Volume.name [0]: sample_geometry
Volume.p_physics.is_vacuum [0]: 0
Volume.p_physics.my_absorption [0]: 0.273042
Volume.p_physics.number of processes [0]: 2
Volume.geometry.shape [0]: cylinder
Volume.geometry.center.x [0]: 0.000000
Volume.geometry.center.y [0]: 0.000000
Volume.geometry.center.z [0]: 1.000000
Volume.geometry.rotation_matrix[0] [0]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [0]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [0]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [0]: 0.007500
Volume.geometry.geometry_parameters.height [0]: 0.030000
Volume.geometry.focus_data_array.elements[0].Aim [0]: [0.000000 0.000000 1.000000]
name of geometry [1]: sample_container
component index [1]: 9
Volume.name [1]: sample_container
Volume.p_physics.is_vacuum [1]: 0
Volume.p_physics.my_absorption [1]: 0.347892
Volume.p_physics.number of processes [1]: 2
Volume.geometry.shape [1]: cylinder
Volume.geometry.center.x [1]: 0.000000
Volume.geometry.center.y [1]: 0.000000
Volume.geometry.center.z [1]: 1.000000
Volume.geometry.rotation_matrix[0] [1]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [1]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [1]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [1]: 0.009000
Volume.geometry.geometry_parameters.height [1]: 0.033000
Volume.geometry.focus_data_array.elements[0].Aim [1]: [0.000000 0.000000 1.000000]
name of geometry [2]: cryostat_wall
component index [2]: 10
Volume.name [2]: cryostat_wall
Volume.p_physics.is_vacuum [2]: 0
Volume.p_physics.my_absorption [2]: 0.347892
Volume.p_physics.number of processes [2]: 2
Volume.geometry.shape [2]: cylinder
Volume.geometry.center.x [2]: 0.000000
Volume.geometry.center.y [2]: 0.000000
Volume.geometry.center.z [2]: 1.000000
Volume.geometry.rotation_matrix[0] [2]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [2]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [2]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [2]: 0.030000
Volume.geometry.geometry_parameters.height [2]: 0.120000
Volume.geometry.focus_data_array.elements[0].Aim [2]: [0.000000 0.000000 1.000000]
name of geometry [3]: cryostat_wall_vacuum
component index [3]: 11
Volume.name [3]: cryostat_wall_vacuum
Volume.p_physics.is_vacuum [3]: 1
Volume.p_physics.my_absorption [3]: 0.000000
Volume.p_physics.number of processes [3]: 0
Volume.geometry.shape [3]: cylinder
Volume.geometry.center.x [3]: 0.000000
Volume.geometry.center.y [3]: 0.000000
Volume.geometry.center.z [3]: 1.000000
Volume.geometry.rotation_matrix[0] [3]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [3]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [3]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [3]: 0.028000
Volume.geometry.geometry_parameters.height [3]: 0.112000
Volume.geometry.focus_data_array.elements[0].Aim [3]: [0.000000 0.000000 1.000000]
---------------------------------------------------------------------
number_of_volumes = 5
number_of_masks = 0
number_of_masked_volumes = 0
---- Overview of the lists generated for each volume ----
List overview for surrounding vacuum
LIST: Children for Volume 0 = [1,2,3,4]
LIST: Direct_children for Volume 0 = [3]
LIST: Intersect_check_list for Volume 0 = [3]
LIST: Mask_intersect_list for Volume 0 = []
LIST: Destinations_list for Volume 0 = []
LIST: Reduced_destinations_list for Volume 0 = []
LIST: Next_volume_list for Volume 0 = [3]
LIST: mask_list for Volume 0 = []
LIST: masked_by_list for Volume 0 = []
LIST: masked_by_mask_index_list for Volume 0 = []
mask_mode for Volume 0 = 0
List overview for sample_geometry with cylinder shape made of Sample
LIST: Children for Volume 1 = []
LIST: Direct_children for Volume 1 = []
LIST: Intersect_check_list for Volume 1 = []
LIST: Mask_intersect_list for Volume 1 = []
LIST: Destinations_list for Volume 1 = [2]
LIST: Reduced_destinations_list for Volume 1 = [2]
LIST: Next_volume_list for Volume 1 = [2]
Is_vacuum for Volume 1 = 0
is_mask_volume for Volume 1 = 0
is_masked_volume for Volume 1 = 0
is_exit_volume for Volume 1 = 0
LIST: mask_list for Volume 1 = []
LIST: masked_by_list for Volume 1 = []
LIST: masked_by_mask_index_list for Volume 1 = []
mask_mode for Volume 1 = 0
List overview for sample_container with cylinder shape made of Al
LIST: Children for Volume 2 = [1]
LIST: Direct_children for Volume 2 = [1]
LIST: Intersect_check_list for Volume 2 = [1]
LIST: Mask_intersect_list for Volume 2 = []
LIST: Destinations_list for Volume 2 = [4]
LIST: Reduced_destinations_list for Volume 2 = [4]
LIST: Next_volume_list for Volume 2 = [4,1]
Is_vacuum for Volume 2 = 0
is_mask_volume for Volume 2 = 0
is_masked_volume for Volume 2 = 0
is_exit_volume for Volume 2 = 0
LIST: mask_list for Volume 2 = []
LIST: masked_by_list for Volume 2 = []
LIST: masked_by_mask_index_list for Volume 2 = []
mask_mode for Volume 2 = 0
List overview for cryostat_wall with cylinder shape made of Al
LIST: Children for Volume 3 = [1,2,4]
LIST: Direct_children for Volume 3 = [4]
LIST: Intersect_check_list for Volume 3 = [4]
LIST: Mask_intersect_list for Volume 3 = []
LIST: Destinations_list for Volume 3 = [0]
LIST: Reduced_destinations_list for Volume 3 = []
LIST: Next_volume_list for Volume 3 = [0,4]
Is_vacuum for Volume 3 = 0
is_mask_volume for Volume 3 = 0
is_masked_volume for Volume 3 = 0
is_exit_volume for Volume 3 = 0
LIST: mask_list for Volume 3 = []
LIST: masked_by_list for Volume 3 = []
LIST: masked_by_mask_index_list for Volume 3 = []
mask_mode for Volume 3 = 0
List overview for cryostat_wall_vacuum with cylinder shape made of Vacuum
LIST: Children for Volume 4 = [1,2]
LIST: Direct_children for Volume 4 = [2]
LIST: Intersect_check_list for Volume 4 = [2]
LIST: Mask_intersect_list for Volume 4 = []
LIST: Destinations_list for Volume 4 = [3]
LIST: Reduced_destinations_list for Volume 4 = [3]
LIST: Next_volume_list for Volume 4 = [3,2]
Is_vacuum for Volume 4 = 1
is_mask_volume for Volume 4 = 0
is_masked_volume for Volume 4 = 0
is_exit_volume for Volume 4 = 0
LIST: mask_list for Volume 4 = []
LIST: masked_by_list for Volume 4 = []
LIST: masked_by_mask_index_list for Volume 4 = []
mask_mode for Volume 4 = 0
Union_master component master initialized sucessfully
Detector: logger_space_zx_I=18164.3 logger_space_zx_ERR=102.047 logger_space_zx_N=88260 "logger_zx.dat"
Detector: logger_space_zy_I=18164.3 logger_space_zy_ERR=102.047 logger_space_zy_N=88260 "logger_zy.dat"
Top 20 most common histories. Shows the index of volumes entered (VX), and the scattering processes (PX)
606 N I=4.743002E+01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> V4 -> V3 -> V0
102 N I=2.206381E+01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> V2 -> V4 -> V3 -> V0
10 N I=2.386701E+00 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> P1 -> V2 -> V4 -> V3 -> V0
8 N I=1.667700E+00 V0 -> V3 -> V4 -> V2 -> V1 -> P0 -> V2 -> V4 -> V3 -> V0
9 N I=1.474406E+00 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V0
2 N I=1.352136E+00 V0 -> V3 -> V4 -> V2 -> P1 -> V1 -> P1 -> V2 -> V4 -> V3 -> V0
6 N I=9.219711E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> P1 -> V4 -> V3 -> V0
1 N I=7.634924E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P0 -> V2 -> V4 -> V3 -> V0
2 N I=3.960638E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
1 N I=3.269082E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
1 N I=2.694791E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V0
2 N I=2.643546E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> P1 -> V1 -> V2 -> V4 -> V3 -> V0
1 N I=2.553636E-01 V0 -> V3 -> V4 -> V3 -> P1 -> P1 -> V0
1 N I=2.552505E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> P1 -> P1 -> V0
1 N I=2.545248E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> P1 -> V4 -> V3 -> P1 -> V0
1 N I=1.997199E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V0
1 N I=1.658401E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> P1 -> P1 -> V4 -> V3 -> V0
1 N I=1.655725E-01 V0 -> V3 -> V4 -> V2 -> P1 -> V1 -> V2 -> P1 -> V4 -> V3 -> V0
1 N I=1.654906E-01 V0 -> V3 -> P1 -> P1 -> V4 -> V3 -> V0
1 N I=7.862257E-02 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
INFO: Placing instr file copy python_tutorial.instr in dataset /Users/madsbertelsen/PaNOSC/McStasScript/github/McStasScript/docs/source/tutorial/data_folder/union_tagging_2
loading system configuration
with open("run_folder/union_history.dat", "r", errors="ignore") as file:
instrument_string = file.read()
print(instrument_string)
History file written by the McStas component Union_master
When running with MPI, the results may be from just a single thread, meaning intensities are divided by number of threads
----- Description of the used volumes -----------------------------------------------------------------------------------
V0: Surrounding vacuum
V1: sample_geometry Material: Sample P0: Sample_inc P1: Sample_pow
V2: sample_container Material: Al P0: <W? P1: Al_pow
V3: cryostat_wall Material: Al P0: <W? P1: Al_pow
V4: cryostat_wall_vacuum Material: Vacuum
----- Histories sorted after intensity ----------------------------------------------------------------------------------
606 N I=4.743002E+01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> V4 -> V3 -> V0
102 N I=2.206381E+01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> V2 -> V4 -> V3 -> V0
10 N I=2.386701E+00 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> P1 -> V2 -> V4 -> V3 -> V0
8 N I=1.667700E+00 V0 -> V3 -> V4 -> V2 -> V1 -> P0 -> V2 -> V4 -> V3 -> V0
9 N I=1.474406E+00 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V0
2 N I=1.352136E+00 V0 -> V3 -> V4 -> V2 -> P1 -> V1 -> P1 -> V2 -> V4 -> V3 -> V0
6 N I=9.219711E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> P1 -> V4 -> V3 -> V0
1 N I=7.634924E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P0 -> V2 -> V4 -> V3 -> V0
2 N I=3.960638E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
1 N I=3.269082E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
1 N I=2.694791E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V0
2 N I=2.643546E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> V2 -> P1 -> V1 -> V2 -> V4 -> V3 -> V0
1 N I=2.553636E-01 V0 -> V3 -> V4 -> V3 -> P1 -> P1 -> V0
1 N I=2.552505E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> V4 -> V3 -> P1 -> P1 -> V0
1 N I=2.545248E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> P1 -> V4 -> V3 -> P1 -> V0
1 N I=1.997199E-01 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V0
1 N I=1.658401E-01 V0 -> V3 -> V4 -> V2 -> V1 -> V2 -> P1 -> P1 -> V4 -> V3 -> V0
1 N I=1.655725E-01 V0 -> V3 -> V4 -> V2 -> P1 -> V1 -> V2 -> P1 -> V4 -> V3 -> V0
1 N I=1.654906E-01 V0 -> V3 -> P1 -> P1 -> V4 -> V3 -> V0
1 N I=7.862257E-02 V0 -> V3 -> V4 -> V2 -> V1 -> P1 -> P1 -> V2 -> V4 -> V3 -> P1 -> V4 -> V3 -> V0
Interpreting the data¶
Now all the histories contain a scattering process as this is necessary to reach the rectangle placed by the Union_conditional_PSD component set at 2theta = 60 deg. We also observe the intensities are much lower, simply because only a fraction of the simulated rays satisfy this condition.