Advanced geometry component concepts: Exit geometry and number of activations
Contents
Advanced geometry component concepts: Exit geometry and number of activations¶
This notebook explains the concept of exit geometry and the activation counter both of which are tied to the geometry components and how they are treated by the Union_master.
An exit geometry is created by setting the material_string of a geometry to “Exit”, and if a ray enters such a geometry, it is immediately released from the master component. Normally this only happens when the ray does not intersect any geometries. There are several uses for this, for example inserting a monitor within a Union geometry ensemble.
import mcstasscript as ms
Set up an example with empty sample container¶
First we set up an example with an empty sample container.
instrument = ms.McStas_instr("python_tutorial", input_path="run_folder")
Al_inc = instrument.add_component("Al_inc", "Incoherent_process")
Al_inc.sigma = 0.0082
Al_inc.unit_cell_volume = 66.4
Al_pow = instrument.add_component("Al_pow", "Powder_process")
Al_pow.reflections = '"Al.laz"'
Al = instrument.add_component("Al", "Union_make_material")
Al.process_string = '"Al_inc,Al_pow"'
Al.my_absorption = 100*0.231/66.4 # barns [m^2 E-28]*Å^3 [m^3 E-30]=[m E-2], factor 100
src = instrument.add_component("source", "Source_div")
src.xwidth = 0.01
src.yheight = 0.035
src.focus_aw = 0.01
src.focus_ah = 0.01
src.lambda0 = instrument.add_parameter("wavelength", value=5.0,
comment="Wavelength in [Ang]")
src.dlambda = "0.01*wavelength"
src.flux = 1E13
sample_volume = instrument.add_component("sample_volume", "Union_cylinder")
sample_volume.yheight = 0.03
sample_volume.radius = 0.0075
sample_volume.material_string='"Vacuum"'
sample_volume.priority = 100
sample_volume.set_AT([0,0,1], RELATIVE=src)
container = instrument.add_component("sample_container", "Union_cylinder")
container.set_RELATIVE(sample_volume)
container.yheight = 0.03+0.003 # 1.5 mm top and button
container.radius = 0.0075 + 0.0015 # 1.5 mm sides of container
container.material_string='"Al"'
container.priority = 99
container_lid = instrument.add_component("sample_container_lid", "Union_cylinder")
container_lid.set_AT([0, 0.0155, 0], RELATIVE=container)
container_lid.yheight = 0.004
container_lid.radius = 0.013
container_lid.material_string='"Al"'
container_lid.priority = 98
inner_wall = instrument.add_component("cryostat_wall", "Union_cylinder")
inner_wall.set_AT([0,0,0], RELATIVE=sample_volume)
inner_wall.yheight = 0.12
inner_wall.radius = 0.03
inner_wall.material_string='"Al"'
inner_wall.priority = 80
inner_wall_vac = instrument.add_component("cryostat_wall_vacuum", "Union_cylinder")
inner_wall_vac.set_AT([0,0,0], RELATIVE=sample_volume)
inner_wall_vac.yheight = 0.12 - 0.008
inner_wall_vac.radius = 0.03 - 0.002
inner_wall_vac.material_string='"Vacuum"'
inner_wall_vac.priority = 81
logger_zx = instrument.add_component("logger_space_zx", "Union_logger_2D_space")
logger_zx.set_RELATIVE(sample_volume)
logger_zx.D_direction_1 = '"z"'
logger_zx.D1_min = -0.04
logger_zx.D1_max = 0.04
logger_zx.n1 = 300
logger_zx.D_direction_2 = '"x"'
logger_zx.D2_min = -0.04
logger_zx.D2_max = 0.04
logger_zx.n2 = 300
logger_zx.filename = '"logger_zx.dat"'
logger_zy = instrument.add_component("logger_space_zy", "Union_logger_2D_space")
logger_zy.set_RELATIVE(sample_volume)
logger_zy.D_direction_1 = '"z"'
logger_zy.D1_min = -0.04
logger_zy.D1_max = 0.04
logger_zy.n1 = 300
logger_zy.D_direction_2 = '"y"'
logger_zy.D2_min = -0.06
logger_zy.D2_max = 0.06
logger_zy.n2 = 300
logger_zy.filename = '"logger_zy.dat"'
master = instrument.add_component("master", "Union_master")
banana = instrument.add_component("banana", "Monitor_nD", RELATIVE=sample_volume)
banana.xwidth = 1.5
banana.yheight = 0.4
banana.restore_neutron = 1
banana.options = '"theta limits=[5 175] bins=250, banana"'
instrument.show_diagram()
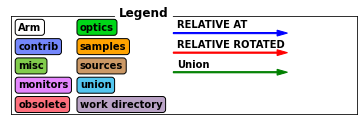
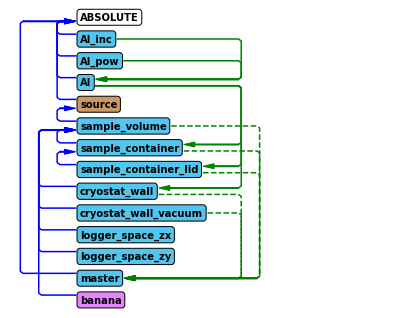
We see there is one material defined, Al, and 3 of the 5 geometries use this as their material. The 5 geometries are all picked up by the master component.
instrument.set_parameters(wavelength=3.0)
instrument.settings(ncount=3E6, output_path="data_folder/union_external")
data_empty = instrument.backengine()
INFO: Using directory: "/Users/madsbertelsen/PaNOSC/McStasScript/github/McStasScript/docs/source/tutorial/data_folder/union_external_3"
INFO: Regenerating c-file: python_tutorial.c
CFLAGS= -I@MCCODE_LIB@/share/ -I@MCCODE_LIB@/share/
INFO: Recompiling: ./python_tutorial.out
mccode-r.c:2837:3: warning: expression result unused [-Wunused-value]
*t0;
^~~
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1604:105: warning: incompatible function pointer types passing 'int (const struct saved_history_struct *, const struct saved_history_struct *)' to parameter of type 'int (* _Nonnull)(const void *, const void *)' [-Wincompatible-function-pointer-types]
qsort(total_history.saved_histories,total_history.used_elements,sizeof (struct saved_history_struct), Sample_compare_history_intensities);
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/stdlib.h:161:22: note: passing argument to parameter '__compar' here
int (* _Nonnull __compar)(const void *, const void *));
^
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1613:20: warning: incompatible pointer types passing 'struct saved_history_struct *' to parameter of type 'struct dynamic_history_list *' [-Wincompatible-pointer-types]
printf_history(&total_history.saved_histories[history_iterate]);
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1434:50: note: passing argument to parameter 'history' here
void printf_history(struct dynamic_history_list *history) {
^
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:2030:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:839:1: warning: non-void function does not return a value in all control paths [-Wreturn-type]
};
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:883:1: warning: non-void function does not return a value [-Wreturn-type]
};
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3274:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3274:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3276:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3276:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3278:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3278:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3280:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3280:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:14810:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zx_filename
^~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:14810:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zx_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:15053:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zy_filename
^~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:15053:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zy_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:788:15: warning: left operand of comma operator has no effect [-Wunused-value]
if (volume_index_main,Volumes[volume_index_main]->geometry.is_mask_volume == 0 ||
^~~~~~~~~~~~~~~~~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:788:90: warning: left operand of comma operator has no effect [-Wunused-value]
if (volume_index_main,Volumes[volume_index_main]->geometry.is_mask_volume == 0 ||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~^~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:789:92: warning: left operand of comma operator has no effect [-Wunused-value]
volume_index_main,Volumes[volume_index_main]->geometry.is_masked_volume == 0 ||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~^~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
14 warnings generated.
INFO: ===
Opening input file '/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//data/Al.laz' (Table_Read_Offset)
Table from file 'Al.laz' (block 1) is 26 x 18 (x=1:8), constant step. interpolation: linear
'# TITLE *Aluminum-Al-[FM3-M] Miller, H.P.jr.;DuMond, J.W.M.[1942] at 298 K; ...'
PowderN: Al_pow: Reading 26 rows from Al.laz
PowderN: Al_pow: Read 26 reflections from file 'Al.laz'
PowderN: Al_pow: Vc=66.4 [Angs] sigma_abs=0.924 [barn] sigma_inc=0.0328 [barn] reflections=Al.laz
---------------------------------------------------------------------
global_process_list.num_elements: 2
name of process [0]: Al_inc
component index [0]: 1
name of process [1]: Al_pow
component index [1]: 2
---------------------------------------------------------------------
global_material_list.num_elements: 1
name of material [0]: Al
component index [0]: 3
my_absoprtion [0]: 0.347892
number of processes [0]: 2
---------------------------------------------------------------------
global_geometry_list.num_elements: 1
name of geometry [0]: sample_volume
component index [0]: 5
Volume.name [0]: sample_volume
Volume.p_physics.is_vacuum [0]: 1
Volume.p_physics.my_absorption [0]: 0.000000
Volume.p_physics.number of processes [0]: 0
Volume.geometry.shape [0]: cylinder
Volume.geometry.center.x [0]: 0.000000
Volume.geometry.center.y [0]: 0.000000
Volume.geometry.center.z [0]: 1.000000
Volume.geometry.rotation_matrix[0] [0]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [0]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [0]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [0]: 0.007500
Volume.geometry.geometry_parameters.height [0]: 0.030000
Volume.geometry.focus_data_array.elements[0].Aim [0]: [0.000000 0.000000 1.000000]
name of geometry [1]: sample_container
component index [1]: 6
Volume.name [1]: sample_container
Volume.p_physics.is_vacuum [1]: 0
Volume.p_physics.my_absorption [1]: 0.347892
Volume.p_physics.number of processes [1]: 2
Volume.geometry.shape [1]: cylinder
Volume.geometry.center.x [1]: 0.000000
Volume.geometry.center.y [1]: 0.000000
Volume.geometry.center.z [1]: 1.000000
Volume.geometry.rotation_matrix[0] [1]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [1]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [1]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [1]: 0.009000
Volume.geometry.geometry_parameters.height [1]: 0.033000
Volume.geometry.focus_data_array.elements[0].Aim [1]: [0.000000 0.000000 1.000000]
name of geometry [2]: sample_container_lid
component index [2]: 7
Volume.name [2]: sample_container_lid
Volume.p_physics.is_vacuum [2]: 0
Volume.p_physics.my_absorption [2]: 0.347892
Volume.p_physics.number of processes [2]: 2
Volume.geometry.shape [2]: cylinder
Volume.geometry.center.x [2]: 0.000000
Volume.geometry.center.y [2]: 0.015500
Volume.geometry.center.z [2]: 1.000000
Volume.geometry.rotation_matrix[0] [2]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [2]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [2]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [2]: 0.013000
Volume.geometry.geometry_parameters.height [2]: 0.004000
Volume.geometry.focus_data_array.elements[0].Aim [2]: [0.000000 0.000000 1.000000]
name of geometry [3]: cryostat_wall
component index [3]: 8
Volume.name [3]: cryostat_wall
Volume.p_physics.is_vacuum [3]: 0
Volume.p_physics.my_absorption [3]: 0.347892
Volume.p_physics.number of processes [3]: 2
Volume.geometry.shape [3]: cylinder
Volume.geometry.center.x [3]: 0.000000
Volume.geometry.center.y [3]: 0.000000
Volume.geometry.center.z [3]: 1.000000
Volume.geometry.rotation_matrix[0] [3]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [3]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [3]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [3]: 0.030000
Volume.geometry.geometry_parameters.height [3]: 0.120000
Volume.geometry.focus_data_array.elements[0].Aim [3]: [0.000000 0.000000 1.000000]
name of geometry [4]: cryostat_wall_vacuum
component index [4]: 9
Volume.name [4]: cryostat_wall_vacuum
Volume.p_physics.is_vacuum [4]: 1
Volume.p_physics.my_absorption [4]: 0.000000
Volume.p_physics.number of processes [4]: 0
Volume.geometry.shape [4]: cylinder
Volume.geometry.center.x [4]: 0.000000
Volume.geometry.center.y [4]: 0.000000
Volume.geometry.center.z [4]: 1.000000
Volume.geometry.rotation_matrix[0] [4]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [4]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [4]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [4]: 0.028000
Volume.geometry.geometry_parameters.height [4]: 0.112000
Volume.geometry.focus_data_array.elements[0].Aim [4]: [0.000000 0.000000 1.000000]
---------------------------------------------------------------------
number_of_volumes = 6
number_of_masks = 0
number_of_masked_volumes = 0
---- Overview of the lists generated for each volume ----
List overview for surrounding vacuum
LIST: Children for Volume 0 = [1,2,3,4,5]
LIST: Direct_children for Volume 0 = [4]
LIST: Intersect_check_list for Volume 0 = [4]
LIST: Mask_intersect_list for Volume 0 = []
LIST: Destinations_list for Volume 0 = []
LIST: Reduced_destinations_list for Volume 0 = []
LIST: Next_volume_list for Volume 0 = [4]
LIST: mask_list for Volume 0 = []
LIST: masked_by_list for Volume 0 = []
LIST: masked_by_mask_index_list for Volume 0 = []
mask_mode for Volume 0 = 0
List overview for sample_volume with cylinder shape made of Vacuum
LIST: Children for Volume 1 = []
LIST: Direct_children for Volume 1 = []
LIST: Intersect_check_list for Volume 1 = []
LIST: Mask_intersect_list for Volume 1 = []
LIST: Destinations_list for Volume 1 = [2,3]
LIST: Reduced_destinations_list for Volume 1 = [2,3]
LIST: Next_volume_list for Volume 1 = [2,3]
Is_vacuum for Volume 1 = 1
is_mask_volume for Volume 1 = 0
is_masked_volume for Volume 1 = 0
is_exit_volume for Volume 1 = 0
LIST: mask_list for Volume 1 = []
LIST: masked_by_list for Volume 1 = []
LIST: masked_by_mask_index_list for Volume 1 = []
mask_mode for Volume 1 = 0
List overview for sample_container with cylinder shape made of Al
LIST: Children for Volume 2 = [1]
LIST: Direct_children for Volume 2 = [1]
LIST: Intersect_check_list for Volume 2 = [1]
LIST: Mask_intersect_list for Volume 2 = []
LIST: Destinations_list for Volume 2 = [3,5]
LIST: Reduced_destinations_list for Volume 2 = [5]
LIST: Next_volume_list for Volume 2 = [3,5,1]
Is_vacuum for Volume 2 = 0
is_mask_volume for Volume 2 = 0
is_masked_volume for Volume 2 = 0
is_exit_volume for Volume 2 = 0
LIST: mask_list for Volume 2 = []
LIST: masked_by_list for Volume 2 = []
LIST: masked_by_mask_index_list for Volume 2 = []
mask_mode for Volume 2 = 0
List overview for sample_container_lid with cylinder shape made of Al
LIST: Children for Volume 3 = []
LIST: Direct_children for Volume 3 = []
LIST: Intersect_check_list for Volume 3 = [2]
LIST: Mask_intersect_list for Volume 3 = []
LIST: Destinations_list for Volume 3 = [5]
LIST: Reduced_destinations_list for Volume 3 = [5]
LIST: Next_volume_list for Volume 3 = [5,2]
Is_vacuum for Volume 3 = 0
is_mask_volume for Volume 3 = 0
is_masked_volume for Volume 3 = 0
is_exit_volume for Volume 3 = 0
LIST: mask_list for Volume 3 = []
LIST: masked_by_list for Volume 3 = []
LIST: masked_by_mask_index_list for Volume 3 = []
mask_mode for Volume 3 = 0
List overview for cryostat_wall with cylinder shape made of Al
LIST: Children for Volume 4 = [1,2,3,5]
LIST: Direct_children for Volume 4 = [5]
LIST: Intersect_check_list for Volume 4 = [5]
LIST: Mask_intersect_list for Volume 4 = []
LIST: Destinations_list for Volume 4 = [0]
LIST: Reduced_destinations_list for Volume 4 = []
LIST: Next_volume_list for Volume 4 = [0,5]
Is_vacuum for Volume 4 = 0
is_mask_volume for Volume 4 = 0
is_masked_volume for Volume 4 = 0
is_exit_volume for Volume 4 = 0
LIST: mask_list for Volume 4 = []
LIST: masked_by_list for Volume 4 = []
LIST: masked_by_mask_index_list for Volume 4 = []
mask_mode for Volume 4 = 0
List overview for cryostat_wall_vacuum with cylinder shape made of Vacuum
LIST: Children for Volume 5 = [1,2,3]
LIST: Direct_children for Volume 5 = [2,3]
LIST: Intersect_check_list for Volume 5 = [2,3]
LIST: Mask_intersect_list for Volume 5 = []
LIST: Destinations_list for Volume 5 = [4]
LIST: Reduced_destinations_list for Volume 5 = [4]
LIST: Next_volume_list for Volume 5 = [4,2,3]
Is_vacuum for Volume 5 = 1
is_mask_volume for Volume 5 = 0
is_masked_volume for Volume 5 = 0
is_exit_volume for Volume 5 = 0
LIST: mask_list for Volume 5 = []
LIST: masked_by_list for Volume 5 = []
LIST: masked_by_mask_index_list for Volume 5 = []
mask_mode for Volume 5 = 0
Union_master component master initialized sucessfully
Detector: logger_space_zx_I=3321.57 logger_space_zx_ERR=8.1606 logger_space_zx_N=173579 "logger_zx.dat"
Detector: logger_space_zy_I=3321.57 logger_space_zy_ERR=8.1606 logger_space_zy_N=173579 "logger_zy.dat"
Detector: banana_I=319.678 banana_ERR=2.52868 banana_N=16779 "banana_1682428072.th"
INFO: Placing instr file copy python_tutorial.instr in dataset /Users/madsbertelsen/PaNOSC/McStasScript/github/McStasScript/docs/source/tutorial/data_folder/union_external_3
loading system configuration
ms.name_plot_options("logger_space_zx", data_empty, log=True, orders_of_mag=4)
ms.name_plot_options("logger_space_zy", data_empty, log=True, orders_of_mag=4)
ms.make_sub_plot(data_empty[0:2])
ms.make_sub_plot(data_empty[2])
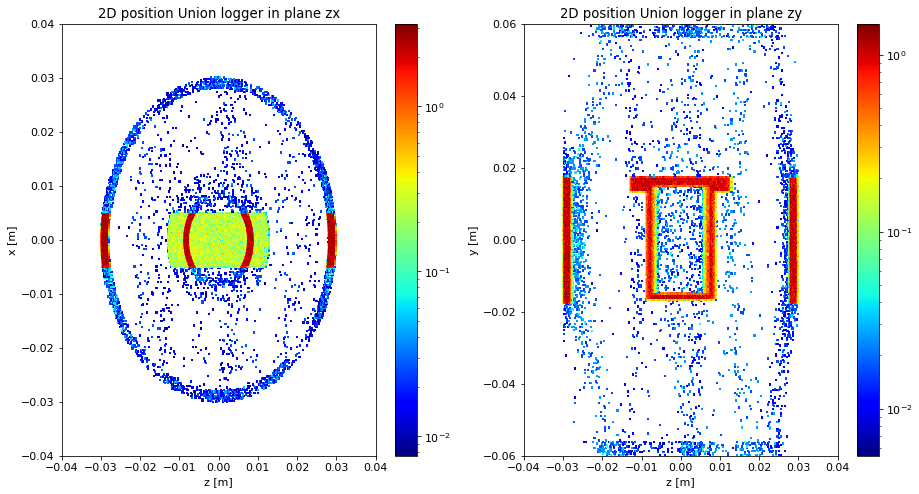
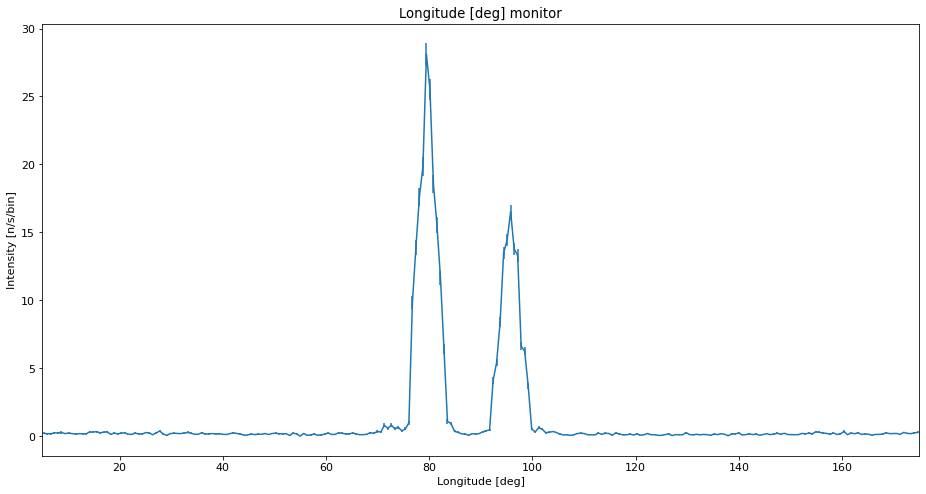
The loggers show our empty cryostat and sample container illuminated by a beam, and the banana monitor contains some powder scattering from the aluminium.
Adding an exit volume¶
Now we switch the sample_volume material from Vacuum to exit, ejecting rays from the simulation when they encounter it.
sample_volume.material_string='"Exit"'
instrument.show_diagram()
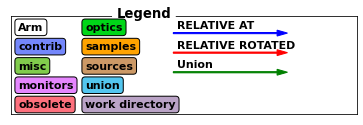
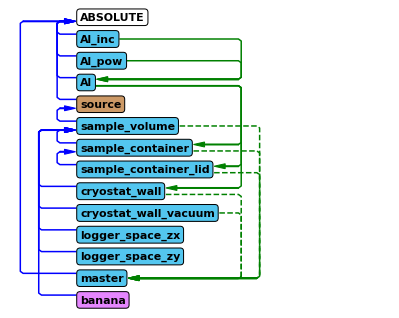
The diagram does not change between Vacuum and Exit being used for the sample_volume. Let us run the simulation and see the difference.
data = instrument.backengine()
INFO: Using directory: "/Users/madsbertelsen/PaNOSC/McStasScript/github/McStasScript/docs/source/tutorial/data_folder/union_external_4"
INFO: Regenerating c-file: python_tutorial.c
CFLAGS= -I@MCCODE_LIB@/share/ -I@MCCODE_LIB@/share/
INFO: Recompiling: ./python_tutorial.out
mccode-r.c:2837:3: warning: expression result unused [-Wunused-value]
*t0;
^~~
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1604:105: warning: incompatible function pointer types passing 'int (const struct saved_history_struct *, const struct saved_history_struct *)' to parameter of type 'int (* _Nonnull)(const void *, const void *)' [-Wincompatible-function-pointer-types]
qsort(total_history.saved_histories,total_history.used_elements,sizeof (struct saved_history_struct), Sample_compare_history_intensities);
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/stdlib.h:161:22: note: passing argument to parameter '__compar' here
int (* _Nonnull __compar)(const void *, const void *));
^
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1613:20: warning: incompatible pointer types passing 'struct saved_history_struct *' to parameter of type 'struct dynamic_history_list *' [-Wincompatible-pointer-types]
printf_history(&total_history.saved_histories[history_iterate]);
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1434:50: note: passing argument to parameter 'history' here
void printf_history(struct dynamic_history_list *history) {
^
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:2030:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:839:1: warning: non-void function does not return a value in all control paths [-Wreturn-type]
};
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:883:1: warning: non-void function does not return a value [-Wreturn-type]
};
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3274:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3274:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3276:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3276:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3278:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3278:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3280:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3280:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:14810:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zx_filename
^~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:14810:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zx_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:15053:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zy_filename
^~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:15053:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zy_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:788:15: warning: left operand of comma operator has no effect [-Wunused-value]
if (volume_index_main,Volumes[volume_index_main]->geometry.is_mask_volume == 0 ||
^~~~~~~~~~~~~~~~~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:788:90: warning: left operand of comma operator has no effect [-Wunused-value]
if (volume_index_main,Volumes[volume_index_main]->geometry.is_mask_volume == 0 ||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~^~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:789:92: warning: left operand of comma operator has no effect [-Wunused-value]
volume_index_main,Volumes[volume_index_main]->geometry.is_masked_volume == 0 ||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~^~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
14 warnings generated.
INFO: ===
Opening input file '/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//data/Al.laz' (Table_Read_Offset)
Table from file 'Al.laz' (block 1) is 26 x 18 (x=1:8), constant step. interpolation: linear
'# TITLE *Aluminum-Al-[FM3-M] Miller, H.P.jr.;DuMond, J.W.M.[1942] at 298 K; ...'
PowderN: Al_pow: Reading 26 rows from Al.laz
PowderN: Al_pow: Read 26 reflections from file 'Al.laz'
PowderN: Al_pow: Vc=66.4 [Angs] sigma_abs=0.924 [barn] sigma_inc=0.0328 [barn] reflections=Al.laz
---------------------------------------------------------------------
global_process_list.num_elements: 2
name of process [0]: Al_inc
component index [0]: 1
name of process [1]: Al_pow
component index [1]: 2
---------------------------------------------------------------------
global_material_list.num_elements: 1
name of material [0]: Al
component index [0]: 3
my_absoprtion [0]: 0.347892
number of processes [0]: 2
---------------------------------------------------------------------
global_geometry_list.num_elements: 1
name of geometry [0]: sample_volume
component index [0]: 5
Volume.name [0]: sample_volume
Volume.p_physics.is_vacuum [0]: 1
Volume.p_physics.my_absorption [0]: 0.000000
Volume.p_physics.number of processes [0]: 0
Volume.geometry.shape [0]: cylinder
Volume.geometry.center.x [0]: 0.000000
Volume.geometry.center.y [0]: 0.000000
Volume.geometry.center.z [0]: 1.000000
Volume.geometry.rotation_matrix[0] [0]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [0]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [0]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [0]: 0.007500
Volume.geometry.geometry_parameters.height [0]: 0.030000
Volume.geometry.focus_data_array.elements[0].Aim [0]: [0.000000 0.000000 1.000000]
name of geometry [1]: sample_container
component index [1]: 6
Volume.name [1]: sample_container
Volume.p_physics.is_vacuum [1]: 0
Volume.p_physics.my_absorption [1]: 0.347892
Volume.p_physics.number of processes [1]: 2
Volume.geometry.shape [1]: cylinder
Volume.geometry.center.x [1]: 0.000000
Volume.geometry.center.y [1]: 0.000000
Volume.geometry.center.z [1]: 1.000000
Volume.geometry.rotation_matrix[0] [1]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [1]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [1]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [1]: 0.009000
Volume.geometry.geometry_parameters.height [1]: 0.033000
Volume.geometry.focus_data_array.elements[0].Aim [1]: [0.000000 0.000000 1.000000]
name of geometry [2]: sample_container_lid
component index [2]: 7
Volume.name [2]: sample_container_lid
Volume.p_physics.is_vacuum [2]: 0
Volume.p_physics.my_absorption [2]: 0.347892
Volume.p_physics.number of processes [2]: 2
Volume.geometry.shape [2]: cylinder
Volume.geometry.center.x [2]: 0.000000
Volume.geometry.center.y [2]: 0.015500
Volume.geometry.center.z [2]: 1.000000
Volume.geometry.rotation_matrix[0] [2]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [2]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [2]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [2]: 0.013000
Volume.geometry.geometry_parameters.height [2]: 0.004000
Volume.geometry.focus_data_array.elements[0].Aim [2]: [0.000000 0.000000 1.000000]
name of geometry [3]: cryostat_wall
component index [3]: 8
Volume.name [3]: cryostat_wall
Volume.p_physics.is_vacuum [3]: 0
Volume.p_physics.my_absorption [3]: 0.347892
Volume.p_physics.number of processes [3]: 2
Volume.geometry.shape [3]: cylinder
Volume.geometry.center.x [3]: 0.000000
Volume.geometry.center.y [3]: 0.000000
Volume.geometry.center.z [3]: 1.000000
Volume.geometry.rotation_matrix[0] [3]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [3]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [3]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [3]: 0.030000
Volume.geometry.geometry_parameters.height [3]: 0.120000
Volume.geometry.focus_data_array.elements[0].Aim [3]: [0.000000 0.000000 1.000000]
name of geometry [4]: cryostat_wall_vacuum
component index [4]: 9
Volume.name [4]: cryostat_wall_vacuum
Volume.p_physics.is_vacuum [4]: 1
Volume.p_physics.my_absorption [4]: 0.000000
Volume.p_physics.number of processes [4]: 0
Volume.geometry.shape [4]: cylinder
Volume.geometry.center.x [4]: 0.000000
Volume.geometry.center.y [4]: 0.000000
Volume.geometry.center.z [4]: 1.000000
Volume.geometry.rotation_matrix[0] [4]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [4]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [4]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [4]: 0.028000
Volume.geometry.geometry_parameters.height [4]: 0.112000
Volume.geometry.focus_data_array.elements[0].Aim [4]: [0.000000 0.000000 1.000000]
---------------------------------------------------------------------
number_of_volumes = 6
number_of_masks = 0
number_of_masked_volumes = 0
---- Overview of the lists generated for each volume ----
List overview for surrounding vacuum
LIST: Children for Volume 0 = [1,2,3,4,5]
LIST: Direct_children for Volume 0 = [4]
LIST: Intersect_check_list for Volume 0 = [4]
LIST: Mask_intersect_list for Volume 0 = []
LIST: Destinations_list for Volume 0 = []
LIST: Reduced_destinations_list for Volume 0 = []
LIST: Next_volume_list for Volume 0 = [4]
LIST: mask_list for Volume 0 = []
LIST: masked_by_list for Volume 0 = []
LIST: masked_by_mask_index_list for Volume 0 = []
mask_mode for Volume 0 = 0
List overview for sample_volume with shape cylinder
LIST: Children for Volume 1 = []
LIST: Direct_children for Volume 1 = []
LIST: Intersect_check_list for Volume 1 = []
LIST: Mask_intersect_list for Volume 1 = []
LIST: Destinations_list for Volume 1 = [2,3]
LIST: Reduced_destinations_list for Volume 1 = [2,3]
LIST: Next_volume_list for Volume 1 = [2,3]
Is_vacuum for Volume 1 = 1
is_mask_volume for Volume 1 = 0
is_masked_volume for Volume 1 = 0
is_exit_volume for Volume 1 = 1
LIST: mask_list for Volume 1 = []
LIST: masked_by_list for Volume 1 = []
LIST: masked_by_mask_index_list for Volume 1 = []
mask_mode for Volume 1 = 0
List overview for sample_container with cylinder shape made of Al
LIST: Children for Volume 2 = [1]
LIST: Direct_children for Volume 2 = [1]
LIST: Intersect_check_list for Volume 2 = [1]
LIST: Mask_intersect_list for Volume 2 = []
LIST: Destinations_list for Volume 2 = [3,5]
LIST: Reduced_destinations_list for Volume 2 = [5]
LIST: Next_volume_list for Volume 2 = [3,5,1]
Is_vacuum for Volume 2 = 0
is_mask_volume for Volume 2 = 0
is_masked_volume for Volume 2 = 0
is_exit_volume for Volume 2 = 0
LIST: mask_list for Volume 2 = []
LIST: masked_by_list for Volume 2 = []
LIST: masked_by_mask_index_list for Volume 2 = []
mask_mode for Volume 2 = 0
List overview for sample_container_lid with cylinder shape made of Al
LIST: Children for Volume 3 = []
LIST: Direct_children for Volume 3 = []
LIST: Intersect_check_list for Volume 3 = [2]
LIST: Mask_intersect_list for Volume 3 = []
LIST: Destinations_list for Volume 3 = [5]
LIST: Reduced_destinations_list for Volume 3 = [5]
LIST: Next_volume_list for Volume 3 = [5,2]
Is_vacuum for Volume 3 = 0
is_mask_volume for Volume 3 = 0
is_masked_volume for Volume 3 = 0
is_exit_volume for Volume 3 = 0
LIST: mask_list for Volume 3 = []
LIST: masked_by_list for Volume 3 = []
LIST: masked_by_mask_index_list for Volume 3 = []
mask_mode for Volume 3 = 0
List overview for cryostat_wall with cylinder shape made of Al
LIST: Children for Volume 4 = [1,2,3,5]
LIST: Direct_children for Volume 4 = [5]
LIST: Intersect_check_list for Volume 4 = [5]
LIST: Mask_intersect_list for Volume 4 = []
LIST: Destinations_list for Volume 4 = [0]
LIST: Reduced_destinations_list for Volume 4 = []
LIST: Next_volume_list for Volume 4 = [0,5]
Is_vacuum for Volume 4 = 0
is_mask_volume for Volume 4 = 0
is_masked_volume for Volume 4 = 0
is_exit_volume for Volume 4 = 0
LIST: mask_list for Volume 4 = []
LIST: masked_by_list for Volume 4 = []
LIST: masked_by_mask_index_list for Volume 4 = []
mask_mode for Volume 4 = 0
List overview for cryostat_wall_vacuum with cylinder shape made of Vacuum
LIST: Children for Volume 5 = [1,2,3]
LIST: Direct_children for Volume 5 = [2,3]
LIST: Intersect_check_list for Volume 5 = [2,3]
LIST: Mask_intersect_list for Volume 5 = []
LIST: Destinations_list for Volume 5 = [4]
LIST: Reduced_destinations_list for Volume 5 = [4]
LIST: Next_volume_list for Volume 5 = [4,2,3]
Is_vacuum for Volume 5 = 1
is_mask_volume for Volume 5 = 0
is_masked_volume for Volume 5 = 0
is_exit_volume for Volume 5 = 0
LIST: mask_list for Volume 5 = []
LIST: masked_by_list for Volume 5 = []
LIST: masked_by_mask_index_list for Volume 5 = []
mask_mode for Volume 5 = 0
Union_master component master initialized sucessfully
Detector: logger_space_zx_I=2168.4 logger_space_zx_ERR=6.59484 logger_space_zx_N=113204 "logger_zx.dat"
Detector: logger_space_zy_I=2168.4 logger_space_zy_ERR=6.59484 logger_space_zy_N=113204 "logger_zy.dat"
Detector: banana_I=213.28 banana_ERR=2.05973 banana_N=11248 "banana_1682428083.th"
INFO: Placing instr file copy python_tutorial.instr in dataset /Users/madsbertelsen/PaNOSC/McStasScript/github/McStasScript/docs/source/tutorial/data_folder/union_external_4
loading system configuration
ms.name_plot_options("logger_space_zx", data, log=True, orders_of_mag=4)
ms.name_plot_options("logger_space_zy", data, log=True, orders_of_mag=4)
ms.make_sub_plot(data[0:2])
ms.make_sub_plot(data[2])
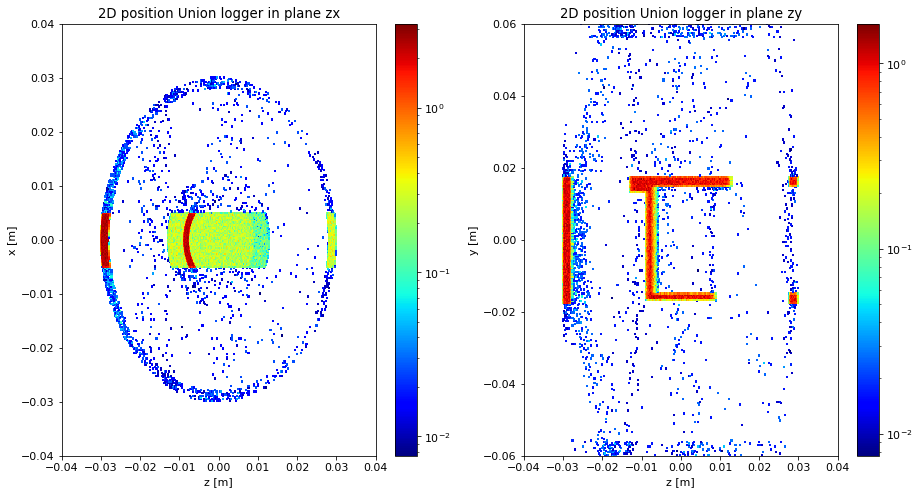
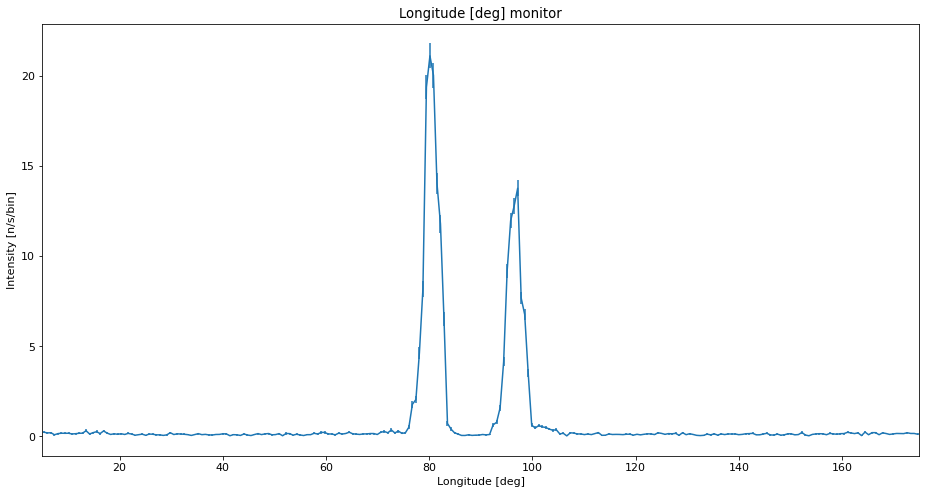
From the logger data one can see the back part of the sample container and exit of the cryostat is no longer illuminated, as the rays are removed from the Union simulation as soon as the touch the volume inside the sample container. The removed rays do not disappear completely, they are delivered to the next McStas component in the instrument.
Adding an external component in the gap¶
We can now see any part of the beam that intersected the exit volume is basically removed from the Union simulation. It is now however possible to insert another component within that exit volume, for example a sample not available as a Union process. Here we just use a PowderN sample in order to demonstrate. We select the same dimensions as the exit volume, but subtract 10 micrometer to avoid a perfect overlap.
sample = instrument.add_component("sample", "PowderN", after="master")
sample.set_AT([0,0,0], RELATIVE=sample_volume)
sample.radius = sample_volume.radius - 1E-5
sample.yheight = sample_volume.yheight - 2E-5
sample.reflections = '"Na2Ca3Al2F14.laz"'
instrument.show_diagram()
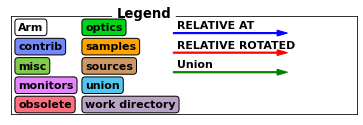
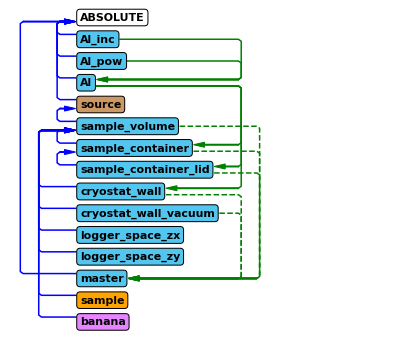
Running the simulation again¶
We run the simulation again, but know that the scattering within the sample wont be directly visible in the loggers.
data_wrong = instrument.backengine()
INFO: Using directory: "/Users/madsbertelsen/PaNOSC/McStasScript/github/McStasScript/docs/source/tutorial/data_folder/union_external_5"
INFO: Regenerating c-file: python_tutorial.c
CFLAGS= -I@MCCODE_LIB@/share/ -I@MCCODE_LIB@/share/
INFO: Recompiling: ./python_tutorial.out
mccode-r.c:2837:3: warning: expression result unused [-Wunused-value]
*t0;
^~~
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1604:105: warning: incompatible function pointer types passing 'int (const struct saved_history_struct *, const struct saved_history_struct *)' to parameter of type 'int (* _Nonnull)(const void *, const void *)' [-Wincompatible-function-pointer-types]
qsort(total_history.saved_histories,total_history.used_elements,sizeof (struct saved_history_struct), Sample_compare_history_intensities);
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/stdlib.h:161:22: note: passing argument to parameter '__compar' here
int (* _Nonnull __compar)(const void *, const void *));
^
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1613:20: warning: incompatible pointer types passing 'struct saved_history_struct *' to parameter of type 'struct dynamic_history_list *' [-Wincompatible-pointer-types]
printf_history(&total_history.saved_histories[history_iterate]);
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1434:50: note: passing argument to parameter 'history' here
void printf_history(struct dynamic_history_list *history) {
^
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:2030:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:839:1: warning: non-void function does not return a value in all control paths [-Wreturn-type]
};
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:883:1: warning: non-void function does not return a value [-Wreturn-type]
};
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3274:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3274:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3276:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3276:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3278:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3278:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3280:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3280:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:15340:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zx_filename
^~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:15340:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zx_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:15583:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zy_filename
^~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:15583:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zy_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:788:15: warning: left operand of comma operator has no effect [-Wunused-value]
if (volume_index_main,Volumes[volume_index_main]->geometry.is_mask_volume == 0 ||
^~~~~~~~~~~~~~~~~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:788:90: warning: left operand of comma operator has no effect [-Wunused-value]
if (volume_index_main,Volumes[volume_index_main]->geometry.is_mask_volume == 0 ||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~^~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:789:92: warning: left operand of comma operator has no effect [-Wunused-value]
volume_index_main,Volumes[volume_index_main]->geometry.is_masked_volume == 0 ||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~^~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
14 warnings generated.
INFO: ===
Opening input file '/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//data/Al.laz' (Table_Read_Offset)
Table from file 'Al.laz' (block 1) is 26 x 18 (x=1:8), constant step. interpolation: linear
'# TITLE *Aluminum-Al-[FM3-M] Miller, H.P.jr.;DuMond, J.W.M.[1942] at 298 K; ...'
PowderN: Al_pow: Reading 26 rows from Al.laz
PowderN: Al_pow: Read 26 reflections from file 'Al.laz'
PowderN: Al_pow: Vc=66.4 [Angs] sigma_abs=0.924 [barn] sigma_inc=0.0328 [barn] reflections=Al.laz
---------------------------------------------------------------------
global_process_list.num_elements: 2
name of process [0]: Al_inc
component index [0]: 1
name of process [1]: Al_pow
component index [1]: 2
---------------------------------------------------------------------
global_material_list.num_elements: 1
name of material [0]: Al
component index [0]: 3
my_absoprtion [0]: 0.347892
number of processes [0]: 2
---------------------------------------------------------------------
global_geometry_list.num_elements: 1
name of geometry [0]: sample_volume
component index [0]: 5
Volume.name [0]: sample_volume
Volume.p_physics.is_vacuum [0]: 1
Volume.p_physics.my_absorption [0]: 0.000000
Volume.p_physics.number of processes [0]: 0
Volume.geometry.shape [0]: cylinder
Volume.geometry.center.x [0]: 0.000000
Volume.geometry.center.y [0]: 0.000000
Volume.geometry.center.z [0]: 1.000000
Volume.geometry.rotation_matrix[0] [0]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [0]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [0]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [0]: 0.007500
Volume.geometry.geometry_parameters.height [0]: 0.030000
Volume.geometry.focus_data_array.elements[0].Aim [0]: [0.000000 0.000000 1.000000]
name of geometry [1]: sample_container
component index [1]: 6
Volume.name [1]: sample_container
Volume.p_physics.is_vacuum [1]: 0
Volume.p_physics.my_absorption [1]: 0.347892
Volume.p_physics.number of processes [1]: 2
Volume.geometry.shape [1]: cylinder
Volume.geometry.center.x [1]: 0.000000
Volume.geometry.center.y [1]: 0.000000
Volume.geometry.center.z [1]: 1.000000
Volume.geometry.rotation_matrix[0] [1]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [1]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [1]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [1]: 0.009000
Volume.geometry.geometry_parameters.height [1]: 0.033000
Volume.geometry.focus_data_array.elements[0].Aim [1]: [0.000000 0.000000 1.000000]
name of geometry [2]: sample_container_lid
component index [2]: 7
Volume.name [2]: sample_container_lid
Volume.p_physics.is_vacuum [2]: 0
Volume.p_physics.my_absorption [2]: 0.347892
Volume.p_physics.number of processes [2]: 2
Volume.geometry.shape [2]: cylinder
Volume.geometry.center.x [2]: 0.000000
Volume.geometry.center.y [2]: 0.015500
Volume.geometry.center.z [2]: 1.000000
Volume.geometry.rotation_matrix[0] [2]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [2]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [2]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [2]: 0.013000
Volume.geometry.geometry_parameters.height [2]: 0.004000
Volume.geometry.focus_data_array.elements[0].Aim [2]: [0.000000 0.000000 1.000000]
name of geometry [3]: cryostat_wall
component index [3]: 8
Volume.name [3]: cryostat_wall
Volume.p_physics.is_vacuum [3]: 0
Volume.p_physics.my_absorption [3]: 0.347892
Volume.p_physics.number of processes [3]: 2
Volume.geometry.shape [3]: cylinder
Volume.geometry.center.x [3]: 0.000000
Volume.geometry.center.y [3]: 0.000000
Volume.geometry.center.z [3]: 1.000000
Volume.geometry.rotation_matrix[0] [3]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [3]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [3]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [3]: 0.030000
Volume.geometry.geometry_parameters.height [3]: 0.120000
Volume.geometry.focus_data_array.elements[0].Aim [3]: [0.000000 0.000000 1.000000]
name of geometry [4]: cryostat_wall_vacuum
component index [4]: 9
Volume.name [4]: cryostat_wall_vacuum
Volume.p_physics.is_vacuum [4]: 1
Volume.p_physics.my_absorption [4]: 0.000000
Volume.p_physics.number of processes [4]: 0
Volume.geometry.shape [4]: cylinder
Volume.geometry.center.x [4]: 0.000000
Volume.geometry.center.y [4]: 0.000000
Volume.geometry.center.z [4]: 1.000000
Volume.geometry.rotation_matrix[0] [4]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [4]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [4]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [4]: 0.028000
Volume.geometry.geometry_parameters.height [4]: 0.112000
Volume.geometry.focus_data_array.elements[0].Aim [4]: [0.000000 0.000000 1.000000]
---------------------------------------------------------------------
number_of_volumes = 6
number_of_masks = 0
number_of_masked_volumes = 0
---- Overview of the lists generated for each volume ----
List overview for surrounding vacuum
LIST: Children for Volume 0 = [1,2,3,4,5]
LIST: Direct_children for Volume 0 = [4]
LIST: Intersect_check_list for Volume 0 = [4]
LIST: Mask_intersect_list for Volume 0 = []
LIST: Destinations_list for Volume 0 = []
LIST: Reduced_destinations_list for Volume 0 = []
LIST: Next_volume_list for Volume 0 = [4]
LIST: mask_list for Volume 0 = []
LIST: masked_by_list for Volume 0 = []
LIST: masked_by_mask_index_list for Volume 0 = []
mask_mode for Volume 0 = 0
List overview for sample_volume with shape cylinder
LIST: Children for Volume 1 = []
LIST: Direct_children for Volume 1 = []
LIST: Intersect_check_list for Volume 1 = []
LIST: Mask_intersect_list for Volume 1 = []
LIST: Destinations_list for Volume 1 = [2,3]
LIST: Reduced_destinations_list for Volume 1 = [2,3]
LIST: Next_volume_list for Volume 1 = [2,3]
Is_vacuum for Volume 1 = 1
is_mask_volume for Volume 1 = 0
is_masked_volume for Volume 1 = 0
is_exit_volume for Volume 1 = 1
LIST: mask_list for Volume 1 = []
LIST: masked_by_list for Volume 1 = []
LIST: masked_by_mask_index_list for Volume 1 = []
mask_mode for Volume 1 = 0
List overview for sample_container with cylinder shape made of Al
LIST: Children for Volume 2 = [1]
LIST: Direct_children for Volume 2 = [1]
LIST: Intersect_check_list for Volume 2 = [1]
LIST: Mask_intersect_list for Volume 2 = []
LIST: Destinations_list for Volume 2 = [3,5]
LIST: Reduced_destinations_list for Volume 2 = [5]
LIST: Next_volume_list for Volume 2 = [3,5,1]
Is_vacuum for Volume 2 = 0
is_mask_volume for Volume 2 = 0
is_masked_volume for Volume 2 = 0
is_exit_volume for Volume 2 = 0
LIST: mask_list for Volume 2 = []
LIST: masked_by_list for Volume 2 = []
LIST: masked_by_mask_index_list for Volume 2 = []
mask_mode for Volume 2 = 0
List overview for sample_container_lid with cylinder shape made of Al
LIST: Children for Volume 3 = []
LIST: Direct_children for Volume 3 = []
LIST: Intersect_check_list for Volume 3 = [2]
LIST: Mask_intersect_list for Volume 3 = []
LIST: Destinations_list for Volume 3 = [5]
LIST: Reduced_destinations_list for Volume 3 = [5]
LIST: Next_volume_list for Volume 3 = [5,2]
Is_vacuum for Volume 3 = 0
is_mask_volume for Volume 3 = 0
is_masked_volume for Volume 3 = 0
is_exit_volume for Volume 3 = 0
LIST: mask_list for Volume 3 = []
LIST: masked_by_list for Volume 3 = []
LIST: masked_by_mask_index_list for Volume 3 = []
mask_mode for Volume 3 = 0
List overview for cryostat_wall with cylinder shape made of Al
LIST: Children for Volume 4 = [1,2,3,5]
LIST: Direct_children for Volume 4 = [5]
LIST: Intersect_check_list for Volume 4 = [5]
LIST: Mask_intersect_list for Volume 4 = []
LIST: Destinations_list for Volume 4 = [0]
LIST: Reduced_destinations_list for Volume 4 = []
LIST: Next_volume_list for Volume 4 = [0,5]
Is_vacuum for Volume 4 = 0
is_mask_volume for Volume 4 = 0
is_masked_volume for Volume 4 = 0
is_exit_volume for Volume 4 = 0
LIST: mask_list for Volume 4 = []
LIST: masked_by_list for Volume 4 = []
LIST: masked_by_mask_index_list for Volume 4 = []
mask_mode for Volume 4 = 0
List overview for cryostat_wall_vacuum with cylinder shape made of Vacuum
LIST: Children for Volume 5 = [1,2,3]
LIST: Direct_children for Volume 5 = [2,3]
LIST: Intersect_check_list for Volume 5 = [2,3]
LIST: Mask_intersect_list for Volume 5 = []
LIST: Destinations_list for Volume 5 = [4]
LIST: Reduced_destinations_list for Volume 5 = [4]
LIST: Next_volume_list for Volume 5 = [4,2,3]
Is_vacuum for Volume 5 = 1
is_mask_volume for Volume 5 = 0
is_masked_volume for Volume 5 = 0
is_exit_volume for Volume 5 = 0
LIST: mask_list for Volume 5 = []
LIST: masked_by_list for Volume 5 = []
LIST: masked_by_mask_index_list for Volume 5 = []
mask_mode for Volume 5 = 0
Union_master component master initialized sucessfully
Opening input file '/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//data/Na2Ca3Al2F14.laz' (Table_Read_Offset)
Table from file 'Na2Ca3Al2F14.laz' (block 1) is 841 x 18 (x=1:20), constant step. interpolation: linear
'# TITLE *-Na2Ca3Al2F14-[I213] Courbion, G.;Ferey, G.[1988] Standard NAC cal ...'
PowderN: sample: Reading 841 rows from Na2Ca3Al2F14.laz
PowderN: sample: Read 841 reflections from file 'Na2Ca3Al2F14.laz'
PowderN: sample: Vc=1079.1 [Angs] sigma_abs=11.7856 [barn] sigma_inc=13.6704 [barn] reflections=Na2Ca3Al2F14.laz
Detector: logger_space_zx_I=2184.21 logger_space_zx_ERR=6.61945 logger_space_zx_N=114014 "logger_zx.dat"
Detector: logger_space_zy_I=2184.21 logger_space_zy_ERR=6.61945 logger_space_zy_N=114014 "logger_zy.dat"
Detector: banana_I=1698.02 banana_ERR=5.10672 banana_N=322775 "banana_1682428092.th"
PowderN: sample: Info: you may highly improve the computation efficiency by using
SPLIT 37 COMPONENT sample=PowderN(...)
in the instrument description python_tutorial.instr.
INFO: Placing instr file copy python_tutorial.instr in dataset /Users/madsbertelsen/PaNOSC/McStasScript/github/McStasScript/docs/source/tutorial/data_folder/union_external_5
loading system configuration
ms.name_plot_options("logger_space_zx", data_wrong, log=True, orders_of_mag=4)
ms.name_plot_options("logger_space_zy", data_wrong, log=True, orders_of_mag=4)
ms.make_sub_plot(data_wrong[0:2])
ms.make_sub_plot(data_wrong[2])
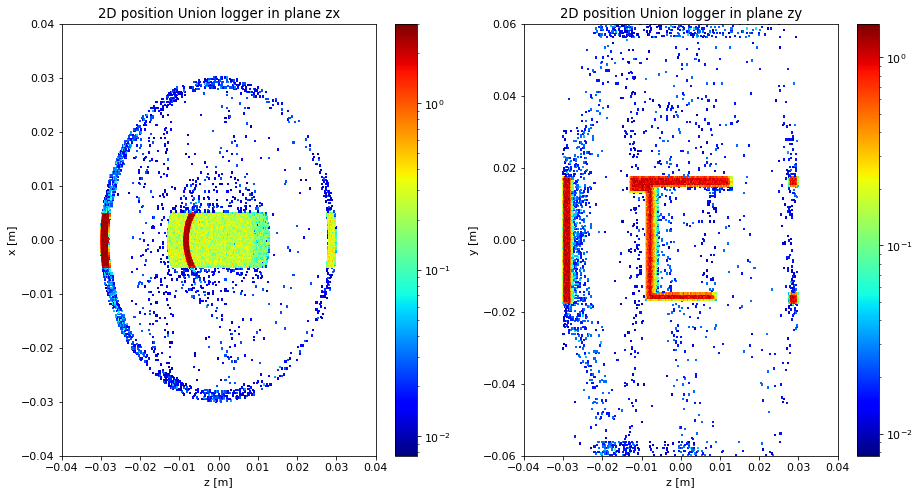
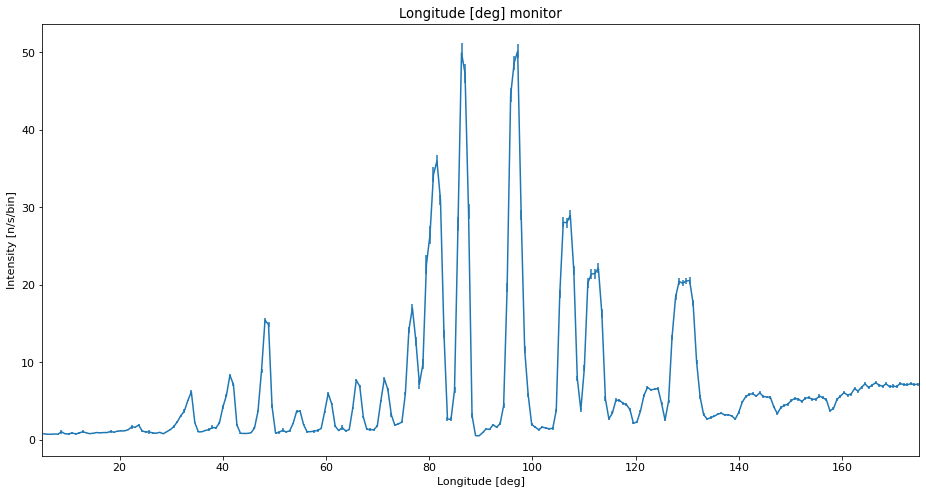
Interpretation of the data¶
Now we have added a sample inside the Union geometry, but when the neutron reaches that sample, it is ignored by the sample environment leading to unphysical behavior. Here the beam does not illuminate the sample environment on the way out, and all rays scattered by the PowderN sample are not attenuated by the walls of the cryostat when leaving.
Allowing the rays to return to the Union_master¶
Now we could recreate the entire sample environment with new geometries and insert an additional unit master to grab the neutrons after the external sample, yet this would be error prone as all geometries would need to be exactly the same. Instead it is possible to tell Union geometries that they should be simulated in several of the next Union_master components using the number_of_activation parameter on each Union geometry, which is 1 per default.
Setting it to 2, we tell the geometries that they should be simulated in the two next Union_master components. We do not update the sample_volume which is an exit volume, as this would allow the ray to escape once more. Instead we will replace it with Vacuum, but one could also have placed something closer to the actual sample.
One last necessary detail is to set the allow_inside_start parameter on the second Union_master component. This disables an error message that would occur if a neutron starts inside a Union geometry, as this is most likely an error. Here we want to do this on purpose, and we need to let the Union_master component know this is allowed.
container.number_of_activations = 2
container_lid.number_of_activations = 2
inner_wall.number_of_activations = 2
inner_wall_vac.number_of_activations = 2
sample_replacement = instrument.add_component("sample_volume_replace", "Union_cylinder", after=sample)
sample_replacement.yheight = sample_volume.yheight
sample_replacement.radius = sample_volume.radius
sample_replacement.material_string='"Vacuum"'
sample_replacement.priority = 101
sample_replacement.set_AT([0,0,0], RELATIVE=sample_volume)
master_2 = instrument.add_component("master_after_sample", "Union_master", after=sample_replacement)
master_2.allow_inside_start=1
instrument.show_diagram()
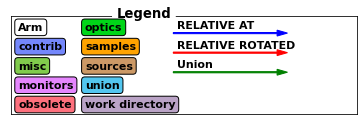
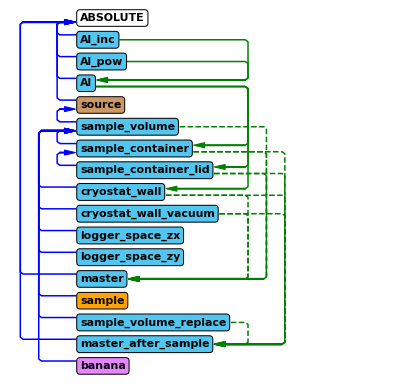
Notice that sample_volume is only simulated in the first master, there is no arrow to the second master as with all the other geometries.
data = instrument.backengine()
INFO: Using directory: "/Users/madsbertelsen/PaNOSC/McStasScript/github/McStasScript/docs/source/tutorial/data_folder/union_external_6"
INFO: Regenerating c-file: python_tutorial.c
CFLAGS= -I@MCCODE_LIB@/share/ -I@MCCODE_LIB@/share/
INFO: Recompiling: ./python_tutorial.out
mccode-r.c:2837:3: warning: expression result unused [-Wunused-value]
*t0;
^~~
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1604:105: warning: incompatible function pointer types passing 'int (const struct saved_history_struct *, const struct saved_history_struct *)' to parameter of type 'int (* _Nonnull)(const void *, const void *)' [-Wincompatible-function-pointer-types]
qsort(total_history.saved_histories,total_history.used_elements,sizeof (struct saved_history_struct), Sample_compare_history_intensities);
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/stdlib.h:161:22: note: passing argument to parameter '__compar' here
int (* _Nonnull __compar)(const void *, const void *));
^
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1613:20: warning: incompatible pointer types passing 'struct saved_history_struct *' to parameter of type 'struct dynamic_history_list *' [-Wincompatible-pointer-types]
printf_history(&total_history.saved_histories[history_iterate]);
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1434:50: note: passing argument to parameter 'history' here
void printf_history(struct dynamic_history_list *history) {
^
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:2030:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:839:1: warning: non-void function does not return a value in all control paths [-Wreturn-type]
};
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:883:1: warning: non-void function does not return a value [-Wreturn-type]
};
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3274:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3274:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3276:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3276:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3278:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3278:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3280:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3280:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:15972:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zx_filename
^~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:15972:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zx_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:16215:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zy_filename
^~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:16215:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zy_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:788:15: warning: left operand of comma operator has no effect [-Wunused-value]
if (volume_index_main,Volumes[volume_index_main]->geometry.is_mask_volume == 0 ||
^~~~~~~~~~~~~~~~~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:788:90: warning: left operand of comma operator has no effect [-Wunused-value]
if (volume_index_main,Volumes[volume_index_main]->geometry.is_mask_volume == 0 ||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~^~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:789:92: warning: left operand of comma operator has no effect [-Wunused-value]
volume_index_main,Volumes[volume_index_main]->geometry.is_masked_volume == 0 ||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~^~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:788:15: warning: left operand of comma operator has no effect [-Wunused-value]
if (volume_index_main,Volumes[volume_index_main]->geometry.is_mask_volume == 0 ||
^~~~~~~~~~~~~~~~~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:788:90: warning: left operand of comma operator has no effect [-Wunused-value]
if (volume_index_main,Volumes[volume_index_main]->geometry.is_mask_volume == 0 ||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~^~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:789:92: warning: left operand of comma operator has no effect [-Wunused-value]
volume_index_main,Volumes[volume_index_main]->geometry.is_masked_volume == 0 ||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~^~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
17 warnings generated.
INFO: ===
Opening input file '/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//data/Al.laz' (Table_Read_Offset)
Table from file 'Al.laz' (block 1) is 26 x 18 (x=1:8), constant step. interpolation: linear
'# TITLE *Aluminum-Al-[FM3-M] Miller, H.P.jr.;DuMond, J.W.M.[1942] at 298 K; ...'
PowderN: Al_pow: Reading 26 rows from Al.laz
PowderN: Al_pow: Read 26 reflections from file 'Al.laz'
PowderN: Al_pow: Vc=66.4 [Angs] sigma_abs=0.924 [barn] sigma_inc=0.0328 [barn] reflections=Al.laz
---------------------------------------------------------------------
global_process_list.num_elements: 2
name of process [0]: Al_inc
component index [0]: 1
name of process [1]: Al_pow
component index [1]: 2
---------------------------------------------------------------------
global_material_list.num_elements: 1
name of material [0]: Al
component index [0]: 3
my_absoprtion [0]: 0.347892
number of processes [0]: 2
---------------------------------------------------------------------
global_geometry_list.num_elements: 1
name of geometry [0]: sample_volume
component index [0]: 5
Volume.name [0]: sample_volume
Volume.p_physics.is_vacuum [0]: 1
Volume.p_physics.my_absorption [0]: 0.000000
Volume.p_physics.number of processes [0]: 0
Volume.geometry.shape [0]: cylinder
Volume.geometry.center.x [0]: 0.000000
Volume.geometry.center.y [0]: 0.000000
Volume.geometry.center.z [0]: 1.000000
Volume.geometry.rotation_matrix[0] [0]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [0]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [0]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [0]: 0.007500
Volume.geometry.geometry_parameters.height [0]: 0.030000
Volume.geometry.focus_data_array.elements[0].Aim [0]: [0.000000 0.000000 1.000000]
name of geometry [1]: sample_container
component index [1]: 6
Volume.name [1]: sample_container
Volume.p_physics.is_vacuum [1]: 0
Volume.p_physics.my_absorption [1]: 0.347892
Volume.p_physics.number of processes [1]: 2
Volume.geometry.shape [1]: cylinder
Volume.geometry.center.x [1]: 0.000000
Volume.geometry.center.y [1]: 0.000000
Volume.geometry.center.z [1]: 1.000000
Volume.geometry.rotation_matrix[0] [1]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [1]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [1]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [1]: 0.009000
Volume.geometry.geometry_parameters.height [1]: 0.033000
Volume.geometry.focus_data_array.elements[0].Aim [1]: [0.000000 0.000000 1.000000]
name of geometry [2]: sample_container_lid
component index [2]: 7
Volume.name [2]: sample_container_lid
Volume.p_physics.is_vacuum [2]: 0
Volume.p_physics.my_absorption [2]: 0.347892
Volume.p_physics.number of processes [2]: 2
Volume.geometry.shape [2]: cylinder
Volume.geometry.center.x [2]: 0.000000
Volume.geometry.center.y [2]: 0.015500
Volume.geometry.center.z [2]: 1.000000
Volume.geometry.rotation_matrix[0] [2]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [2]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [2]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [2]: 0.013000
Volume.geometry.geometry_parameters.height [2]: 0.004000
Volume.geometry.focus_data_array.elements[0].Aim [2]: [0.000000 0.000000 1.000000]
name of geometry [3]: cryostat_wall
component index [3]: 8
Volume.name [3]: cryostat_wall
Volume.p_physics.is_vacuum [3]: 0
Volume.p_physics.my_absorption [3]: 0.347892
Volume.p_physics.number of processes [3]: 2
Volume.geometry.shape [3]: cylinder
Volume.geometry.center.x [3]: 0.000000
Volume.geometry.center.y [3]: 0.000000
Volume.geometry.center.z [3]: 1.000000
Volume.geometry.rotation_matrix[0] [3]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [3]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [3]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [3]: 0.030000
Volume.geometry.geometry_parameters.height [3]: 0.120000
Volume.geometry.focus_data_array.elements[0].Aim [3]: [0.000000 0.000000 1.000000]
name of geometry [4]: cryostat_wall_vacuum
component index [4]: 9
Volume.name [4]: cryostat_wall_vacuum
Volume.p_physics.is_vacuum [4]: 1
Volume.p_physics.my_absorption [4]: 0.000000
Volume.p_physics.number of processes [4]: 0
Volume.geometry.shape [4]: cylinder
Volume.geometry.center.x [4]: 0.000000
Volume.geometry.center.y [4]: 0.000000
Volume.geometry.center.z [4]: 1.000000
Volume.geometry.rotation_matrix[0] [4]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [4]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [4]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [4]: 0.028000
Volume.geometry.geometry_parameters.height [4]: 0.112000
Volume.geometry.focus_data_array.elements[0].Aim [4]: [0.000000 0.000000 1.000000]
---------------------------------------------------------------------
number_of_volumes = 6
number_of_masks = 0
number_of_masked_volumes = 0
---- Overview of the lists generated for each volume ----
List overview for surrounding vacuum
LIST: Children for Volume 0 = [1,2,3,4,5]
LIST: Direct_children for Volume 0 = [4]
LIST: Intersect_check_list for Volume 0 = [4]
LIST: Mask_intersect_list for Volume 0 = []
LIST: Destinations_list for Volume 0 = []
LIST: Reduced_destinations_list for Volume 0 = []
LIST: Next_volume_list for Volume 0 = [4]
LIST: mask_list for Volume 0 = []
LIST: masked_by_list for Volume 0 = []
LIST: masked_by_mask_index_list for Volume 0 = []
mask_mode for Volume 0 = 0
List overview for sample_volume with shape cylinder
LIST: Children for Volume 1 = []
LIST: Direct_children for Volume 1 = []
LIST: Intersect_check_list for Volume 1 = []
LIST: Mask_intersect_list for Volume 1 = []
LIST: Destinations_list for Volume 1 = [2,3]
LIST: Reduced_destinations_list for Volume 1 = [2,3]
LIST: Next_volume_list for Volume 1 = [2,3]
Is_vacuum for Volume 1 = 1
is_mask_volume for Volume 1 = 0
is_masked_volume for Volume 1 = 0
is_exit_volume for Volume 1 = 1
LIST: mask_list for Volume 1 = []
LIST: masked_by_list for Volume 1 = []
LIST: masked_by_mask_index_list for Volume 1 = []
mask_mode for Volume 1 = 0
List overview for sample_container with cylinder shape made of Al
LIST: Children for Volume 2 = [1]
LIST: Direct_children for Volume 2 = [1]
LIST: Intersect_check_list for Volume 2 = [1]
LIST: Mask_intersect_list for Volume 2 = []
LIST: Destinations_list for Volume 2 = [3,5]
LIST: Reduced_destinations_list for Volume 2 = [5]
LIST: Next_volume_list for Volume 2 = [3,5,1]
Is_vacuum for Volume 2 = 0
is_mask_volume for Volume 2 = 0
is_masked_volume for Volume 2 = 0
is_exit_volume for Volume 2 = 0
LIST: mask_list for Volume 2 = []
LIST: masked_by_list for Volume 2 = []
LIST: masked_by_mask_index_list for Volume 2 = []
mask_mode for Volume 2 = 0
List overview for sample_container_lid with cylinder shape made of Al
LIST: Children for Volume 3 = []
LIST: Direct_children for Volume 3 = []
LIST: Intersect_check_list for Volume 3 = [2]
LIST: Mask_intersect_list for Volume 3 = []
LIST: Destinations_list for Volume 3 = [5]
LIST: Reduced_destinations_list for Volume 3 = [5]
LIST: Next_volume_list for Volume 3 = [5,2]
Is_vacuum for Volume 3 = 0
is_mask_volume for Volume 3 = 0
is_masked_volume for Volume 3 = 0
is_exit_volume for Volume 3 = 0
LIST: mask_list for Volume 3 = []
LIST: masked_by_list for Volume 3 = []
LIST: masked_by_mask_index_list for Volume 3 = []
mask_mode for Volume 3 = 0
List overview for cryostat_wall with cylinder shape made of Al
LIST: Children for Volume 4 = [1,2,3,5]
LIST: Direct_children for Volume 4 = [5]
LIST: Intersect_check_list for Volume 4 = [5]
LIST: Mask_intersect_list for Volume 4 = []
LIST: Destinations_list for Volume 4 = [0]
LIST: Reduced_destinations_list for Volume 4 = []
LIST: Next_volume_list for Volume 4 = [0,5]
Is_vacuum for Volume 4 = 0
is_mask_volume for Volume 4 = 0
is_masked_volume for Volume 4 = 0
is_exit_volume for Volume 4 = 0
LIST: mask_list for Volume 4 = []
LIST: masked_by_list for Volume 4 = []
LIST: masked_by_mask_index_list for Volume 4 = []
mask_mode for Volume 4 = 0
List overview for cryostat_wall_vacuum with cylinder shape made of Vacuum
LIST: Children for Volume 5 = [1,2,3]
LIST: Direct_children for Volume 5 = [2,3]
LIST: Intersect_check_list for Volume 5 = [2,3]
LIST: Mask_intersect_list for Volume 5 = []
LIST: Destinations_list for Volume 5 = [4]
LIST: Reduced_destinations_list for Volume 5 = [4]
LIST: Next_volume_list for Volume 5 = [4,2,3]
Is_vacuum for Volume 5 = 1
is_mask_volume for Volume 5 = 0
is_masked_volume for Volume 5 = 0
is_exit_volume for Volume 5 = 0
LIST: mask_list for Volume 5 = []
LIST: masked_by_list for Volume 5 = []
LIST: masked_by_mask_index_list for Volume 5 = []
mask_mode for Volume 5 = 0
Union_master component master initialized sucessfully
Opening input file '/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//data/Na2Ca3Al2F14.laz' (Table_Read_Offset)
Table from file 'Na2Ca3Al2F14.laz' (block 1) is 841 x 18 (x=1:20), constant step. interpolation: linear
'# TITLE *-Na2Ca3Al2F14-[I213] Courbion, G.;Ferey, G.[1988] Standard NAC cal ...'
PowderN: sample: Reading 841 rows from Na2Ca3Al2F14.laz
PowderN: sample: Read 841 reflections from file 'Na2Ca3Al2F14.laz'
PowderN: sample: Vc=1079.1 [Angs] sigma_abs=11.7856 [barn] sigma_inc=13.6704 [barn] reflections=Na2Ca3Al2F14.laz
---------------------------------------------------------------------
global_process_list.num_elements: 2
name of process [0]: Al_inc
component index [0]: 1
name of process [1]: Al_pow
component index [1]: 2
---------------------------------------------------------------------
global_material_list.num_elements: 1
name of material [0]: Al
component index [0]: 3
my_absoprtion [0]: 0.347892
number of processes [0]: 2
---------------------------------------------------------------------
global_geometry_list.num_elements: 1
name of geometry [1]: sample_container
component index [1]: 6
Volume.name [1]: sample_container
Volume.p_physics.is_vacuum [1]: 0
Volume.p_physics.my_absorption [1]: 0.347892
Volume.p_physics.number of processes [1]: 2
Volume.geometry.shape [1]: cylinder
Volume.geometry.center.x [1]: 0.000000
Volume.geometry.center.y [1]: 0.000000
Volume.geometry.center.z [1]: 1.000000
Volume.geometry.rotation_matrix[0] [1]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [1]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [1]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [1]: 0.009000
Volume.geometry.geometry_parameters.height [1]: 0.033000
Volume.geometry.focus_data_array.elements[0].Aim [1]: [0.000000 0.000000 1.000000]
name of geometry [2]: sample_container_lid
component index [2]: 7
Volume.name [2]: sample_container_lid
Volume.p_physics.is_vacuum [2]: 0
Volume.p_physics.my_absorption [2]: 0.347892
Volume.p_physics.number of processes [2]: 2
Volume.geometry.shape [2]: cylinder
Volume.geometry.center.x [2]: 0.000000
Volume.geometry.center.y [2]: 0.015500
Volume.geometry.center.z [2]: 1.000000
Volume.geometry.rotation_matrix[0] [2]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [2]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [2]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [2]: 0.013000
Volume.geometry.geometry_parameters.height [2]: 0.004000
Volume.geometry.focus_data_array.elements[0].Aim [2]: [0.000000 0.000000 1.000000]
name of geometry [3]: cryostat_wall
component index [3]: 8
Volume.name [3]: cryostat_wall
Volume.p_physics.is_vacuum [3]: 0
Volume.p_physics.my_absorption [3]: 0.347892
Volume.p_physics.number of processes [3]: 2
Volume.geometry.shape [3]: cylinder
Volume.geometry.center.x [3]: 0.000000
Volume.geometry.center.y [3]: 0.000000
Volume.geometry.center.z [3]: 1.000000
Volume.geometry.rotation_matrix[0] [3]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [3]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [3]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [3]: 0.030000
Volume.geometry.geometry_parameters.height [3]: 0.120000
Volume.geometry.focus_data_array.elements[0].Aim [3]: [0.000000 0.000000 1.000000]
name of geometry [4]: cryostat_wall_vacuum
component index [4]: 9
Volume.name [4]: cryostat_wall_vacuum
Volume.p_physics.is_vacuum [4]: 1
Volume.p_physics.my_absorption [4]: 0.000000
Volume.p_physics.number of processes [4]: 0
Volume.geometry.shape [4]: cylinder
Volume.geometry.center.x [4]: 0.000000
Volume.geometry.center.y [4]: 0.000000
Volume.geometry.center.z [4]: 1.000000
Volume.geometry.rotation_matrix[0] [4]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [4]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [4]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [4]: 0.028000
Volume.geometry.geometry_parameters.height [4]: 0.112000
Volume.geometry.focus_data_array.elements[0].Aim [4]: [0.000000 0.000000 1.000000]
name of geometry [5]: sample_volume_replace
component index [5]: 14
Volume.name [5]: sample_volume_replace
Volume.p_physics.is_vacuum [5]: 1
Volume.p_physics.my_absorption [5]: 0.000000
Volume.p_physics.number of processes [5]: 0
Volume.geometry.shape [5]: cylinder
Volume.geometry.center.x [5]: 0.000000
Volume.geometry.center.y [5]: 0.000000
Volume.geometry.center.z [5]: 1.000000
Volume.geometry.rotation_matrix[0] [5]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [5]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [5]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [5]: 0.007500
Volume.geometry.geometry_parameters.height [5]: 0.030000
Volume.geometry.focus_data_array.elements[0].Aim [5]: [0.000000 0.000000 1.000000]
---------------------------------------------------------------------
number_of_volumes = 6
number_of_masks = 0
number_of_masked_volumes = 0
---- Overview of the lists generated for each volume ----
List overview for surrounding vacuum
LIST: Children for Volume 0 = [1,2,3,4,5]
LIST: Direct_children for Volume 0 = [3]
LIST: Intersect_check_list for Volume 0 = [3]
LIST: Mask_intersect_list for Volume 0 = []
LIST: Destinations_list for Volume 0 = []
LIST: Reduced_destinations_list for Volume 0 = []
LIST: Next_volume_list for Volume 0 = [3]
LIST: mask_list for Volume 0 = []
LIST: masked_by_list for Volume 0 = []
LIST: masked_by_mask_index_list for Volume 0 = []
mask_mode for Volume 0 = 0
List overview for sample_container with cylinder shape made of Al
LIST: Children for Volume 1 = [5]
LIST: Direct_children for Volume 1 = [5]
LIST: Intersect_check_list for Volume 1 = [5]
LIST: Mask_intersect_list for Volume 1 = []
LIST: Destinations_list for Volume 1 = [2,4]
LIST: Reduced_destinations_list for Volume 1 = [4]
LIST: Next_volume_list for Volume 1 = [2,4,5]
Is_vacuum for Volume 1 = 0
is_mask_volume for Volume 1 = 0
is_masked_volume for Volume 1 = 0
is_exit_volume for Volume 1 = 0
LIST: mask_list for Volume 1 = []
LIST: masked_by_list for Volume 1 = []
LIST: masked_by_mask_index_list for Volume 1 = []
mask_mode for Volume 1 = 0
List overview for sample_container_lid with cylinder shape made of Al
LIST: Children for Volume 2 = []
LIST: Direct_children for Volume 2 = []
LIST: Intersect_check_list for Volume 2 = [1]
LIST: Mask_intersect_list for Volume 2 = []
LIST: Destinations_list for Volume 2 = [4]
LIST: Reduced_destinations_list for Volume 2 = [4]
LIST: Next_volume_list for Volume 2 = [4,1]
Is_vacuum for Volume 2 = 0
is_mask_volume for Volume 2 = 0
is_masked_volume for Volume 2 = 0
is_exit_volume for Volume 2 = 0
LIST: mask_list for Volume 2 = []
LIST: masked_by_list for Volume 2 = []
LIST: masked_by_mask_index_list for Volume 2 = []
mask_mode for Volume 2 = 0
List overview for cryostat_wall with cylinder shape made of Al
LIST: Children for Volume 3 = [1,2,4,5]
LIST: Direct_children for Volume 3 = [4]
LIST: Intersect_check_list for Volume 3 = [4]
LIST: Mask_intersect_list for Volume 3 = []
LIST: Destinations_list for Volume 3 = [0]
LIST: Reduced_destinations_list for Volume 3 = []
LIST: Next_volume_list for Volume 3 = [0,4]
Is_vacuum for Volume 3 = 0
is_mask_volume for Volume 3 = 0
is_masked_volume for Volume 3 = 0
is_exit_volume for Volume 3 = 0
LIST: mask_list for Volume 3 = []
LIST: masked_by_list for Volume 3 = []
LIST: masked_by_mask_index_list for Volume 3 = []
mask_mode for Volume 3 = 0
List overview for cryostat_wall_vacuum with cylinder shape made of Vacuum
LIST: Children for Volume 4 = [1,2,5]
LIST: Direct_children for Volume 4 = [1,2]
LIST: Intersect_check_list for Volume 4 = [1,2]
LIST: Mask_intersect_list for Volume 4 = []
LIST: Destinations_list for Volume 4 = [3]
LIST: Reduced_destinations_list for Volume 4 = [3]
LIST: Next_volume_list for Volume 4 = [3,1,2]
Is_vacuum for Volume 4 = 1
is_mask_volume for Volume 4 = 0
is_masked_volume for Volume 4 = 0
is_exit_volume for Volume 4 = 0
LIST: mask_list for Volume 4 = []
LIST: masked_by_list for Volume 4 = []
LIST: masked_by_mask_index_list for Volume 4 = []
mask_mode for Volume 4 = 0
List overview for sample_volume_replace with cylinder shape made of Vacuum
LIST: Children for Volume 5 = []
LIST: Direct_children for Volume 5 = []
LIST: Intersect_check_list for Volume 5 = []
LIST: Mask_intersect_list for Volume 5 = []
LIST: Destinations_list for Volume 5 = [1,2]
LIST: Reduced_destinations_list for Volume 5 = [1,2]
LIST: Next_volume_list for Volume 5 = [1,2]
Is_vacuum for Volume 5 = 1
is_mask_volume for Volume 5 = 0
is_masked_volume for Volume 5 = 0
is_exit_volume for Volume 5 = 0
LIST: mask_list for Volume 5 = []
LIST: masked_by_list for Volume 5 = []
LIST: masked_by_mask_index_list for Volume 5 = []
mask_mode for Volume 5 = 0
Union_master component master_after_sample initialized sucessfully
WARNING: Ray started in volume ''sample_volume_replace'' rather than the surrounding vacuum in component master_after_sample. This warning is only shown once.
Detector: logger_space_zx_I=3331.61 logger_space_zx_ERR=12.9945 logger_space_zx_N=193484 "logger_zx.dat"
Detector: logger_space_zy_I=3331.61 logger_space_zy_ERR=12.9945 logger_space_zy_N=193484 "logger_zy.dat"
Detector: banana_I=1640.63 banana_ERR=5.92943 banana_N=301427 "banana_1682428104.th"
PowderN: sample: Info: you may highly improve the computation efficiency by using
SPLIT 37 COMPONENT sample=PowderN(...)
in the instrument description python_tutorial.instr.
INFO: Placing instr file copy python_tutorial.instr in dataset /Users/madsbertelsen/PaNOSC/McStasScript/github/McStasScript/docs/source/tutorial/data_folder/union_external_6
loading system configuration
ms.name_plot_options("logger_space_zx", data, log=True, orders_of_mag=4)
ms.name_plot_options("logger_space_zy", data, log=True, orders_of_mag=4)
ms.make_sub_plot(data[0:2])
ms.make_sub_plot(data[2])
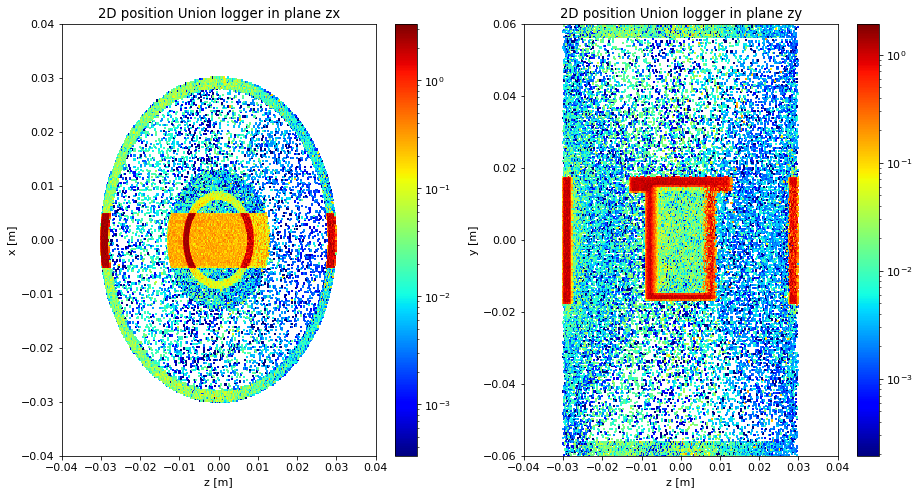
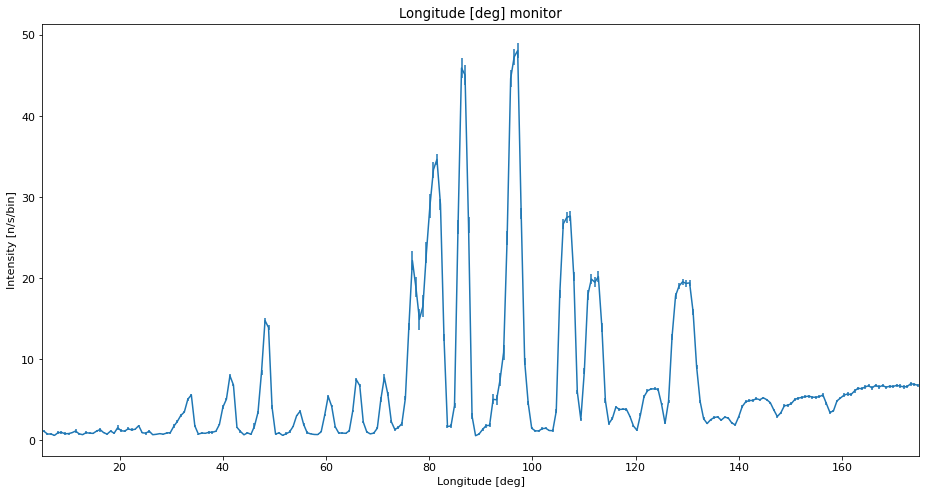
Interpretation of the data¶
Now we see evidence of the beam leaving the sample environment after interacting with the sample, and also elevated scattering in comparison to the empty can. This is now a reasonable simulation containing an external component inside a Union geometry ensemble, but there is still one problem, if the ray leaves the external component and reenters later, it will find a Vacuum instead of that sample. This can be fixed to some extend by adding a second copy of the external component and a third Union_master component, while incrementing the number_of_activations on all geometries, then the ray would be able to leave and enter the external component once before the external component effectively disappears. Even with this assumption, it is still a reasonable approximation and a flexible approach to add for example a mirror inside a sample environment.
Comparison of the three datasets¶
Here we compare the three datasets, the empty sample environment, the wrong simulation where rays scattered in the sample could not interact with the sample environment, and the full simulation.
banana_empty = ms.name_search("banana", data_empty)
banana_wrong = ms.name_search("banana", data_wrong)
banana_sample = ms.name_search("banana", data)
import matplotlib.pyplot as plt
plt.figure(figsize=(14,6))
plt.plot(banana_empty.xaxis, banana_empty.Intensity, "r",
banana_wrong.xaxis, banana_wrong.Intensity, "b",
banana_sample.xaxis, banana_sample.Intensity, "k")
plt.xlabel("2Theta [deg]")
plt.ylabel("Intensity [n/s]")
plt.legend(["No sample", "Wrong simulation, no exit", "Full simulation"])
<matplotlib.legend.Legend at 0x7ff1b0602490>
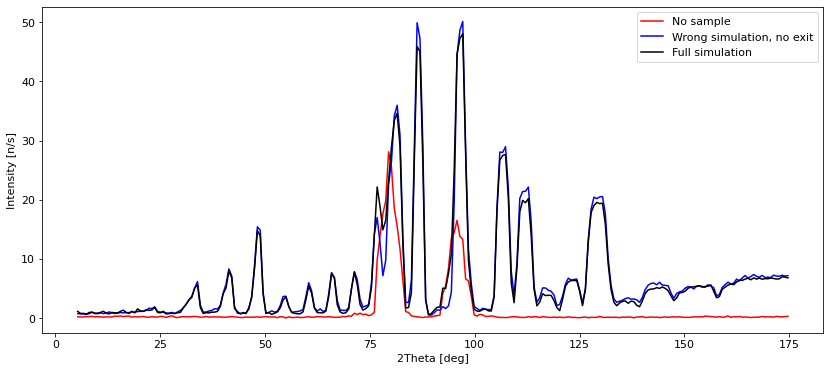
Interpretation of the data¶
We see that the wrong simulation have slightly lower background, and more peak intensity. We also see the peak shape is different near the aluminium Bragg peaks. Since the Union components contain a powder process, one can also recreate this example without using an external PowderN component to check the accuracy of the approach. It is however not a fair comparison, as the Union powder process will perform multiple scattering where PowderN will not.