Visualizing what happens in Union master
Contents
Visualizing what happens in Union master¶
One disadvantage to collecting all the simulation in the Union_master component, is that it is not possible to insert monitors between the parts to check on the beam. This issue is addressed by adding logger components that can record scattering and absorption events that occurs during the simulation. This notebook will show examples on the usage of loggers and their features.
Set up materials and geometry to investigate¶
First we set up the same mock cryostat we created in the advanced geometry tutorial to have an interesting system to investigate using the loggers.
import mcstasscript as ms
instrument = ms.McStas_instr("python_tutorial", input_path="run_folder")
Al_inc = instrument.add_component("Al_inc", "Incoherent_process")
Al_inc.sigma = 0.0082
Al_inc.unit_cell_volume = 66.4
Al_pow = instrument.add_component("Al_pow", "Powder_process")
Al_pow.reflections = '"Al.laz"'
Al = instrument.add_component("Al", "Union_make_material")
Al.process_string = '"Al_inc,Al_pow"'
Al.my_absorption = 100*0.231/66.4 # barns [m^2 E-28]*Å^3[m^3 E-30]=[m E-2], factor 100
Sample_inc = instrument.add_component("Sample_inc", "Incoherent_process")
Sample_inc.sigma = 3.4176
Sample_inc.unit_cell_volume = 1079.1
Sample_pow = instrument.add_component("Sample_pow", "Powder_process")
Sample_pow.reflections = '"Na2Ca3Al2F14.laz"'
Sample = instrument.add_component("Sample", "Union_make_material")
Sample.process_string = '"Sample_inc,Sample_pow"'
Sample.my_absorption = 100*2.9464/1079.1
src = instrument.add_component("source", "Source_div")
src.xwidth = 0.01
src.yheight = 0.035
src.focus_aw = 0.01
src.focus_ah = 0.01
src.lambda0 = instrument.add_parameter("wavelength", value=5.0,
comment="Wavelength in [Ang]")
src.dlambda = "0.01*wavelength"
src.flux = 1E13
sample_geometry = instrument.add_component("sample_geometry", "Union_cylinder")
sample_geometry.yheight = 0.03
sample_geometry.radius = 0.0075
sample_geometry.material_string='"Sample"'
sample_geometry.priority = 100
sample_geometry.set_AT([0,0,1], RELATIVE=src)
container = instrument.add_component("sample_container", "Union_cylinder")
container.set_RELATIVE(sample_geometry)
container.yheight = 0.03+0.003 # 1.5 mm top and button
container.radius = 0.0075 + 0.0015 # 1.5 mm sides of container
container.material_string='"Al"'
container.priority = 99
container_lid = instrument.add_component("sample_container_lid", "Union_cylinder")
container_lid.set_AT([0, 0.0155, 0], RELATIVE=container)
container_lid.yheight = 0.004
container_lid.radius = 0.013
container_lid.material_string='"Al"'
container_lid.priority = 98
inner_wall = instrument.add_component("cryostat_wall", "Union_cylinder")
inner_wall.set_AT([0,0,0], RELATIVE=sample_geometry)
inner_wall.yheight = 0.12
inner_wall.radius = 0.03
inner_wall.material_string='"Al"'
inner_wall.priority = 80
inner_wall_vac = instrument.add_component("cryostat_wall_vacuum", "Union_cylinder")
inner_wall_vac.set_AT([0,0,0], RELATIVE=sample_geometry)
inner_wall_vac.yheight = 0.12 - 0.008
inner_wall_vac.radius = 0.03 - 0.002
inner_wall_vac.material_string='"Vacuum"'
inner_wall_vac.priority = 81
outer_wall = instrument.add_component("outer_cryostat_wall", "Union_cylinder")
outer_wall.set_AT([0,0,0], RELATIVE=sample_geometry)
outer_wall.yheight = 0.15
outer_wall.radius = 0.1
outer_wall.material_string='"Al"'
outer_wall.priority = 60
outer_wall_vac = instrument.add_component("outer_cryostat_wall_vacuum", "Union_cylinder")
outer_wall_vac.set_AT([0,0,0], RELATIVE=sample_geometry)
outer_wall_vac.yheight = 0.15 - 0.01
outer_wall_vac.radius = 0.1 - 0.003
outer_wall_vac.material_string='"Vacuum"'
outer_wall_vac.priority = 61
instrument.show_components()
Al_inc Incoherent_process
AT (0, 0, 0) ABSOLUTE
Al_pow Powder_process
AT (0, 0, 0) ABSOLUTE
Al Union_make_material
AT (0, 0, 0) ABSOLUTE
Sample_inc Incoherent_process
AT (0, 0, 0) ABSOLUTE
Sample_pow Powder_process
AT (0, 0, 0) ABSOLUTE
Sample Union_make_material
AT (0, 0, 0) ABSOLUTE
source Source_div
AT (0, 0, 0) ABSOLUTE
sample_geometry Union_cylinder
AT (0, 0, 1) RELATIVE source
sample_container Union_cylinder
AT (0, 0, 0) RELATIVE sample_geometry
sample_container_lid Union_cylinder
AT (0, 0.0155, 0) RELATIVE sample_container
cryostat_wall Union_cylinder
AT (0, 0, 0) RELATIVE sample_geometry
cryostat_wall_vacuum Union_cylinder
AT (0, 0, 0) RELATIVE sample_geometry
outer_cryostat_wall Union_cylinder
AT (0, 0, 0) RELATIVE sample_geometry
outer_cryostat_wall_vacuum Union_cylinder
AT (0, 0, 0) RELATIVE sample_geometry
instrument.available_components("union")
Here are all components in the union category.
AF_HB_1D_process Union_conditional_PSD
IncoherentPhonon_process Union_conditional_standard
Incoherent_process Union_cone
NCrystal_process Union_cylinder
PhononSimple_process Union_logger_1D
Powder_process Union_logger_2DQ
Single_crystal_process Union_logger_2D_kf
Template_process Union_logger_2D_kf_time
Texture_process Union_logger_2D_space
Union_abs_logger_1D_space Union_logger_2D_space_time
Union_abs_logger_1D_space_event Union_logger_3D_space
Union_abs_logger_1D_space_tof Union_make_material
Union_abs_logger_1D_space_tof_to_lambda Union_master
Union_abs_logger_2D_space Union_mesh
Union_abs_logger_event Union_sphere
Union_box
Adding Union logger components¶
Union logger components need to be added before the Union_master component, as the master need to record the necessary information when the simulation is being performed. There are two different kind of Union logger components, the loggers that record scattering and the abs_loggers that record absorption. They have similar parameters and user interface. Here is a list of the currently available loggers:
Union_logger_1D
Union_logger_2D_space
Union_logger_2D_space_time
Union_logger_3D_space
Union_logger_2D_kf
Union_logger_2D_kf_time
Union_logger_2DQ
Union_abs_logger_1D_space
Union_abs_logger_1D_space_tof
Union_abs_logger_2D_space
The most commonly used logger is probably the Union_logger_2D_space, this component records spatial distribution of scattering, here are the available parameters.
instrument.component_help("Union_logger_2D_space")
___ Help Union_logger_2D_space _____________________________________________________
|optional parameter|required parameter|default value|user specified value|
target_geometry = "NULL" [string] // Comma seperated list of geometry names
that will be logged, leave empty for all
volumes (even not defined yet)
target_process = "NULL" [string] // Comma seperated names of physical
processes, if volumes are selected, one can
select Union_process names
D_direction_1 = "x" [string] // Direction for first axis ("x", "y" or "z")
D1_min = -5.0 [1] // histogram boundery, min position value for first axis
D1_max = 5.0 [1] // histogram boundery, max position value for first axis
n1 = 90.0 [1] // number of bins for first axis
D_direction_2 = "z" [string] // Direction for second axis ("x", "y" or "z")
D2_min = -5.0 [1] // histogram boundery, min position value for second axis
D2_max = 5.0 [1] // histogram boundery, max position value for second axis
n2 = 90.0 [1] // number of bins for second axis
filename = "NULL" [string] // Filename for logging output
order_total = 0.0 [1] // Only log rays that scatter for the n'th time, 0 for
all orders
order_volume = 0.0 [1] // Only log rays that scatter for the n'th time in the
same geometry
order_volume_process = 0.0 [1] // Only log rays that scatter for the n'th time
in the same geometry, using the same process
logger_conditional_extend_index = -1.0 [1] // If a conditional is used with
this logger, the result of each
conditional calculation can be made
available in extend as a array called
"logger_conditional_extend", and one
would then acces
logger_conditional_extend[n] if
logger_conditional_extend_index is
set to n
-------------------------------------------------------------------------------------
Setting up a 2D_space logger¶
One can select which two axis to record using D_direction_1 and D_direction_2, and the range with for example D1_min and D1_max. When spatial information is recorded it is also important to place the logger at an appropriate position, here we center it on the sample position.
logger_zx = instrument.add_component("logger_space_zx", "Union_logger_2D_space")
logger_zx.set_RELATIVE(sample_geometry)
logger_zx.D_direction_1 = '"z"'
logger_zx.D1_min = -0.12
logger_zx.D1_max = 0.12
logger_zx.n1 = 300
logger_zx.D_direction_2 = '"x"'
logger_zx.D2_min = -0.12
logger_zx.D2_max = 0.12
logger_zx.n2 = 300
logger_zx.filename = '"logger_zx.dat"'
logger_zy = instrument.add_component("logger_space_zy", "Union_logger_2D_space")
logger_zy.set_RELATIVE(sample_geometry)
logger_zy.D_direction_1 = '"z"'
logger_zy.D1_min = -0.12
logger_zy.D1_max = 0.12
logger_zy.n1 = 300
logger_zy.D_direction_2 = '"y"'
logger_zy.D2_min = -0.12
logger_zy.D2_max = 0.12
logger_zy.n2 = 300
logger_zy.filename = '"logger_zy.dat"'
master = instrument.add_component("master", "Union_master")
Running the simulation¶
If mpi is installed, one can add mpi=N where N is the number of cores available to speed up the simulation.
instrument.set_parameters(wavelength=3.0)
instrument.settings(ncount=3E6, output_path="data_folder/union_loggers")
data = instrument.backengine()
INFO: Using directory: "/Users/madsbertelsen/PaNOSC/McStasScript/github/McStasScript/docs/source/tutorial/data_folder/union_loggers_4"
INFO: Regenerating c-file: python_tutorial.c
CFLAGS= -I@MCCODE_LIB@/share/ -I@MCCODE_LIB@/share/
INFO: Recompiling: ./python_tutorial.out
mccode-r.c:2837:3: warning: expression result unused [-Wunused-value]
*t0;
^~~
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1604:105: warning: incompatible function pointer types passing 'int (const struct saved_history_struct *, const struct saved_history_struct *)' to parameter of type 'int (* _Nonnull)(const void *, const void *)' [-Wincompatible-function-pointer-types]
qsort(total_history.saved_histories,total_history.used_elements,sizeof (struct saved_history_struct), Sample_compare_history_intensities);
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/stdlib.h:161:22: note: passing argument to parameter '__compar' here
int (* _Nonnull __compar)(const void *, const void *));
^
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1613:20: warning: incompatible pointer types passing 'struct saved_history_struct *' to parameter of type 'struct dynamic_history_list *' [-Wincompatible-pointer-types]
printf_history(&total_history.saved_histories[history_iterate]);
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1434:50: note: passing argument to parameter 'history' here
void printf_history(struct dynamic_history_list *history) {
^
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:2030:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:839:1: warning: non-void function does not return a value in all control paths [-Wreturn-type]
};
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:883:1: warning: non-void function does not return a value [-Wreturn-type]
};
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3274:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3274:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3276:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3276:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3278:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3278:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3280:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3280:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:14067:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zx_filename
^~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:14067:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zx_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:14310:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zy_filename
^~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:14310:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zy_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:788:15: warning: left operand of comma operator has no effect [-Wunused-value]
if (volume_index_main,Volumes[volume_index_main]->geometry.is_mask_volume == 0 ||
^~~~~~~~~~~~~~~~~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:788:90: warning: left operand of comma operator has no effect [-Wunused-value]
if (volume_index_main,Volumes[volume_index_main]->geometry.is_mask_volume == 0 ||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~^~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:789:92: warning: left operand of comma operator has no effect [-Wunused-value]
volume_index_main,Volumes[volume_index_main]->geometry.is_masked_volume == 0 ||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~^~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
14 warnings generated.
INFO: ===
Opening input file '/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//data/Al.laz' (Table_Read_Offset)
Table from file 'Al.laz' (block 1) is 26 x 18 (x=1:8), constant step. interpolation: linear
'# TITLE *Aluminum-Al-[FM3-M] Miller, H.P.jr.;DuMond, J.W.M.[1942] at 298 K; ...'
PowderN: Al_pow: Reading 26 rows from Al.laz
PowderN: Al_pow: Read 26 reflections from file 'Al.laz'
PowderN: Al_pow: Vc=66.4 [Angs] sigma_abs=0.924 [barn] sigma_inc=0.0328 [barn] reflections=Al.laz
Opening input file '/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//data/Na2Ca3Al2F14.laz' (Table_Read_Offset)
Table from file 'Na2Ca3Al2F14.laz' (block 1) is 841 x 18 (x=1:20), constant step. interpolation: linear
'# TITLE *-Na2Ca3Al2F14-[I213] Courbion, G.;Ferey, G.[1988] Standard NAC cal ...'
PowderN: Sample_pow: Reading 841 rows from Na2Ca3Al2F14.laz
PowderN: Sample_pow: Read 841 reflections from file 'Na2Ca3Al2F14.laz'
PowderN: Sample_pow: Vc=1079.1 [Angs] sigma_abs=11.7856 [barn] sigma_inc=13.6704 [barn] reflections=Na2Ca3Al2F14.laz
---------------------------------------------------------------------
global_process_list.num_elements: 4
name of process [0]: Al_inc
component index [0]: 1
name of process [1]: Al_pow
component index [1]: 2
name of process [2]: Sample_inc
component index [2]: 4
name of process [3]: Sample_pow
component index [3]: 5
---------------------------------------------------------------------
global_material_list.num_elements: 2
name of material [0]: Al
component index [0]: 3
my_absoprtion [0]: 0.347892
number of processes [0]: 2
name of material [1]: Sample
component index [1]: 6
my_absoprtion [1]: 0.273042
number of processes [1]: 2
---------------------------------------------------------------------
global_geometry_list.num_elements: 2
name of geometry [0]: sample_geometry
component index [0]: 8
Volume.name [0]: sample_geometry
Volume.p_physics.is_vacuum [0]: 0
Volume.p_physics.my_absorption [0]: 0.273042
Volume.p_physics.number of processes [0]: 2
Volume.geometry.shape [0]: cylinder
Volume.geometry.center.x [0]: 0.000000
Volume.geometry.center.y [0]: 0.000000
Volume.geometry.center.z [0]: 1.000000
Volume.geometry.rotation_matrix[0] [0]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [0]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [0]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [0]: 0.007500
Volume.geometry.geometry_parameters.height [0]: 0.030000
Volume.geometry.focus_data_array.elements[0].Aim [0]: [0.000000 0.000000 1.000000]
name of geometry [1]: sample_container
component index [1]: 9
Volume.name [1]: sample_container
Volume.p_physics.is_vacuum [1]: 0
Volume.p_physics.my_absorption [1]: 0.347892
Volume.p_physics.number of processes [1]: 2
Volume.geometry.shape [1]: cylinder
Volume.geometry.center.x [1]: 0.000000
Volume.geometry.center.y [1]: 0.000000
Volume.geometry.center.z [1]: 1.000000
Volume.geometry.rotation_matrix[0] [1]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [1]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [1]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [1]: 0.009000
Volume.geometry.geometry_parameters.height [1]: 0.033000
Volume.geometry.focus_data_array.elements[0].Aim [1]: [0.000000 0.000000 1.000000]
name of geometry [2]: sample_container_lid
component index [2]: 10
Volume.name [2]: sample_container_lid
Volume.p_physics.is_vacuum [2]: 0
Volume.p_physics.my_absorption [2]: 0.347892
Volume.p_physics.number of processes [2]: 2
Volume.geometry.shape [2]: cylinder
Volume.geometry.center.x [2]: 0.000000
Volume.geometry.center.y [2]: 0.015500
Volume.geometry.center.z [2]: 1.000000
Volume.geometry.rotation_matrix[0] [2]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [2]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [2]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [2]: 0.013000
Volume.geometry.geometry_parameters.height [2]: 0.004000
Volume.geometry.focus_data_array.elements[0].Aim [2]: [0.000000 0.000000 1.000000]
name of geometry [3]: cryostat_wall
component index [3]: 11
Volume.name [3]: cryostat_wall
Volume.p_physics.is_vacuum [3]: 0
Volume.p_physics.my_absorption [3]: 0.347892
Volume.p_physics.number of processes [3]: 2
Volume.geometry.shape [3]: cylinder
Volume.geometry.center.x [3]: 0.000000
Volume.geometry.center.y [3]: 0.000000
Volume.geometry.center.z [3]: 1.000000
Volume.geometry.rotation_matrix[0] [3]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [3]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [3]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [3]: 0.030000
Volume.geometry.geometry_parameters.height [3]: 0.120000
Volume.geometry.focus_data_array.elements[0].Aim [3]: [0.000000 0.000000 1.000000]
name of geometry [4]: cryostat_wall_vacuum
component index [4]: 12
Volume.name [4]: cryostat_wall_vacuum
Volume.p_physics.is_vacuum [4]: 1
Volume.p_physics.my_absorption [4]: 0.000000
Volume.p_physics.number of processes [4]: 0
Volume.geometry.shape [4]: cylinder
Volume.geometry.center.x [4]: 0.000000
Volume.geometry.center.y [4]: 0.000000
Volume.geometry.center.z [4]: 1.000000
Volume.geometry.rotation_matrix[0] [4]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [4]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [4]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [4]: 0.028000
Volume.geometry.geometry_parameters.height [4]: 0.112000
Volume.geometry.focus_data_array.elements[0].Aim [4]: [0.000000 0.000000 1.000000]
name of geometry [5]: outer_cryostat_wall
component index [5]: 13
Volume.name [5]: outer_cryostat_wall
Volume.p_physics.is_vacuum [5]: 0
Volume.p_physics.my_absorption [5]: 0.347892
Volume.p_physics.number of processes [5]: 2
Volume.geometry.shape [5]: cylinder
Volume.geometry.center.x [5]: 0.000000
Volume.geometry.center.y [5]: 0.000000
Volume.geometry.center.z [5]: 1.000000
Volume.geometry.rotation_matrix[0] [5]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [5]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [5]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [5]: 0.100000
Volume.geometry.geometry_parameters.height [5]: 0.150000
Volume.geometry.focus_data_array.elements[0].Aim [5]: [0.000000 0.000000 1.000000]
name of geometry [6]: outer_cryostat_wall_vacuum
component index [6]: 14
Volume.name [6]: outer_cryostat_wall_vacuum
Volume.p_physics.is_vacuum [6]: 1
Volume.p_physics.my_absorption [6]: 0.000000
Volume.p_physics.number of processes [6]: 0
Volume.geometry.shape [6]: cylinder
Volume.geometry.center.x [6]: 0.000000
Volume.geometry.center.y [6]: 0.000000
Volume.geometry.center.z [6]: 1.000000
Volume.geometry.rotation_matrix[0] [6]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [6]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [6]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [6]: 0.097000
Volume.geometry.geometry_parameters.height [6]: 0.140000
Volume.geometry.focus_data_array.elements[0].Aim [6]: [0.000000 0.000000 1.000000]
---------------------------------------------------------------------
number_of_volumes = 8
number_of_masks = 0
number_of_masked_volumes = 0
---- Overview of the lists generated for each volume ----
List overview for surrounding vacuum
LIST: Children for Volume 0 = [1,2,3,4,5,6,7]
LIST: Direct_children for Volume 0 = [6]
LIST: Intersect_check_list for Volume 0 = [6]
LIST: Mask_intersect_list for Volume 0 = []
LIST: Destinations_list for Volume 0 = []
LIST: Reduced_destinations_list for Volume 0 = []
LIST: Next_volume_list for Volume 0 = [6]
LIST: mask_list for Volume 0 = []
LIST: masked_by_list for Volume 0 = []
LIST: masked_by_mask_index_list for Volume 0 = []
mask_mode for Volume 0 = 0
List overview for sample_geometry with cylinder shape made of Sample
LIST: Children for Volume 1 = []
LIST: Direct_children for Volume 1 = []
LIST: Intersect_check_list for Volume 1 = []
LIST: Mask_intersect_list for Volume 1 = []
LIST: Destinations_list for Volume 1 = [2,3]
LIST: Reduced_destinations_list for Volume 1 = [2,3]
LIST: Next_volume_list for Volume 1 = [2,3]
Is_vacuum for Volume 1 = 0
is_mask_volume for Volume 1 = 0
is_masked_volume for Volume 1 = 0
is_exit_volume for Volume 1 = 0
LIST: mask_list for Volume 1 = []
LIST: masked_by_list for Volume 1 = []
LIST: masked_by_mask_index_list for Volume 1 = []
mask_mode for Volume 1 = 0
List overview for sample_container with cylinder shape made of Al
LIST: Children for Volume 2 = [1]
LIST: Direct_children for Volume 2 = [1]
LIST: Intersect_check_list for Volume 2 = [1]
LIST: Mask_intersect_list for Volume 2 = []
LIST: Destinations_list for Volume 2 = [3,5]
LIST: Reduced_destinations_list for Volume 2 = [5]
LIST: Next_volume_list for Volume 2 = [3,5,1]
Is_vacuum for Volume 2 = 0
is_mask_volume for Volume 2 = 0
is_masked_volume for Volume 2 = 0
is_exit_volume for Volume 2 = 0
LIST: mask_list for Volume 2 = []
LIST: masked_by_list for Volume 2 = []
LIST: masked_by_mask_index_list for Volume 2 = []
mask_mode for Volume 2 = 0
List overview for sample_container_lid with cylinder shape made of Al
LIST: Children for Volume 3 = []
LIST: Direct_children for Volume 3 = []
LIST: Intersect_check_list for Volume 3 = [2]
LIST: Mask_intersect_list for Volume 3 = []
LIST: Destinations_list for Volume 3 = [5]
LIST: Reduced_destinations_list for Volume 3 = [5]
LIST: Next_volume_list for Volume 3 = [5,2]
Is_vacuum for Volume 3 = 0
is_mask_volume for Volume 3 = 0
is_masked_volume for Volume 3 = 0
is_exit_volume for Volume 3 = 0
LIST: mask_list for Volume 3 = []
LIST: masked_by_list for Volume 3 = []
LIST: masked_by_mask_index_list for Volume 3 = []
mask_mode for Volume 3 = 0
List overview for cryostat_wall with cylinder shape made of Al
LIST: Children for Volume 4 = [1,2,3,5]
LIST: Direct_children for Volume 4 = [5]
LIST: Intersect_check_list for Volume 4 = [5]
LIST: Mask_intersect_list for Volume 4 = []
LIST: Destinations_list for Volume 4 = [7]
LIST: Reduced_destinations_list for Volume 4 = [7]
LIST: Next_volume_list for Volume 4 = [7,5]
Is_vacuum for Volume 4 = 0
is_mask_volume for Volume 4 = 0
is_masked_volume for Volume 4 = 0
is_exit_volume for Volume 4 = 0
LIST: mask_list for Volume 4 = []
LIST: masked_by_list for Volume 4 = []
LIST: masked_by_mask_index_list for Volume 4 = []
mask_mode for Volume 4 = 0
List overview for cryostat_wall_vacuum with cylinder shape made of Vacuum
LIST: Children for Volume 5 = [1,2,3]
LIST: Direct_children for Volume 5 = [2,3]
LIST: Intersect_check_list for Volume 5 = [2,3]
LIST: Mask_intersect_list for Volume 5 = []
LIST: Destinations_list for Volume 5 = [4]
LIST: Reduced_destinations_list for Volume 5 = [4]
LIST: Next_volume_list for Volume 5 = [4,2,3]
Is_vacuum for Volume 5 = 1
is_mask_volume for Volume 5 = 0
is_masked_volume for Volume 5 = 0
is_exit_volume for Volume 5 = 0
LIST: mask_list for Volume 5 = []
LIST: masked_by_list for Volume 5 = []
LIST: masked_by_mask_index_list for Volume 5 = []
mask_mode for Volume 5 = 0
List overview for outer_cryostat_wall with cylinder shape made of Al
LIST: Children for Volume 6 = [1,2,3,4,5,7]
LIST: Direct_children for Volume 6 = [7]
LIST: Intersect_check_list for Volume 6 = [7]
LIST: Mask_intersect_list for Volume 6 = []
LIST: Destinations_list for Volume 6 = [0]
LIST: Reduced_destinations_list for Volume 6 = []
LIST: Next_volume_list for Volume 6 = [0,7]
Is_vacuum for Volume 6 = 0
is_mask_volume for Volume 6 = 0
is_masked_volume for Volume 6 = 0
is_exit_volume for Volume 6 = 0
LIST: mask_list for Volume 6 = []
LIST: masked_by_list for Volume 6 = []
LIST: masked_by_mask_index_list for Volume 6 = []
mask_mode for Volume 6 = 0
List overview for outer_cryostat_wall_vacuum with cylinder shape made of Vacuum
LIST: Children for Volume 7 = [1,2,3,4,5]
LIST: Direct_children for Volume 7 = [4]
LIST: Intersect_check_list for Volume 7 = [4]
LIST: Mask_intersect_list for Volume 7 = []
LIST: Destinations_list for Volume 7 = [6]
LIST: Reduced_destinations_list for Volume 7 = [6]
LIST: Next_volume_list for Volume 7 = [6,4]
Is_vacuum for Volume 7 = 1
is_mask_volume for Volume 7 = 0
is_masked_volume for Volume 7 = 0
is_exit_volume for Volume 7 = 0
LIST: mask_list for Volume 7 = []
LIST: masked_by_list for Volume 7 = []
LIST: masked_by_mask_index_list for Volume 7 = []
mask_mode for Volume 7 = 0
Union_master component master initialized sucessfully
Detector: logger_space_zx_I=20555.8 logger_space_zx_ERR=33.6068 logger_space_zx_N=1.01183e+06 "logger_zx.dat"
Detector: logger_space_zy_I=20555.8 logger_space_zy_ERR=33.6068 logger_space_zy_N=1.01183e+06 "logger_zy.dat"
INFO: Placing instr file copy python_tutorial.instr in dataset /Users/madsbertelsen/PaNOSC/McStasScript/github/McStasScript/docs/source/tutorial/data_folder/union_loggers_4
loading system configuration
ms.make_sub_plot(data, log=True, orders_of_mag=4)
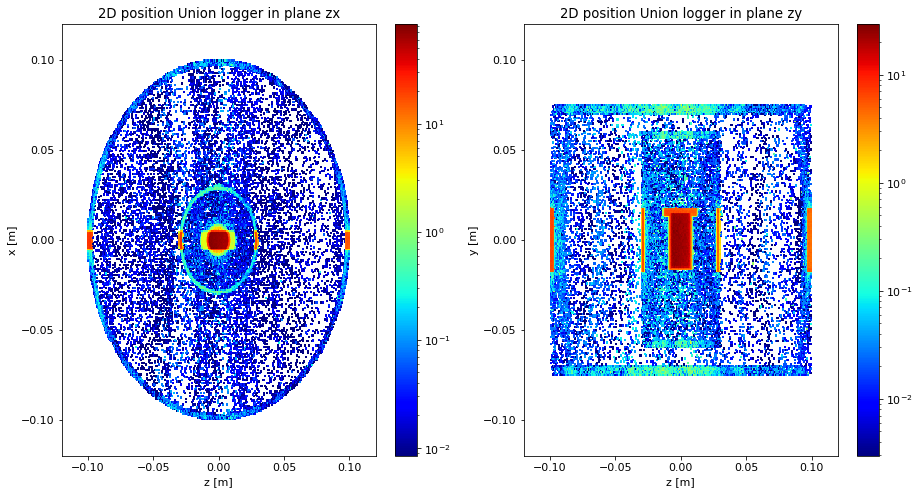
Interpreting the data¶
The zx logger views the cryostat from the top, while the zy loggers shows it from the side. These are histograms of scattered intensity, and it is clear the majority of the scattering happens in the direct beam. There are however scattering events in all parts of our mock cryostat, as neutrons that scattered in either the sample or cryostat walls could go in any direction due to the incoherent scattering. The aluminium and sample also have powder scattering, so some patterns can be seen from the debye scherrer cones.
Logger targets¶
It is possible to attach a logger to a certain geometry, or even a list of geometries using the target_geometry parameter. In that way one can for example view the scattering in the sample environment, while ignoring the sample. It is also possible to select a number of specific scattering processes to investigate with the target_process parameter. This is especially useful when working with a single crystal process, that only scatters when the Bragg condition is met.
Let us modify our existing loggers to view certain parts of the simulated system, and then rerun the simulation. If mpi is installed, one can add mpi=N where N is the number of cores available to speed up the simulation.
logger_zx.target_geometry = '"outer_cryostat_wall,cryostat_wall"'
logger_zy.target_geometry = '"sample_geometry"'
data = instrument.backengine()
INFO: Using directory: "/Users/madsbertelsen/PaNOSC/McStasScript/github/McStasScript/docs/source/tutorial/data_folder/union_loggers_5"
INFO: Regenerating c-file: python_tutorial.c
CFLAGS= -I@MCCODE_LIB@/share/ -I@MCCODE_LIB@/share/
INFO: Recompiling: ./python_tutorial.out
mccode-r.c:2837:3: warning: expression result unused [-Wunused-value]
*t0;
^~~
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1604:105: warning: incompatible function pointer types passing 'int (const struct saved_history_struct *, const struct saved_history_struct *)' to parameter of type 'int (* _Nonnull)(const void *, const void *)' [-Wincompatible-function-pointer-types]
qsort(total_history.saved_histories,total_history.used_elements,sizeof (struct saved_history_struct), Sample_compare_history_intensities);
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/stdlib.h:161:22: note: passing argument to parameter '__compar' here
int (* _Nonnull __compar)(const void *, const void *));
^
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1613:20: warning: incompatible pointer types passing 'struct saved_history_struct *' to parameter of type 'struct dynamic_history_list *' [-Wincompatible-pointer-types]
printf_history(&total_history.saved_histories[history_iterate]);
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1434:50: note: passing argument to parameter 'history' here
void printf_history(struct dynamic_history_list *history) {
^
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:2030:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:839:1: warning: non-void function does not return a value in all control paths [-Wreturn-type]
};
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:883:1: warning: non-void function does not return a value [-Wreturn-type]
};
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3274:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3274:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3276:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3276:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3278:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3278:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3280:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3280:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:14067:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zx_filename
^~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:14067:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zx_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:14310:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zy_filename
^~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:14310:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zy_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:788:15: warning: left operand of comma operator has no effect [-Wunused-value]
if (volume_index_main,Volumes[volume_index_main]->geometry.is_mask_volume == 0 ||
^~~~~~~~~~~~~~~~~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:788:90: warning: left operand of comma operator has no effect [-Wunused-value]
if (volume_index_main,Volumes[volume_index_main]->geometry.is_mask_volume == 0 ||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~^~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:789:92: warning: left operand of comma operator has no effect [-Wunused-value]
volume_index_main,Volumes[volume_index_main]->geometry.is_masked_volume == 0 ||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~^~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
14 warnings generated.
INFO: ===
Opening input file '/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//data/Al.laz' (Table_Read_Offset)
Table from file 'Al.laz' (block 1) is 26 x 18 (x=1:8), constant step. interpolation: linear
'# TITLE *Aluminum-Al-[FM3-M] Miller, H.P.jr.;DuMond, J.W.M.[1942] at 298 K; ...'
PowderN: Al_pow: Reading 26 rows from Al.laz
PowderN: Al_pow: Read 26 reflections from file 'Al.laz'
PowderN: Al_pow: Vc=66.4 [Angs] sigma_abs=0.924 [barn] sigma_inc=0.0328 [barn] reflections=Al.laz
Opening input file '/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//data/Na2Ca3Al2F14.laz' (Table_Read_Offset)
Table from file 'Na2Ca3Al2F14.laz' (block 1) is 841 x 18 (x=1:20), constant step. interpolation: linear
'# TITLE *-Na2Ca3Al2F14-[I213] Courbion, G.;Ferey, G.[1988] Standard NAC cal ...'
PowderN: Sample_pow: Reading 841 rows from Na2Ca3Al2F14.laz
PowderN: Sample_pow: Read 841 reflections from file 'Na2Ca3Al2F14.laz'
PowderN: Sample_pow: Vc=1079.1 [Angs] sigma_abs=11.7856 [barn] sigma_inc=13.6704 [barn] reflections=Na2Ca3Al2F14.laz
---------------------------------------------------------------------
global_process_list.num_elements: 4
name of process [0]: Al_inc
component index [0]: 1
name of process [1]: Al_pow
component index [1]: 2
name of process [2]: Sample_inc
component index [2]: 4
name of process [3]: Sample_pow
component index [3]: 5
---------------------------------------------------------------------
global_material_list.num_elements: 2
name of material [0]: Al
component index [0]: 3
my_absoprtion [0]: 0.347892
number of processes [0]: 2
name of material [1]: Sample
component index [1]: 6
my_absoprtion [1]: 0.273042
number of processes [1]: 2
---------------------------------------------------------------------
global_geometry_list.num_elements: 2
name of geometry [0]: sample_geometry
component index [0]: 8
Volume.name [0]: sample_geometry
Volume.p_physics.is_vacuum [0]: 0
Volume.p_physics.my_absorption [0]: 0.273042
Volume.p_physics.number of processes [0]: 2
Volume.geometry.shape [0]: cylinder
Volume.geometry.center.x [0]: 0.000000
Volume.geometry.center.y [0]: 0.000000
Volume.geometry.center.z [0]: 1.000000
Volume.geometry.rotation_matrix[0] [0]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [0]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [0]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [0]: 0.007500
Volume.geometry.geometry_parameters.height [0]: 0.030000
Volume.geometry.focus_data_array.elements[0].Aim [0]: [0.000000 0.000000 1.000000]
name of geometry [1]: sample_container
component index [1]: 9
Volume.name [1]: sample_container
Volume.p_physics.is_vacuum [1]: 0
Volume.p_physics.my_absorption [1]: 0.347892
Volume.p_physics.number of processes [1]: 2
Volume.geometry.shape [1]: cylinder
Volume.geometry.center.x [1]: 0.000000
Volume.geometry.center.y [1]: 0.000000
Volume.geometry.center.z [1]: 1.000000
Volume.geometry.rotation_matrix[0] [1]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [1]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [1]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [1]: 0.009000
Volume.geometry.geometry_parameters.height [1]: 0.033000
Volume.geometry.focus_data_array.elements[0].Aim [1]: [0.000000 0.000000 1.000000]
name of geometry [2]: sample_container_lid
component index [2]: 10
Volume.name [2]: sample_container_lid
Volume.p_physics.is_vacuum [2]: 0
Volume.p_physics.my_absorption [2]: 0.347892
Volume.p_physics.number of processes [2]: 2
Volume.geometry.shape [2]: cylinder
Volume.geometry.center.x [2]: 0.000000
Volume.geometry.center.y [2]: 0.015500
Volume.geometry.center.z [2]: 1.000000
Volume.geometry.rotation_matrix[0] [2]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [2]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [2]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [2]: 0.013000
Volume.geometry.geometry_parameters.height [2]: 0.004000
Volume.geometry.focus_data_array.elements[0].Aim [2]: [0.000000 0.000000 1.000000]
name of geometry [3]: cryostat_wall
component index [3]: 11
Volume.name [3]: cryostat_wall
Volume.p_physics.is_vacuum [3]: 0
Volume.p_physics.my_absorption [3]: 0.347892
Volume.p_physics.number of processes [3]: 2
Volume.geometry.shape [3]: cylinder
Volume.geometry.center.x [3]: 0.000000
Volume.geometry.center.y [3]: 0.000000
Volume.geometry.center.z [3]: 1.000000
Volume.geometry.rotation_matrix[0] [3]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [3]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [3]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [3]: 0.030000
Volume.geometry.geometry_parameters.height [3]: 0.120000
Volume.geometry.focus_data_array.elements[0].Aim [3]: [0.000000 0.000000 1.000000]
name of geometry [4]: cryostat_wall_vacuum
component index [4]: 12
Volume.name [4]: cryostat_wall_vacuum
Volume.p_physics.is_vacuum [4]: 1
Volume.p_physics.my_absorption [4]: 0.000000
Volume.p_physics.number of processes [4]: 0
Volume.geometry.shape [4]: cylinder
Volume.geometry.center.x [4]: 0.000000
Volume.geometry.center.y [4]: 0.000000
Volume.geometry.center.z [4]: 1.000000
Volume.geometry.rotation_matrix[0] [4]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [4]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [4]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [4]: 0.028000
Volume.geometry.geometry_parameters.height [4]: 0.112000
Volume.geometry.focus_data_array.elements[0].Aim [4]: [0.000000 0.000000 1.000000]
name of geometry [5]: outer_cryostat_wall
component index [5]: 13
Volume.name [5]: outer_cryostat_wall
Volume.p_physics.is_vacuum [5]: 0
Volume.p_physics.my_absorption [5]: 0.347892
Volume.p_physics.number of processes [5]: 2
Volume.geometry.shape [5]: cylinder
Volume.geometry.center.x [5]: 0.000000
Volume.geometry.center.y [5]: 0.000000
Volume.geometry.center.z [5]: 1.000000
Volume.geometry.rotation_matrix[0] [5]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [5]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [5]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [5]: 0.100000
Volume.geometry.geometry_parameters.height [5]: 0.150000
Volume.geometry.focus_data_array.elements[0].Aim [5]: [0.000000 0.000000 1.000000]
name of geometry [6]: outer_cryostat_wall_vacuum
component index [6]: 14
Volume.name [6]: outer_cryostat_wall_vacuum
Volume.p_physics.is_vacuum [6]: 1
Volume.p_physics.my_absorption [6]: 0.000000
Volume.p_physics.number of processes [6]: 0
Volume.geometry.shape [6]: cylinder
Volume.geometry.center.x [6]: 0.000000
Volume.geometry.center.y [6]: 0.000000
Volume.geometry.center.z [6]: 1.000000
Volume.geometry.rotation_matrix[0] [6]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [6]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [6]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [6]: 0.097000
Volume.geometry.geometry_parameters.height [6]: 0.140000
Volume.geometry.focus_data_array.elements[0].Aim [6]: [0.000000 0.000000 1.000000]
---------------------------------------------------------------------
number_of_volumes = 8
number_of_masks = 0
number_of_masked_volumes = 0
---- Overview of the lists generated for each volume ----
List overview for surrounding vacuum
LIST: Children for Volume 0 = [1,2,3,4,5,6,7]
LIST: Direct_children for Volume 0 = [6]
LIST: Intersect_check_list for Volume 0 = [6]
LIST: Mask_intersect_list for Volume 0 = []
LIST: Destinations_list for Volume 0 = []
LIST: Reduced_destinations_list for Volume 0 = []
LIST: Next_volume_list for Volume 0 = [6]
LIST: mask_list for Volume 0 = []
LIST: masked_by_list for Volume 0 = []
LIST: masked_by_mask_index_list for Volume 0 = []
mask_mode for Volume 0 = 0
List overview for sample_geometry with cylinder shape made of Sample
LIST: Children for Volume 1 = []
LIST: Direct_children for Volume 1 = []
LIST: Intersect_check_list for Volume 1 = []
LIST: Mask_intersect_list for Volume 1 = []
LIST: Destinations_list for Volume 1 = [2,3]
LIST: Reduced_destinations_list for Volume 1 = [2,3]
LIST: Next_volume_list for Volume 1 = [2,3]
Is_vacuum for Volume 1 = 0
is_mask_volume for Volume 1 = 0
is_masked_volume for Volume 1 = 0
is_exit_volume for Volume 1 = 0
LIST: mask_list for Volume 1 = []
LIST: masked_by_list for Volume 1 = []
LIST: masked_by_mask_index_list for Volume 1 = []
mask_mode for Volume 1 = 0
List overview for sample_container with cylinder shape made of Al
LIST: Children for Volume 2 = [1]
LIST: Direct_children for Volume 2 = [1]
LIST: Intersect_check_list for Volume 2 = [1]
LIST: Mask_intersect_list for Volume 2 = []
LIST: Destinations_list for Volume 2 = [3,5]
LIST: Reduced_destinations_list for Volume 2 = [5]
LIST: Next_volume_list for Volume 2 = [3,5,1]
Is_vacuum for Volume 2 = 0
is_mask_volume for Volume 2 = 0
is_masked_volume for Volume 2 = 0
is_exit_volume for Volume 2 = 0
LIST: mask_list for Volume 2 = []
LIST: masked_by_list for Volume 2 = []
LIST: masked_by_mask_index_list for Volume 2 = []
mask_mode for Volume 2 = 0
List overview for sample_container_lid with cylinder shape made of Al
LIST: Children for Volume 3 = []
LIST: Direct_children for Volume 3 = []
LIST: Intersect_check_list for Volume 3 = [2]
LIST: Mask_intersect_list for Volume 3 = []
LIST: Destinations_list for Volume 3 = [5]
LIST: Reduced_destinations_list for Volume 3 = [5]
LIST: Next_volume_list for Volume 3 = [5,2]
Is_vacuum for Volume 3 = 0
is_mask_volume for Volume 3 = 0
is_masked_volume for Volume 3 = 0
is_exit_volume for Volume 3 = 0
LIST: mask_list for Volume 3 = []
LIST: masked_by_list for Volume 3 = []
LIST: masked_by_mask_index_list for Volume 3 = []
mask_mode for Volume 3 = 0
List overview for cryostat_wall with cylinder shape made of Al
LIST: Children for Volume 4 = [1,2,3,5]
LIST: Direct_children for Volume 4 = [5]
LIST: Intersect_check_list for Volume 4 = [5]
LIST: Mask_intersect_list for Volume 4 = []
LIST: Destinations_list for Volume 4 = [7]
LIST: Reduced_destinations_list for Volume 4 = [7]
LIST: Next_volume_list for Volume 4 = [7,5]
Is_vacuum for Volume 4 = 0
is_mask_volume for Volume 4 = 0
is_masked_volume for Volume 4 = 0
is_exit_volume for Volume 4 = 0
LIST: mask_list for Volume 4 = []
LIST: masked_by_list for Volume 4 = []
LIST: masked_by_mask_index_list for Volume 4 = []
mask_mode for Volume 4 = 0
List overview for cryostat_wall_vacuum with cylinder shape made of Vacuum
LIST: Children for Volume 5 = [1,2,3]
LIST: Direct_children for Volume 5 = [2,3]
LIST: Intersect_check_list for Volume 5 = [2,3]
LIST: Mask_intersect_list for Volume 5 = []
LIST: Destinations_list for Volume 5 = [4]
LIST: Reduced_destinations_list for Volume 5 = [4]
LIST: Next_volume_list for Volume 5 = [4,2,3]
Is_vacuum for Volume 5 = 1
is_mask_volume for Volume 5 = 0
is_masked_volume for Volume 5 = 0
is_exit_volume for Volume 5 = 0
LIST: mask_list for Volume 5 = []
LIST: masked_by_list for Volume 5 = []
LIST: masked_by_mask_index_list for Volume 5 = []
mask_mode for Volume 5 = 0
List overview for outer_cryostat_wall with cylinder shape made of Al
LIST: Children for Volume 6 = [1,2,3,4,5,7]
LIST: Direct_children for Volume 6 = [7]
LIST: Intersect_check_list for Volume 6 = [7]
LIST: Mask_intersect_list for Volume 6 = []
LIST: Destinations_list for Volume 6 = [0]
LIST: Reduced_destinations_list for Volume 6 = []
LIST: Next_volume_list for Volume 6 = [0,7]
Is_vacuum for Volume 6 = 0
is_mask_volume for Volume 6 = 0
is_masked_volume for Volume 6 = 0
is_exit_volume for Volume 6 = 0
LIST: mask_list for Volume 6 = []
LIST: masked_by_list for Volume 6 = []
LIST: masked_by_mask_index_list for Volume 6 = []
mask_mode for Volume 6 = 0
List overview for outer_cryostat_wall_vacuum with cylinder shape made of Vacuum
LIST: Children for Volume 7 = [1,2,3,4,5]
LIST: Direct_children for Volume 7 = [4]
LIST: Intersect_check_list for Volume 7 = [4]
LIST: Mask_intersect_list for Volume 7 = []
LIST: Destinations_list for Volume 7 = [6]
LIST: Reduced_destinations_list for Volume 7 = [6]
LIST: Next_volume_list for Volume 7 = [6,4]
Is_vacuum for Volume 7 = 1
is_mask_volume for Volume 7 = 0
is_masked_volume for Volume 7 = 0
is_exit_volume for Volume 7 = 0
LIST: mask_list for Volume 7 = []
LIST: masked_by_list for Volume 7 = []
LIST: masked_by_mask_index_list for Volume 7 = []
mask_mode for Volume 7 = 0
Union_master component master initialized sucessfully
Detector: logger_space_zx_I=3795.91 logger_space_zx_ERR=9.82006 logger_space_zx_N=199585 "logger_zx.dat"
Detector: logger_space_zy_I=14907.7 logger_space_zy_ERR=31.2444 logger_space_zy_N=713037 "logger_zy.dat"
INFO: Placing instr file copy python_tutorial.instr in dataset /Users/madsbertelsen/PaNOSC/McStasScript/github/McStasScript/docs/source/tutorial/data_folder/union_loggers_5
loading system configuration
ms.make_sub_plot(data, log=[True, False], orders_of_mag=4)
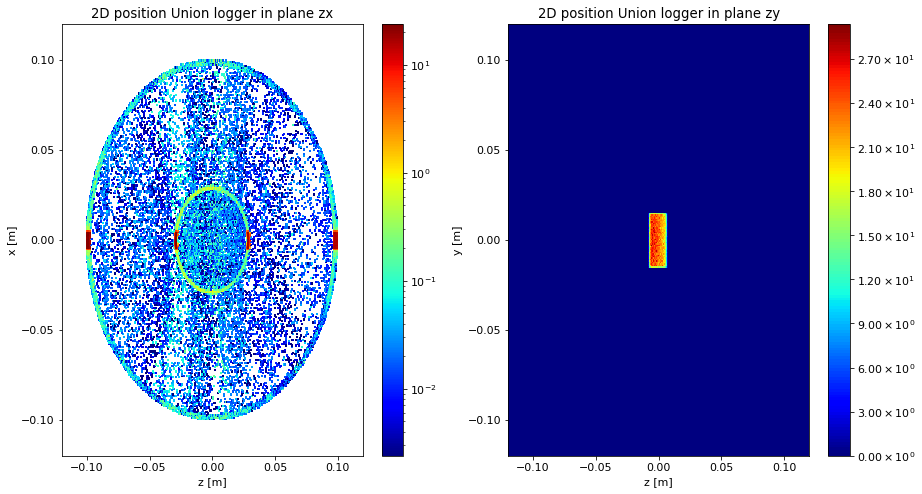
Scattering order¶
All loggers also have the option to only record given scattering orders. For example only record the second scattering.
order_total : Match given number of scattering events, counting all scattering events in the system
order_volume : Match given number of scattering events, only counting events in the current volume
order_volume_process : Match given number of scattering events, only counting events in current volume with current process
We can modify our previous loggers to test out these features. The zx logger viewing from above will keep the target, but we remove the sample target on the zy logger, which is done by setting the taget_geometry to NULL. We choose to look at the second scattering event.
logger_zx.order_total = 2
logger_zy.target_geometry = '"NULL"'
logger_zy.order_total = 2
data = instrument.backengine()
INFO: Using directory: "/Users/madsbertelsen/PaNOSC/McStasScript/github/McStasScript/docs/source/tutorial/data_folder/union_loggers_6"
INFO: Regenerating c-file: python_tutorial.c
CFLAGS= -I@MCCODE_LIB@/share/ -I@MCCODE_LIB@/share/
INFO: Recompiling: ./python_tutorial.out
mccode-r.c:2837:3: warning: expression result unused [-Wunused-value]
*t0;
^~~
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1604:105: warning: incompatible function pointer types passing 'int (const struct saved_history_struct *, const struct saved_history_struct *)' to parameter of type 'int (* _Nonnull)(const void *, const void *)' [-Wincompatible-function-pointer-types]
qsort(total_history.saved_histories,total_history.used_elements,sizeof (struct saved_history_struct), Sample_compare_history_intensities);
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/stdlib.h:161:22: note: passing argument to parameter '__compar' here
int (* _Nonnull __compar)(const void *, const void *));
^
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1613:20: warning: incompatible pointer types passing 'struct saved_history_struct *' to parameter of type 'struct dynamic_history_list *' [-Wincompatible-pointer-types]
printf_history(&total_history.saved_histories[history_iterate]);
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1434:50: note: passing argument to parameter 'history' here
void printf_history(struct dynamic_history_list *history) {
^
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:2030:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:839:1: warning: non-void function does not return a value in all control paths [-Wreturn-type]
};
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:883:1: warning: non-void function does not return a value [-Wreturn-type]
};
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3274:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3274:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3276:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3276:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3278:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3278:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3280:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3280:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:14067:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zx_filename
^~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:14067:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zx_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:14310:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zy_filename
^~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:14310:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zy_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:788:15: warning: left operand of comma operator has no effect [-Wunused-value]
if (volume_index_main,Volumes[volume_index_main]->geometry.is_mask_volume == 0 ||
^~~~~~~~~~~~~~~~~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:788:90: warning: left operand of comma operator has no effect [-Wunused-value]
if (volume_index_main,Volumes[volume_index_main]->geometry.is_mask_volume == 0 ||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~^~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:789:92: warning: left operand of comma operator has no effect [-Wunused-value]
volume_index_main,Volumes[volume_index_main]->geometry.is_masked_volume == 0 ||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~^~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
14 warnings generated.
INFO: ===
Opening input file '/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//data/Al.laz' (Table_Read_Offset)
Table from file 'Al.laz' (block 1) is 26 x 18 (x=1:8), constant step. interpolation: linear
'# TITLE *Aluminum-Al-[FM3-M] Miller, H.P.jr.;DuMond, J.W.M.[1942] at 298 K; ...'
PowderN: Al_pow: Reading 26 rows from Al.laz
PowderN: Al_pow: Read 26 reflections from file 'Al.laz'
PowderN: Al_pow: Vc=66.4 [Angs] sigma_abs=0.924 [barn] sigma_inc=0.0328 [barn] reflections=Al.laz
Opening input file '/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//data/Na2Ca3Al2F14.laz' (Table_Read_Offset)
Table from file 'Na2Ca3Al2F14.laz' (block 1) is 841 x 18 (x=1:20), constant step. interpolation: linear
'# TITLE *-Na2Ca3Al2F14-[I213] Courbion, G.;Ferey, G.[1988] Standard NAC cal ...'
PowderN: Sample_pow: Reading 841 rows from Na2Ca3Al2F14.laz
PowderN: Sample_pow: Read 841 reflections from file 'Na2Ca3Al2F14.laz'
PowderN: Sample_pow: Vc=1079.1 [Angs] sigma_abs=11.7856 [barn] sigma_inc=13.6704 [barn] reflections=Na2Ca3Al2F14.laz
---------------------------------------------------------------------
global_process_list.num_elements: 4
name of process [0]: Al_inc
component index [0]: 1
name of process [1]: Al_pow
component index [1]: 2
name of process [2]: Sample_inc
component index [2]: 4
name of process [3]: Sample_pow
component index [3]: 5
---------------------------------------------------------------------
global_material_list.num_elements: 2
name of material [0]: Al
component index [0]: 3
my_absoprtion [0]: 0.347892
number of processes [0]: 2
name of material [1]: Sample
component index [1]: 6
my_absoprtion [1]: 0.273042
number of processes [1]: 2
---------------------------------------------------------------------
global_geometry_list.num_elements: 2
name of geometry [0]: sample_geometry
component index [0]: 8
Volume.name [0]: sample_geometry
Volume.p_physics.is_vacuum [0]: 0
Volume.p_physics.my_absorption [0]: 0.273042
Volume.p_physics.number of processes [0]: 2
Volume.geometry.shape [0]: cylinder
Volume.geometry.center.x [0]: 0.000000
Volume.geometry.center.y [0]: 0.000000
Volume.geometry.center.z [0]: 1.000000
Volume.geometry.rotation_matrix[0] [0]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [0]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [0]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [0]: 0.007500
Volume.geometry.geometry_parameters.height [0]: 0.030000
Volume.geometry.focus_data_array.elements[0].Aim [0]: [0.000000 0.000000 1.000000]
name of geometry [1]: sample_container
component index [1]: 9
Volume.name [1]: sample_container
Volume.p_physics.is_vacuum [1]: 0
Volume.p_physics.my_absorption [1]: 0.347892
Volume.p_physics.number of processes [1]: 2
Volume.geometry.shape [1]: cylinder
Volume.geometry.center.x [1]: 0.000000
Volume.geometry.center.y [1]: 0.000000
Volume.geometry.center.z [1]: 1.000000
Volume.geometry.rotation_matrix[0] [1]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [1]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [1]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [1]: 0.009000
Volume.geometry.geometry_parameters.height [1]: 0.033000
Volume.geometry.focus_data_array.elements[0].Aim [1]: [0.000000 0.000000 1.000000]
name of geometry [2]: sample_container_lid
component index [2]: 10
Volume.name [2]: sample_container_lid
Volume.p_physics.is_vacuum [2]: 0
Volume.p_physics.my_absorption [2]: 0.347892
Volume.p_physics.number of processes [2]: 2
Volume.geometry.shape [2]: cylinder
Volume.geometry.center.x [2]: 0.000000
Volume.geometry.center.y [2]: 0.015500
Volume.geometry.center.z [2]: 1.000000
Volume.geometry.rotation_matrix[0] [2]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [2]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [2]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [2]: 0.013000
Volume.geometry.geometry_parameters.height [2]: 0.004000
Volume.geometry.focus_data_array.elements[0].Aim [2]: [0.000000 0.000000 1.000000]
name of geometry [3]: cryostat_wall
component index [3]: 11
Volume.name [3]: cryostat_wall
Volume.p_physics.is_vacuum [3]: 0
Volume.p_physics.my_absorption [3]: 0.347892
Volume.p_physics.number of processes [3]: 2
Volume.geometry.shape [3]: cylinder
Volume.geometry.center.x [3]: 0.000000
Volume.geometry.center.y [3]: 0.000000
Volume.geometry.center.z [3]: 1.000000
Volume.geometry.rotation_matrix[0] [3]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [3]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [3]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [3]: 0.030000
Volume.geometry.geometry_parameters.height [3]: 0.120000
Volume.geometry.focus_data_array.elements[0].Aim [3]: [0.000000 0.000000 1.000000]
name of geometry [4]: cryostat_wall_vacuum
component index [4]: 12
Volume.name [4]: cryostat_wall_vacuum
Volume.p_physics.is_vacuum [4]: 1
Volume.p_physics.my_absorption [4]: 0.000000
Volume.p_physics.number of processes [4]: 0
Volume.geometry.shape [4]: cylinder
Volume.geometry.center.x [4]: 0.000000
Volume.geometry.center.y [4]: 0.000000
Volume.geometry.center.z [4]: 1.000000
Volume.geometry.rotation_matrix[0] [4]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [4]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [4]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [4]: 0.028000
Volume.geometry.geometry_parameters.height [4]: 0.112000
Volume.geometry.focus_data_array.elements[0].Aim [4]: [0.000000 0.000000 1.000000]
name of geometry [5]: outer_cryostat_wall
component index [5]: 13
Volume.name [5]: outer_cryostat_wall
Volume.p_physics.is_vacuum [5]: 0
Volume.p_physics.my_absorption [5]: 0.347892
Volume.p_physics.number of processes [5]: 2
Volume.geometry.shape [5]: cylinder
Volume.geometry.center.x [5]: 0.000000
Volume.geometry.center.y [5]: 0.000000
Volume.geometry.center.z [5]: 1.000000
Volume.geometry.rotation_matrix[0] [5]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [5]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [5]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [5]: 0.100000
Volume.geometry.geometry_parameters.height [5]: 0.150000
Volume.geometry.focus_data_array.elements[0].Aim [5]: [0.000000 0.000000 1.000000]
name of geometry [6]: outer_cryostat_wall_vacuum
component index [6]: 14
Volume.name [6]: outer_cryostat_wall_vacuum
Volume.p_physics.is_vacuum [6]: 1
Volume.p_physics.my_absorption [6]: 0.000000
Volume.p_physics.number of processes [6]: 0
Volume.geometry.shape [6]: cylinder
Volume.geometry.center.x [6]: 0.000000
Volume.geometry.center.y [6]: 0.000000
Volume.geometry.center.z [6]: 1.000000
Volume.geometry.rotation_matrix[0] [6]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [6]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [6]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [6]: 0.097000
Volume.geometry.geometry_parameters.height [6]: 0.140000
Volume.geometry.focus_data_array.elements[0].Aim [6]: [0.000000 0.000000 1.000000]
---------------------------------------------------------------------
number_of_volumes = 8
number_of_masks = 0
number_of_masked_volumes = 0
---- Overview of the lists generated for each volume ----
List overview for surrounding vacuum
LIST: Children for Volume 0 = [1,2,3,4,5,6,7]
LIST: Direct_children for Volume 0 = [6]
LIST: Intersect_check_list for Volume 0 = [6]
LIST: Mask_intersect_list for Volume 0 = []
LIST: Destinations_list for Volume 0 = []
LIST: Reduced_destinations_list for Volume 0 = []
LIST: Next_volume_list for Volume 0 = [6]
LIST: mask_list for Volume 0 = []
LIST: masked_by_list for Volume 0 = []
LIST: masked_by_mask_index_list for Volume 0 = []
mask_mode for Volume 0 = 0
List overview for sample_geometry with cylinder shape made of Sample
LIST: Children for Volume 1 = []
LIST: Direct_children for Volume 1 = []
LIST: Intersect_check_list for Volume 1 = []
LIST: Mask_intersect_list for Volume 1 = []
LIST: Destinations_list for Volume 1 = [2,3]
LIST: Reduced_destinations_list for Volume 1 = [2,3]
LIST: Next_volume_list for Volume 1 = [2,3]
Is_vacuum for Volume 1 = 0
is_mask_volume for Volume 1 = 0
is_masked_volume for Volume 1 = 0
is_exit_volume for Volume 1 = 0
LIST: mask_list for Volume 1 = []
LIST: masked_by_list for Volume 1 = []
LIST: masked_by_mask_index_list for Volume 1 = []
mask_mode for Volume 1 = 0
List overview for sample_container with cylinder shape made of Al
LIST: Children for Volume 2 = [1]
LIST: Direct_children for Volume 2 = [1]
LIST: Intersect_check_list for Volume 2 = [1]
LIST: Mask_intersect_list for Volume 2 = []
LIST: Destinations_list for Volume 2 = [3,5]
LIST: Reduced_destinations_list for Volume 2 = [5]
LIST: Next_volume_list for Volume 2 = [3,5,1]
Is_vacuum for Volume 2 = 0
is_mask_volume for Volume 2 = 0
is_masked_volume for Volume 2 = 0
is_exit_volume for Volume 2 = 0
LIST: mask_list for Volume 2 = []
LIST: masked_by_list for Volume 2 = []
LIST: masked_by_mask_index_list for Volume 2 = []
mask_mode for Volume 2 = 0
List overview for sample_container_lid with cylinder shape made of Al
LIST: Children for Volume 3 = []
LIST: Direct_children for Volume 3 = []
LIST: Intersect_check_list for Volume 3 = [2]
LIST: Mask_intersect_list for Volume 3 = []
LIST: Destinations_list for Volume 3 = [5]
LIST: Reduced_destinations_list for Volume 3 = [5]
LIST: Next_volume_list for Volume 3 = [5,2]
Is_vacuum for Volume 3 = 0
is_mask_volume for Volume 3 = 0
is_masked_volume for Volume 3 = 0
is_exit_volume for Volume 3 = 0
LIST: mask_list for Volume 3 = []
LIST: masked_by_list for Volume 3 = []
LIST: masked_by_mask_index_list for Volume 3 = []
mask_mode for Volume 3 = 0
List overview for cryostat_wall with cylinder shape made of Al
LIST: Children for Volume 4 = [1,2,3,5]
LIST: Direct_children for Volume 4 = [5]
LIST: Intersect_check_list for Volume 4 = [5]
LIST: Mask_intersect_list for Volume 4 = []
LIST: Destinations_list for Volume 4 = [7]
LIST: Reduced_destinations_list for Volume 4 = [7]
LIST: Next_volume_list for Volume 4 = [7,5]
Is_vacuum for Volume 4 = 0
is_mask_volume for Volume 4 = 0
is_masked_volume for Volume 4 = 0
is_exit_volume for Volume 4 = 0
LIST: mask_list for Volume 4 = []
LIST: masked_by_list for Volume 4 = []
LIST: masked_by_mask_index_list for Volume 4 = []
mask_mode for Volume 4 = 0
List overview for cryostat_wall_vacuum with cylinder shape made of Vacuum
LIST: Children for Volume 5 = [1,2,3]
LIST: Direct_children for Volume 5 = [2,3]
LIST: Intersect_check_list for Volume 5 = [2,3]
LIST: Mask_intersect_list for Volume 5 = []
LIST: Destinations_list for Volume 5 = [4]
LIST: Reduced_destinations_list for Volume 5 = [4]
LIST: Next_volume_list for Volume 5 = [4,2,3]
Is_vacuum for Volume 5 = 1
is_mask_volume for Volume 5 = 0
is_masked_volume for Volume 5 = 0
is_exit_volume for Volume 5 = 0
LIST: mask_list for Volume 5 = []
LIST: masked_by_list for Volume 5 = []
LIST: masked_by_mask_index_list for Volume 5 = []
mask_mode for Volume 5 = 0
List overview for outer_cryostat_wall with cylinder shape made of Al
LIST: Children for Volume 6 = [1,2,3,4,5,7]
LIST: Direct_children for Volume 6 = [7]
LIST: Intersect_check_list for Volume 6 = [7]
LIST: Mask_intersect_list for Volume 6 = []
LIST: Destinations_list for Volume 6 = [0]
LIST: Reduced_destinations_list for Volume 6 = []
LIST: Next_volume_list for Volume 6 = [0,7]
Is_vacuum for Volume 6 = 0
is_mask_volume for Volume 6 = 0
is_masked_volume for Volume 6 = 0
is_exit_volume for Volume 6 = 0
LIST: mask_list for Volume 6 = []
LIST: masked_by_list for Volume 6 = []
LIST: masked_by_mask_index_list for Volume 6 = []
mask_mode for Volume 6 = 0
List overview for outer_cryostat_wall_vacuum with cylinder shape made of Vacuum
LIST: Children for Volume 7 = [1,2,3,4,5]
LIST: Direct_children for Volume 7 = [4]
LIST: Intersect_check_list for Volume 7 = [4]
LIST: Mask_intersect_list for Volume 7 = []
LIST: Destinations_list for Volume 7 = [6]
LIST: Reduced_destinations_list for Volume 7 = [6]
LIST: Next_volume_list for Volume 7 = [6,4]
Is_vacuum for Volume 7 = 1
is_mask_volume for Volume 7 = 0
is_masked_volume for Volume 7 = 0
is_exit_volume for Volume 7 = 0
LIST: mask_list for Volume 7 = []
LIST: masked_by_list for Volume 7 = []
LIST: masked_by_mask_index_list for Volume 7 = []
mask_mode for Volume 7 = 0
Union_master component master initialized sucessfully
Detector: logger_space_zx_I=731.303 logger_space_zx_ERR=5.1552 logger_space_zx_N=39901 "logger_zx.dat"
Detector: logger_space_zy_I=2798.98 logger_space_zy_ERR=16.4045 logger_space_zy_N=140369 "logger_zy.dat"
INFO: Placing instr file copy python_tutorial.instr in dataset /Users/madsbertelsen/PaNOSC/McStasScript/github/McStasScript/docs/source/tutorial/data_folder/union_loggers_6
loading system configuration
ms.make_sub_plot(data, log=True, orders_of_mag=3)
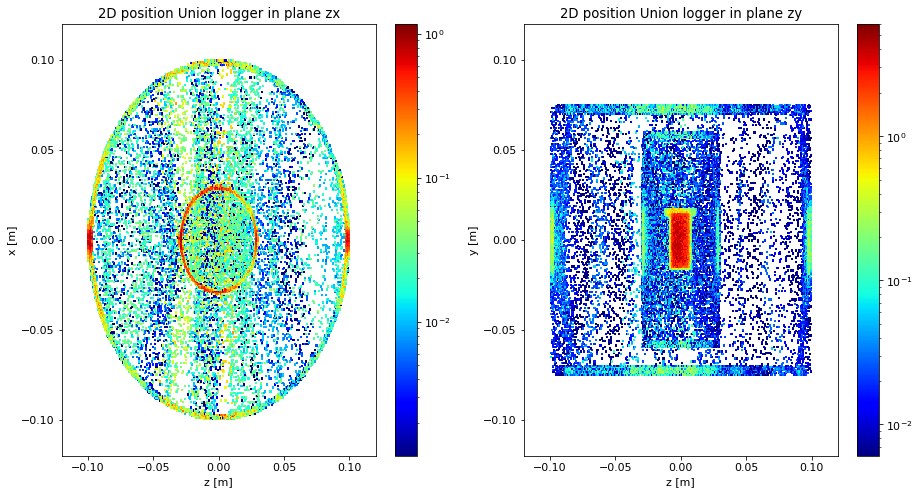
Demonstration of additional logger components¶
Here we add a few more loggers to showcase what kind of information that can be displayed.
1D logger that logs scattered intensity as function of time
2D abs_logger that logs absorption projected onto the scattering plane
2DQ logger that logs scattering vector projected onto the scattering plane
2D kf logger that logs final wavevector projected onto the scattering plane
logger_1D = instrument.add_component("logger_1D", "Union_logger_1D", before="master")
logger_1D.variable = '"time"'
logger_1D.min_value = 0.0006
logger_1D.max_value = 0.0012
logger_1D.n1 = 300
logger_1D.filename = '"logger_1D_time.dat"'
abs_logger_zx = instrument.add_component("abs_logger_space_zx", "Union_abs_logger_2D_space",
before="master")
abs_logger_zx.set_AT([0,0,0], RELATIVE=sample_geometry)
abs_logger_zx.D_direction_1 = '"z"'
abs_logger_zx.D1_min = -0.12
abs_logger_zx.D1_max = 0.12
abs_logger_zx.n1 = 300
abs_logger_zx.D_direction_2 = '"x"'
abs_logger_zx.D2_min = -0.12
abs_logger_zx.D2_max = 0.12
abs_logger_zx.n2 = 300
abs_logger_zx.filename = '"abs_logger_zx.dat"'
logger_2DQ = instrument.add_component("logger_2DQ", "Union_logger_2DQ", before="master")
logger_2DQ.Q_direction_1 = '"z"'
logger_2DQ.Q1_min = -5.0
logger_2DQ.Q1_max = 5.0
logger_2DQ.n1 = 200
logger_2DQ.Q_direction_2 = '"x"'
logger_2DQ.Q2_min = -5.0
logger_2DQ.Q2_max = 5.0
logger_2DQ.n2 = 200
logger_2DQ.filename = '"logger_2DQ.dat"'
logger_2D_kf = instrument.add_component("logger_2D_kf", "Union_logger_2D_kf",
before="master")
logger_2D_kf.Q_direction_1 = '"z"'
logger_2D_kf.Q1_min = -2.5
logger_2D_kf.Q1_max = 2.5
logger_2D_kf.n1 = 200
logger_2D_kf.Q_direction_2 = '"x"'
logger_2D_kf.Q2_min = -2.5
logger_2D_kf.Q2_max = 2.5
logger_2D_kf.n2 = 200
logger_2D_kf.filename = '"logger_2D_kf.dat"'
Runnig the simulation¶
We now rerun the simulation with the new loggers. If mpi is installed, one can add mpi=N where N is the number of cores available to speed up the simulation.
data = instrument.backengine()
INFO: Using directory: "/Users/madsbertelsen/PaNOSC/McStasScript/github/McStasScript/docs/source/tutorial/data_folder/union_loggers_7"
INFO: Regenerating c-file: python_tutorial.c
CFLAGS= -I@MCCODE_LIB@/share/ -I@MCCODE_LIB@/share/
INFO: Recompiling: ./python_tutorial.out
mccode-r.c:2837:3: warning: expression result unused [-Wunused-value]
*t0;
^~~
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1604:105: warning: incompatible function pointer types passing 'int (const struct saved_history_struct *, const struct saved_history_struct *)' to parameter of type 'int (* _Nonnull)(const void *, const void *)' [-Wincompatible-function-pointer-types]
qsort(total_history.saved_histories,total_history.used_elements,sizeof (struct saved_history_struct), Sample_compare_history_intensities);
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/stdlib.h:161:22: note: passing argument to parameter '__compar' here
int (* _Nonnull __compar)(const void *, const void *));
^
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1613:20: warning: incompatible pointer types passing 'struct saved_history_struct *' to parameter of type 'struct dynamic_history_list *' [-Wincompatible-pointer-types]
printf_history(&total_history.saved_histories[history_iterate]);
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1434:50: note: passing argument to parameter 'history' here
void printf_history(struct dynamic_history_list *history) {
^
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:2030:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:839:1: warning: non-void function does not return a value in all control paths [-Wreturn-type]
};
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:883:1: warning: non-void function does not return a value [-Wreturn-type]
};
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3274:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3274:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3276:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3276:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3278:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3278:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3280:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3280:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:20436:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zx_filename
^~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:20436:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zx_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:20679:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zy_filename
^~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:20679:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zy_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_1D.comp:479:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_1D.Filename,filename);
^~~~~~~~
./python_tutorial.c:20918:18: note: expanded from macro 'filename'
#define filename mcclogger_1D_filename
^~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_1D.comp:479:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_1D.Filename,filename);
^
"%s",
./python_tutorial.c:20918:18: note: expanded from macro 'filename'
#define filename mcclogger_1D_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_abs_logger_2D_space.comp:543:49: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_abs_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:21109:18: note: expanded from macro 'filename'
#define filename mccabs_logger_space_zx_filename
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_abs_logger_2D_space.comp:543:49: note: treat the string as an argument to avoid this
sprintf(this_abs_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:21109:18: note: expanded from macro 'filename'
#define filename mccabs_logger_space_zx_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2DQ.comp:535:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:21333:18: note: expanded from macro 'filename'
#define filename mcclogger_2DQ_filename
^~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2DQ.comp:535:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:21333:18: note: expanded from macro 'filename'
#define filename mcclogger_2DQ_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_kf.comp:536:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:21561:18: note: expanded from macro 'filename'
#define filename mcclogger_2D_kf_filename
^~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_kf.comp:536:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:21561:18: note: expanded from macro 'filename'
#define filename mcclogger_2D_kf_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:788:15: warning: left operand of comma operator has no effect [-Wunused-value]
if (volume_index_main,Volumes[volume_index_main]->geometry.is_mask_volume == 0 ||
^~~~~~~~~~~~~~~~~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:788:90: warning: left operand of comma operator has no effect [-Wunused-value]
if (volume_index_main,Volumes[volume_index_main]->geometry.is_mask_volume == 0 ||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~^~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:789:92: warning: left operand of comma operator has no effect [-Wunused-value]
volume_index_main,Volumes[volume_index_main]->geometry.is_masked_volume == 0 ||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~^~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
18 warnings generated.
INFO: ===
Opening input file '/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//data/Al.laz' (Table_Read_Offset)
Table from file 'Al.laz' (block 1) is 26 x 18 (x=1:8), constant step. interpolation: linear
'# TITLE *Aluminum-Al-[FM3-M] Miller, H.P.jr.;DuMond, J.W.M.[1942] at 298 K; ...'
PowderN: Al_pow: Reading 26 rows from Al.laz
PowderN: Al_pow: Read 26 reflections from file 'Al.laz'
PowderN: Al_pow: Vc=66.4 [Angs] sigma_abs=0.924 [barn] sigma_inc=0.0328 [barn] reflections=Al.laz
Opening input file '/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//data/Na2Ca3Al2F14.laz' (Table_Read_Offset)
Table from file 'Na2Ca3Al2F14.laz' (block 1) is 841 x 18 (x=1:20), constant step. interpolation: linear
'# TITLE *-Na2Ca3Al2F14-[I213] Courbion, G.;Ferey, G.[1988] Standard NAC cal ...'
PowderN: Sample_pow: Reading 841 rows from Na2Ca3Al2F14.laz
PowderN: Sample_pow: Read 841 reflections from file 'Na2Ca3Al2F14.laz'
PowderN: Sample_pow: Vc=1079.1 [Angs] sigma_abs=11.7856 [barn] sigma_inc=13.6704 [barn] reflections=Na2Ca3Al2F14.laz
---------------------------------------------------------------------
global_process_list.num_elements: 4
name of process [0]: Al_inc
component index [0]: 1
name of process [1]: Al_pow
component index [1]: 2
name of process [2]: Sample_inc
component index [2]: 4
name of process [3]: Sample_pow
component index [3]: 5
---------------------------------------------------------------------
global_material_list.num_elements: 2
name of material [0]: Al
component index [0]: 3
my_absoprtion [0]: 0.347892
number of processes [0]: 2
name of material [1]: Sample
component index [1]: 6
my_absoprtion [1]: 0.273042
number of processes [1]: 2
---------------------------------------------------------------------
global_geometry_list.num_elements: 2
name of geometry [0]: sample_geometry
component index [0]: 8
Volume.name [0]: sample_geometry
Volume.p_physics.is_vacuum [0]: 0
Volume.p_physics.my_absorption [0]: 0.273042
Volume.p_physics.number of processes [0]: 2
Volume.geometry.shape [0]: cylinder
Volume.geometry.center.x [0]: 0.000000
Volume.geometry.center.y [0]: 0.000000
Volume.geometry.center.z [0]: 1.000000
Volume.geometry.rotation_matrix[0] [0]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [0]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [0]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [0]: 0.007500
Volume.geometry.geometry_parameters.height [0]: 0.030000
Volume.geometry.focus_data_array.elements[0].Aim [0]: [0.000000 0.000000 1.000000]
name of geometry [1]: sample_container
component index [1]: 9
Volume.name [1]: sample_container
Volume.p_physics.is_vacuum [1]: 0
Volume.p_physics.my_absorption [1]: 0.347892
Volume.p_physics.number of processes [1]: 2
Volume.geometry.shape [1]: cylinder
Volume.geometry.center.x [1]: 0.000000
Volume.geometry.center.y [1]: 0.000000
Volume.geometry.center.z [1]: 1.000000
Volume.geometry.rotation_matrix[0] [1]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [1]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [1]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [1]: 0.009000
Volume.geometry.geometry_parameters.height [1]: 0.033000
Volume.geometry.focus_data_array.elements[0].Aim [1]: [0.000000 0.000000 1.000000]
name of geometry [2]: sample_container_lid
component index [2]: 10
Volume.name [2]: sample_container_lid
Volume.p_physics.is_vacuum [2]: 0
Volume.p_physics.my_absorption [2]: 0.347892
Volume.p_physics.number of processes [2]: 2
Volume.geometry.shape [2]: cylinder
Volume.geometry.center.x [2]: 0.000000
Volume.geometry.center.y [2]: 0.015500
Volume.geometry.center.z [2]: 1.000000
Volume.geometry.rotation_matrix[0] [2]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [2]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [2]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [2]: 0.013000
Volume.geometry.geometry_parameters.height [2]: 0.004000
Volume.geometry.focus_data_array.elements[0].Aim [2]: [0.000000 0.000000 1.000000]
name of geometry [3]: cryostat_wall
component index [3]: 11
Volume.name [3]: cryostat_wall
Volume.p_physics.is_vacuum [3]: 0
Volume.p_physics.my_absorption [3]: 0.347892
Volume.p_physics.number of processes [3]: 2
Volume.geometry.shape [3]: cylinder
Volume.geometry.center.x [3]: 0.000000
Volume.geometry.center.y [3]: 0.000000
Volume.geometry.center.z [3]: 1.000000
Volume.geometry.rotation_matrix[0] [3]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [3]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [3]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [3]: 0.030000
Volume.geometry.geometry_parameters.height [3]: 0.120000
Volume.geometry.focus_data_array.elements[0].Aim [3]: [0.000000 0.000000 1.000000]
name of geometry [4]: cryostat_wall_vacuum
component index [4]: 12
Volume.name [4]: cryostat_wall_vacuum
Volume.p_physics.is_vacuum [4]: 1
Volume.p_physics.my_absorption [4]: 0.000000
Volume.p_physics.number of processes [4]: 0
Volume.geometry.shape [4]: cylinder
Volume.geometry.center.x [4]: 0.000000
Volume.geometry.center.y [4]: 0.000000
Volume.geometry.center.z [4]: 1.000000
Volume.geometry.rotation_matrix[0] [4]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [4]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [4]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [4]: 0.028000
Volume.geometry.geometry_parameters.height [4]: 0.112000
Volume.geometry.focus_data_array.elements[0].Aim [4]: [0.000000 0.000000 1.000000]
name of geometry [5]: outer_cryostat_wall
component index [5]: 13
Volume.name [5]: outer_cryostat_wall
Volume.p_physics.is_vacuum [5]: 0
Volume.p_physics.my_absorption [5]: 0.347892
Volume.p_physics.number of processes [5]: 2
Volume.geometry.shape [5]: cylinder
Volume.geometry.center.x [5]: 0.000000
Volume.geometry.center.y [5]: 0.000000
Volume.geometry.center.z [5]: 1.000000
Volume.geometry.rotation_matrix[0] [5]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [5]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [5]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [5]: 0.100000
Volume.geometry.geometry_parameters.height [5]: 0.150000
Volume.geometry.focus_data_array.elements[0].Aim [5]: [0.000000 0.000000 1.000000]
name of geometry [6]: outer_cryostat_wall_vacuum
component index [6]: 14
Volume.name [6]: outer_cryostat_wall_vacuum
Volume.p_physics.is_vacuum [6]: 1
Volume.p_physics.my_absorption [6]: 0.000000
Volume.p_physics.number of processes [6]: 0
Volume.geometry.shape [6]: cylinder
Volume.geometry.center.x [6]: 0.000000
Volume.geometry.center.y [6]: 0.000000
Volume.geometry.center.z [6]: 1.000000
Volume.geometry.rotation_matrix[0] [6]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [6]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [6]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [6]: 0.097000
Volume.geometry.geometry_parameters.height [6]: 0.140000
Volume.geometry.focus_data_array.elements[0].Aim [6]: [0.000000 0.000000 1.000000]
---------------------------------------------------------------------
number_of_volumes = 8
number_of_masks = 0
number_of_masked_volumes = 0
---- Overview of the lists generated for each volume ----
List overview for surrounding vacuum
LIST: Children for Volume 0 = [1,2,3,4,5,6,7]
LIST: Direct_children for Volume 0 = [6]
LIST: Intersect_check_list for Volume 0 = [6]
LIST: Mask_intersect_list for Volume 0 = []
LIST: Destinations_list for Volume 0 = []
LIST: Reduced_destinations_list for Volume 0 = []
LIST: Next_volume_list for Volume 0 = [6]
LIST: mask_list for Volume 0 = []
LIST: masked_by_list for Volume 0 = []
LIST: masked_by_mask_index_list for Volume 0 = []
mask_mode for Volume 0 = 0
List overview for sample_geometry with cylinder shape made of Sample
LIST: Children for Volume 1 = []
LIST: Direct_children for Volume 1 = []
LIST: Intersect_check_list for Volume 1 = []
LIST: Mask_intersect_list for Volume 1 = []
LIST: Destinations_list for Volume 1 = [2,3]
LIST: Reduced_destinations_list for Volume 1 = [2,3]
LIST: Next_volume_list for Volume 1 = [2,3]
Is_vacuum for Volume 1 = 0
is_mask_volume for Volume 1 = 0
is_masked_volume for Volume 1 = 0
is_exit_volume for Volume 1 = 0
LIST: mask_list for Volume 1 = []
LIST: masked_by_list for Volume 1 = []
LIST: masked_by_mask_index_list for Volume 1 = []
mask_mode for Volume 1 = 0
List overview for sample_container with cylinder shape made of Al
LIST: Children for Volume 2 = [1]
LIST: Direct_children for Volume 2 = [1]
LIST: Intersect_check_list for Volume 2 = [1]
LIST: Mask_intersect_list for Volume 2 = []
LIST: Destinations_list for Volume 2 = [3,5]
LIST: Reduced_destinations_list for Volume 2 = [5]
LIST: Next_volume_list for Volume 2 = [3,5,1]
Is_vacuum for Volume 2 = 0
is_mask_volume for Volume 2 = 0
is_masked_volume for Volume 2 = 0
is_exit_volume for Volume 2 = 0
LIST: mask_list for Volume 2 = []
LIST: masked_by_list for Volume 2 = []
LIST: masked_by_mask_index_list for Volume 2 = []
mask_mode for Volume 2 = 0
List overview for sample_container_lid with cylinder shape made of Al
LIST: Children for Volume 3 = []
LIST: Direct_children for Volume 3 = []
LIST: Intersect_check_list for Volume 3 = [2]
LIST: Mask_intersect_list for Volume 3 = []
LIST: Destinations_list for Volume 3 = [5]
LIST: Reduced_destinations_list for Volume 3 = [5]
LIST: Next_volume_list for Volume 3 = [5,2]
Is_vacuum for Volume 3 = 0
is_mask_volume for Volume 3 = 0
is_masked_volume for Volume 3 = 0
is_exit_volume for Volume 3 = 0
LIST: mask_list for Volume 3 = []
LIST: masked_by_list for Volume 3 = []
LIST: masked_by_mask_index_list for Volume 3 = []
mask_mode for Volume 3 = 0
List overview for cryostat_wall with cylinder shape made of Al
LIST: Children for Volume 4 = [1,2,3,5]
LIST: Direct_children for Volume 4 = [5]
LIST: Intersect_check_list for Volume 4 = [5]
LIST: Mask_intersect_list for Volume 4 = []
LIST: Destinations_list for Volume 4 = [7]
LIST: Reduced_destinations_list for Volume 4 = [7]
LIST: Next_volume_list for Volume 4 = [7,5]
Is_vacuum for Volume 4 = 0
is_mask_volume for Volume 4 = 0
is_masked_volume for Volume 4 = 0
is_exit_volume for Volume 4 = 0
LIST: mask_list for Volume 4 = []
LIST: masked_by_list for Volume 4 = []
LIST: masked_by_mask_index_list for Volume 4 = []
mask_mode for Volume 4 = 0
List overview for cryostat_wall_vacuum with cylinder shape made of Vacuum
LIST: Children for Volume 5 = [1,2,3]
LIST: Direct_children for Volume 5 = [2,3]
LIST: Intersect_check_list for Volume 5 = [2,3]
LIST: Mask_intersect_list for Volume 5 = []
LIST: Destinations_list for Volume 5 = [4]
LIST: Reduced_destinations_list for Volume 5 = [4]
LIST: Next_volume_list for Volume 5 = [4,2,3]
Is_vacuum for Volume 5 = 1
is_mask_volume for Volume 5 = 0
is_masked_volume for Volume 5 = 0
is_exit_volume for Volume 5 = 0
LIST: mask_list for Volume 5 = []
LIST: masked_by_list for Volume 5 = []
LIST: masked_by_mask_index_list for Volume 5 = []
mask_mode for Volume 5 = 0
List overview for outer_cryostat_wall with cylinder shape made of Al
LIST: Children for Volume 6 = [1,2,3,4,5,7]
LIST: Direct_children for Volume 6 = [7]
LIST: Intersect_check_list for Volume 6 = [7]
LIST: Mask_intersect_list for Volume 6 = []
LIST: Destinations_list for Volume 6 = [0]
LIST: Reduced_destinations_list for Volume 6 = []
LIST: Next_volume_list for Volume 6 = [0,7]
Is_vacuum for Volume 6 = 0
is_mask_volume for Volume 6 = 0
is_masked_volume for Volume 6 = 0
is_exit_volume for Volume 6 = 0
LIST: mask_list for Volume 6 = []
LIST: masked_by_list for Volume 6 = []
LIST: masked_by_mask_index_list for Volume 6 = []
mask_mode for Volume 6 = 0
List overview for outer_cryostat_wall_vacuum with cylinder shape made of Vacuum
LIST: Children for Volume 7 = [1,2,3,4,5]
LIST: Direct_children for Volume 7 = [4]
LIST: Intersect_check_list for Volume 7 = [4]
LIST: Mask_intersect_list for Volume 7 = []
LIST: Destinations_list for Volume 7 = [6]
LIST: Reduced_destinations_list for Volume 7 = [6]
LIST: Next_volume_list for Volume 7 = [6,4]
Is_vacuum for Volume 7 = 1
is_mask_volume for Volume 7 = 0
is_masked_volume for Volume 7 = 0
is_exit_volume for Volume 7 = 0
LIST: mask_list for Volume 7 = []
LIST: masked_by_list for Volume 7 = []
LIST: masked_by_mask_index_list for Volume 7 = []
mask_mode for Volume 7 = 0
Union_master component master initialized sucessfully
Detector: logger_space_zx_I=728.516 logger_space_zx_ERR=5.10581 logger_space_zx_N=39950 "logger_zx.dat"
Detector: logger_space_zy_I=2799.25 logger_space_zy_ERR=16.164 logger_space_zy_N=140672 "logger_zy.dat"
Detector: logger_1D_I=20543.6 logger_1D_ERR=33.4588 logger_1D_N=1.01222e+06 "logger_1D_time.dat"
Detector: abs_logger_space_zx_I=959.677 abs_logger_space_zx_ERR=1.34296 abs_logger_space_zx_N=1.01222e+06 "abs_logger_zx.dat"
Detector: logger_2DQ_I=20543.6 logger_2DQ_ERR=33.4588 logger_2DQ_N=1.01222e+06 "logger_2DQ.dat"
Detector: logger_2D_kf_I=20543.6 logger_2D_kf_ERR=33.4588 logger_2D_kf_N=1.01222e+06 "logger_2D_kf.dat"
INFO: Placing instr file copy python_tutorial.instr in dataset /Users/madsbertelsen/PaNOSC/McStasScript/github/McStasScript/docs/source/tutorial/data_folder/union_loggers_7
loading system configuration
ms.name_plot_options("logger_space_zx", data, log=True, orders_of_mag=3)
ms.name_plot_options("logger_space_zy", data, log=True, orders_of_mag=3)
ms.name_plot_options("abs_logger_space_zx", data, log=True, orders_of_mag=3)
ms.name_plot_options("logger_1D", data, log=True, orders_of_mag=3)
ms.name_plot_options("logger_2DQ", data, log=True, orders_of_mag=3)
ms.name_plot_options("logger_2D_kf", data, log=True, orders_of_mag=3)
ms.make_sub_plot(data[0:2])
ms.make_sub_plot(data[2:4])
ms.make_sub_plot(data[4:6])
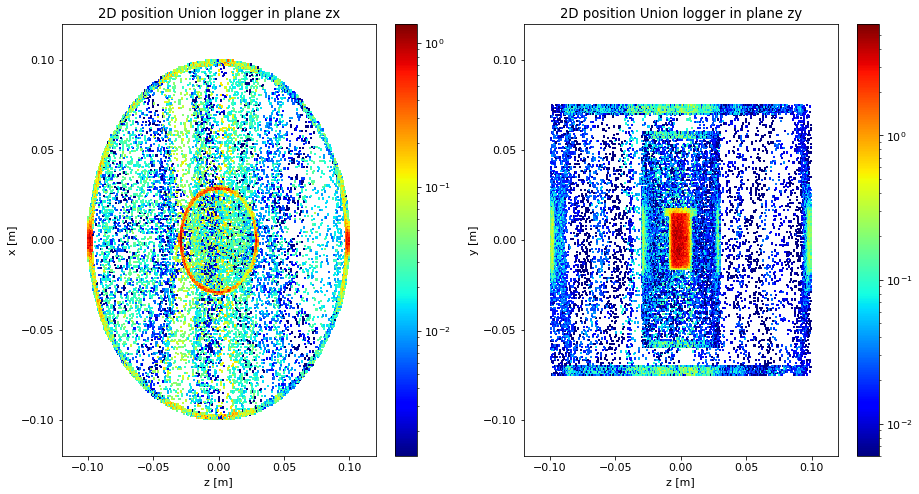
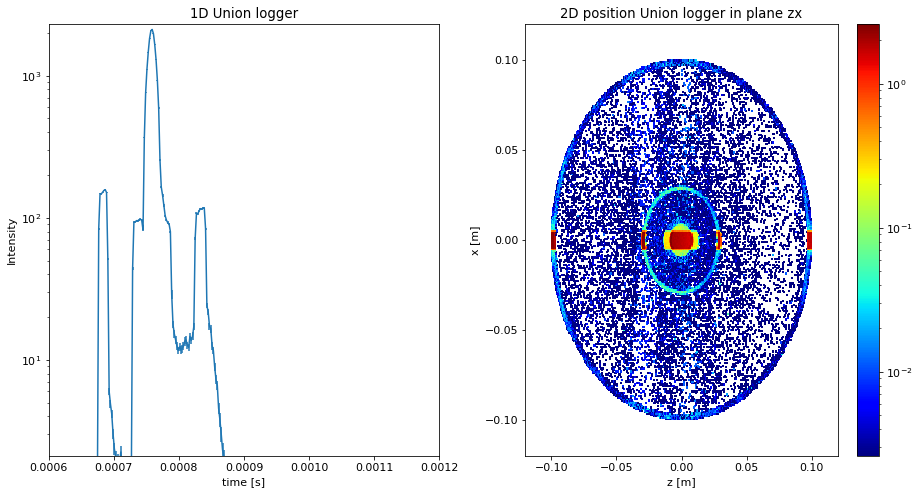
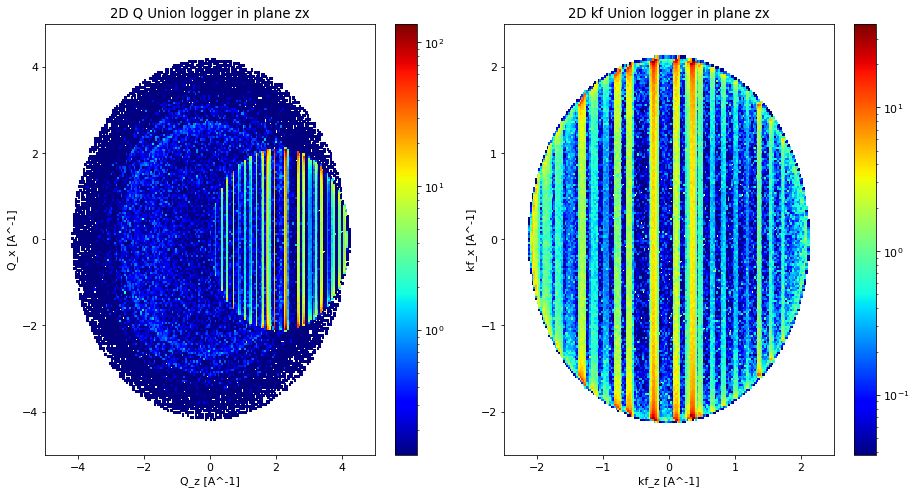
Interpreting the data¶
We see the scattered intensity as a function of time, here the peaks correspond to the direct beam intersecting the sides of the cryostat and sample. The source used release all neutrons at time 0, so it is a perfect pulse.
The absorption monitor shows an image very similar to the scattered intensity, but this could be very different, for example when using materials meant as shielding.
The 2D scattering vector is interesting, it shows a small sphere made of vertical lines, these are powder Bragg peaks. Since the wavevector is almost identical for all incoming neutrons, the first scattering can only access this smaller region of the space. The larger circle is incoherent scattering from second and later scattering events, where the incoming wavevector could be any direction since a scattering already happened.
The 2D final wavevector plot shows mainly the powder Bragg peaks.
Animations and time series¶
Several of the Union loggers sets up more than one McStas monitor, these include:
Union_logger_3D_space
Union_logger_2D_space_time
Union_logger_2D_kf_time
The Union_logger_2D_space_time for example sets up a number of Union_logger_2D_space monitors that are limited to specific time intervals. This can be used to make an animation of the monitor, which we will demonstrate here.
log_2D_st = instrument.add_component("logger_2D_space_time", "Union_logger_2D_space_time",
before="master")
log_2D_st.time_bins = 36
log_2D_st.time_min = 0.0007
log_2D_st.time_max = 0.0011
log_2D_st.set_AT([0,0,0], RELATIVE=sample_geometry)
log_2D_st.D_direction_1 = '"z"'
log_2D_st.D1_min = -0.12
log_2D_st.D1_max = 0.12
log_2D_st.n1 = 300
log_2D_st.D_direction_2 = '"x"'
log_2D_st.D2_min = -0.12
log_2D_st.D2_max = 0.12
log_2D_st.n2 = 300
log_2D_st.filename = '"logger_2D_space_time.dat"'
data = instrument.backengine()
INFO: Using directory: "/Users/madsbertelsen/PaNOSC/McStasScript/github/McStasScript/docs/source/tutorial/data_folder/union_loggers_8"
INFO: Regenerating c-file: python_tutorial.c
CFLAGS= -I@MCCODE_LIB@/share/ -I@MCCODE_LIB@/share/
INFO: Recompiling: ./python_tutorial.out
mccode-r.c:2837:3: warning: expression result unused [-Wunused-value]
*t0;
^~~
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1604:105: warning: incompatible function pointer types passing 'int (const struct saved_history_struct *, const struct saved_history_struct *)' to parameter of type 'int (* _Nonnull)(const void *, const void *)' [-Wincompatible-function-pointer-types]
qsort(total_history.saved_histories,total_history.used_elements,sizeof (struct saved_history_struct), Sample_compare_history_intensities);
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/stdlib.h:161:22: note: passing argument to parameter '__compar' here
int (* _Nonnull __compar)(const void *, const void *));
^
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1613:20: warning: incompatible pointer types passing 'struct saved_history_struct *' to parameter of type 'struct dynamic_history_list *' [-Wincompatible-pointer-types]
printf_history(&total_history.saved_histories[history_iterate]);
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:1434:50: note: passing argument to parameter 'history' here
void printf_history(struct dynamic_history_list *history) {
^
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Incoherent_process.comp:66:
In file included from /Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Union_functions.c:2030:
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:839:1: warning: non-void function does not return a value in all control paths [-Wreturn-type]
};
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:883:1: warning: non-void function does not return a value [-Wreturn-type]
};
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3274:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3274:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3276:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3276:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3278:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3278:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3280:42: warning: if statement has empty body [-Wempty-body]
if (dist_to_corner > sphere_2_radius); { sphere_2_radius = dist_to_corner ; }
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//share/Geometry_functions.c:3280:42: note: put the semicolon on a separate line to silence this warning
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:21010:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zx_filename
^~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:21010:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zx_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:21253:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zy_filename
^~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space.comp:574:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:21253:18: note: expanded from macro 'filename'
#define filename mcclogger_space_zy_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_1D.comp:479:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_1D.Filename,filename);
^~~~~~~~
./python_tutorial.c:21492:18: note: expanded from macro 'filename'
#define filename mcclogger_1D_filename
^~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_1D.comp:479:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_1D.Filename,filename);
^
"%s",
./python_tutorial.c:21492:18: note: expanded from macro 'filename'
#define filename mcclogger_1D_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_abs_logger_2D_space.comp:543:49: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_abs_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:21683:18: note: expanded from macro 'filename'
#define filename mccabs_logger_space_zx_filename
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_abs_logger_2D_space.comp:543:49: note: treat the string as an argument to avoid this
sprintf(this_abs_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:21683:18: note: expanded from macro 'filename'
#define filename mccabs_logger_space_zx_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2DQ.comp:535:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:21907:18: note: expanded from macro 'filename'
#define filename mcclogger_2DQ_filename
^~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2DQ.comp:535:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:21907:18: note: expanded from macro 'filename'
#define filename mcclogger_2DQ_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_kf.comp:536:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_2D.Filename,filename);
^~~~~~~~
./python_tutorial.c:22135:18: note: expanded from macro 'filename'
#define filename mcclogger_2D_kf_filename
^~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_kf.comp:536:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_2D.Filename,filename);
^
"%s",
./python_tutorial.c:22135:18: note: expanded from macro 'filename'
#define filename mcclogger_2D_kf_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space_time.comp:632:45: warning: format string is not a string literal (potentially insecure) [-Wformat-security]
sprintf(this_storage.Detector_3D.Filename,filename);
^~~~~~~~
./python_tutorial.c:22373:18: note: expanded from macro 'filename'
#define filename mcclogger_2D_space_time_filename
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^~~~~~~~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_logger_2D_space_time.comp:632:45: note: treat the string as an argument to avoid this
sprintf(this_storage.Detector_3D.Filename,filename);
^
"%s",
./python_tutorial.c:22373:18: note: expanded from macro 'filename'
#define filename mcclogger_2D_space_time_filename
^
/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/secure/_stdio.h:47:56: note: expanded from macro 'sprintf'
__builtin___sprintf_chk (str, 0, __darwin_obsz(str), __VA_ARGS__)
^
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:788:15: warning: left operand of comma operator has no effect [-Wunused-value]
if (volume_index_main,Volumes[volume_index_main]->geometry.is_mask_volume == 0 ||
^~~~~~~~~~~~~~~~~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:788:90: warning: left operand of comma operator has no effect [-Wunused-value]
if (volume_index_main,Volumes[volume_index_main]->geometry.is_mask_volume == 0 ||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~^~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//contrib/union/Union_master.comp:789:92: warning: left operand of comma operator has no effect [-Wunused-value]
volume_index_main,Volumes[volume_index_main]->geometry.is_masked_volume == 0 ||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~^~
mccode-r.h:219:27: note: expanded from macro 'MPI_MASTER'
#define MPI_MASTER(instr) instr
^~~~~
19 warnings generated.
INFO: ===
Opening input file '/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//data/Al.laz' (Table_Read_Offset)
Table from file 'Al.laz' (block 1) is 26 x 18 (x=1:8), constant step. interpolation: linear
'# TITLE *Aluminum-Al-[FM3-M] Miller, H.P.jr.;DuMond, J.W.M.[1942] at 298 K; ...'
PowderN: Al_pow: Reading 26 rows from Al.laz
PowderN: Al_pow: Read 26 reflections from file 'Al.laz'
PowderN: Al_pow: Vc=66.4 [Angs] sigma_abs=0.924 [barn] sigma_inc=0.0328 [barn] reflections=Al.laz
Opening input file '/Applications/McStas-2.7.1.app/Contents/Resources/mcstas/2.7.1//data/Na2Ca3Al2F14.laz' (Table_Read_Offset)
Table from file 'Na2Ca3Al2F14.laz' (block 1) is 841 x 18 (x=1:20), constant step. interpolation: linear
'# TITLE *-Na2Ca3Al2F14-[I213] Courbion, G.;Ferey, G.[1988] Standard NAC cal ...'
PowderN: Sample_pow: Reading 841 rows from Na2Ca3Al2F14.laz
PowderN: Sample_pow: Read 841 reflections from file 'Na2Ca3Al2F14.laz'
PowderN: Sample_pow: Vc=1079.1 [Angs] sigma_abs=11.7856 [barn] sigma_inc=13.6704 [barn] reflections=Na2Ca3Al2F14.laz
Allocating 3D arrays
---------------------------------------------------------------------
global_process_list.num_elements: 4
name of process [0]: Al_inc
component index [0]: 1
name of process [1]: Al_pow
component index [1]: 2
name of process [2]: Sample_inc
component index [2]: 4
name of process [3]: Sample_pow
component index [3]: 5
---------------------------------------------------------------------
global_material_list.num_elements: 2
name of material [0]: Al
component index [0]: 3
my_absoprtion [0]: 0.347892
number of processes [0]: 2
name of material [1]: Sample
component index [1]: 6
my_absoprtion [1]: 0.273042
number of processes [1]: 2
---------------------------------------------------------------------
global_geometry_list.num_elements: 2
name of geometry [0]: sample_geometry
component index [0]: 8
Volume.name [0]: sample_geometry
Volume.p_physics.is_vacuum [0]: 0
Volume.p_physics.my_absorption [0]: 0.273042
Volume.p_physics.number of processes [0]: 2
Volume.geometry.shape [0]: cylinder
Volume.geometry.center.x [0]: 0.000000
Volume.geometry.center.y [0]: 0.000000
Volume.geometry.center.z [0]: 1.000000
Volume.geometry.rotation_matrix[0] [0]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [0]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [0]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [0]: 0.007500
Volume.geometry.geometry_parameters.height [0]: 0.030000
Volume.geometry.focus_data_array.elements[0].Aim [0]: [0.000000 0.000000 1.000000]
name of geometry [1]: sample_container
component index [1]: 9
Volume.name [1]: sample_container
Volume.p_physics.is_vacuum [1]: 0
Volume.p_physics.my_absorption [1]: 0.347892
Volume.p_physics.number of processes [1]: 2
Volume.geometry.shape [1]: cylinder
Volume.geometry.center.x [1]: 0.000000
Volume.geometry.center.y [1]: 0.000000
Volume.geometry.center.z [1]: 1.000000
Volume.geometry.rotation_matrix[0] [1]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [1]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [1]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [1]: 0.009000
Volume.geometry.geometry_parameters.height [1]: 0.033000
Volume.geometry.focus_data_array.elements[0].Aim [1]: [0.000000 0.000000 1.000000]
name of geometry [2]: sample_container_lid
component index [2]: 10
Volume.name [2]: sample_container_lid
Volume.p_physics.is_vacuum [2]: 0
Volume.p_physics.my_absorption [2]: 0.347892
Volume.p_physics.number of processes [2]: 2
Volume.geometry.shape [2]: cylinder
Volume.geometry.center.x [2]: 0.000000
Volume.geometry.center.y [2]: 0.015500
Volume.geometry.center.z [2]: 1.000000
Volume.geometry.rotation_matrix[0] [2]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [2]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [2]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [2]: 0.013000
Volume.geometry.geometry_parameters.height [2]: 0.004000
Volume.geometry.focus_data_array.elements[0].Aim [2]: [0.000000 0.000000 1.000000]
name of geometry [3]: cryostat_wall
component index [3]: 11
Volume.name [3]: cryostat_wall
Volume.p_physics.is_vacuum [3]: 0
Volume.p_physics.my_absorption [3]: 0.347892
Volume.p_physics.number of processes [3]: 2
Volume.geometry.shape [3]: cylinder
Volume.geometry.center.x [3]: 0.000000
Volume.geometry.center.y [3]: 0.000000
Volume.geometry.center.z [3]: 1.000000
Volume.geometry.rotation_matrix[0] [3]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [3]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [3]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [3]: 0.030000
Volume.geometry.geometry_parameters.height [3]: 0.120000
Volume.geometry.focus_data_array.elements[0].Aim [3]: [0.000000 0.000000 1.000000]
name of geometry [4]: cryostat_wall_vacuum
component index [4]: 12
Volume.name [4]: cryostat_wall_vacuum
Volume.p_physics.is_vacuum [4]: 1
Volume.p_physics.my_absorption [4]: 0.000000
Volume.p_physics.number of processes [4]: 0
Volume.geometry.shape [4]: cylinder
Volume.geometry.center.x [4]: 0.000000
Volume.geometry.center.y [4]: 0.000000
Volume.geometry.center.z [4]: 1.000000
Volume.geometry.rotation_matrix[0] [4]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [4]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [4]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [4]: 0.028000
Volume.geometry.geometry_parameters.height [4]: 0.112000
Volume.geometry.focus_data_array.elements[0].Aim [4]: [0.000000 0.000000 1.000000]
name of geometry [5]: outer_cryostat_wall
component index [5]: 13
Volume.name [5]: outer_cryostat_wall
Volume.p_physics.is_vacuum [5]: 0
Volume.p_physics.my_absorption [5]: 0.347892
Volume.p_physics.number of processes [5]: 2
Volume.geometry.shape [5]: cylinder
Volume.geometry.center.x [5]: 0.000000
Volume.geometry.center.y [5]: 0.000000
Volume.geometry.center.z [5]: 1.000000
Volume.geometry.rotation_matrix[0] [5]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [5]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [5]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [5]: 0.100000
Volume.geometry.geometry_parameters.height [5]: 0.150000
Volume.geometry.focus_data_array.elements[0].Aim [5]: [0.000000 0.000000 1.000000]
name of geometry [6]: outer_cryostat_wall_vacuum
component index [6]: 14
Volume.name [6]: outer_cryostat_wall_vacuum
Volume.p_physics.is_vacuum [6]: 1
Volume.p_physics.my_absorption [6]: 0.000000
Volume.p_physics.number of processes [6]: 0
Volume.geometry.shape [6]: cylinder
Volume.geometry.center.x [6]: 0.000000
Volume.geometry.center.y [6]: 0.000000
Volume.geometry.center.z [6]: 1.000000
Volume.geometry.rotation_matrix[0] [6]: [1.000000 0.000000 0.000000]
Volume.geometry.rotation_matrix[1] [6]: [0.000000 1.000000 0.000000]
Volume.geometry.rotation_matrix[2] [6]: [0.000000 0.000000 1.000000]
Volume.geometry.geometry_parameters.cyl_radius [6]: 0.097000
Volume.geometry.geometry_parameters.height [6]: 0.140000
Volume.geometry.focus_data_array.elements[0].Aim [6]: [0.000000 0.000000 1.000000]
---------------------------------------------------------------------
number_of_volumes = 8
number_of_masks = 0
number_of_masked_volumes = 0
---- Overview of the lists generated for each volume ----
List overview for surrounding vacuum
LIST: Children for Volume 0 = [1,2,3,4,5,6,7]
LIST: Direct_children for Volume 0 = [6]
LIST: Intersect_check_list for Volume 0 = [6]
LIST: Mask_intersect_list for Volume 0 = []
LIST: Destinations_list for Volume 0 = []
LIST: Reduced_destinations_list for Volume 0 = []
LIST: Next_volume_list for Volume 0 = [6]
LIST: mask_list for Volume 0 = []
LIST: masked_by_list for Volume 0 = []
LIST: masked_by_mask_index_list for Volume 0 = []
mask_mode for Volume 0 = 0
List overview for sample_geometry with cylinder shape made of Sample
LIST: Children for Volume 1 = []
LIST: Direct_children for Volume 1 = []
LIST: Intersect_check_list for Volume 1 = []
LIST: Mask_intersect_list for Volume 1 = []
LIST: Destinations_list for Volume 1 = [2,3]
LIST: Reduced_destinations_list for Volume 1 = [2,3]
LIST: Next_volume_list for Volume 1 = [2,3]
Is_vacuum for Volume 1 = 0
is_mask_volume for Volume 1 = 0
is_masked_volume for Volume 1 = 0
is_exit_volume for Volume 1 = 0
LIST: mask_list for Volume 1 = []
LIST: masked_by_list for Volume 1 = []
LIST: masked_by_mask_index_list for Volume 1 = []
mask_mode for Volume 1 = 0
List overview for sample_container with cylinder shape made of Al
LIST: Children for Volume 2 = [1]
LIST: Direct_children for Volume 2 = [1]
LIST: Intersect_check_list for Volume 2 = [1]
LIST: Mask_intersect_list for Volume 2 = []
LIST: Destinations_list for Volume 2 = [3,5]
LIST: Reduced_destinations_list for Volume 2 = [5]
LIST: Next_volume_list for Volume 2 = [3,5,1]
Is_vacuum for Volume 2 = 0
is_mask_volume for Volume 2 = 0
is_masked_volume for Volume 2 = 0
is_exit_volume for Volume 2 = 0
LIST: mask_list for Volume 2 = []
LIST: masked_by_list for Volume 2 = []
LIST: masked_by_mask_index_list for Volume 2 = []
mask_mode for Volume 2 = 0
List overview for sample_container_lid with cylinder shape made of Al
LIST: Children for Volume 3 = []
LIST: Direct_children for Volume 3 = []
LIST: Intersect_check_list for Volume 3 = [2]
LIST: Mask_intersect_list for Volume 3 = []
LIST: Destinations_list for Volume 3 = [5]
LIST: Reduced_destinations_list for Volume 3 = [5]
LIST: Next_volume_list for Volume 3 = [5,2]
Is_vacuum for Volume 3 = 0
is_mask_volume for Volume 3 = 0
is_masked_volume for Volume 3 = 0
is_exit_volume for Volume 3 = 0
LIST: mask_list for Volume 3 = []
LIST: masked_by_list for Volume 3 = []
LIST: masked_by_mask_index_list for Volume 3 = []
mask_mode for Volume 3 = 0
List overview for cryostat_wall with cylinder shape made of Al
LIST: Children for Volume 4 = [1,2,3,5]
LIST: Direct_children for Volume 4 = [5]
LIST: Intersect_check_list for Volume 4 = [5]
LIST: Mask_intersect_list for Volume 4 = []
LIST: Destinations_list for Volume 4 = [7]
LIST: Reduced_destinations_list for Volume 4 = [7]
LIST: Next_volume_list for Volume 4 = [7,5]
Is_vacuum for Volume 4 = 0
is_mask_volume for Volume 4 = 0
is_masked_volume for Volume 4 = 0
is_exit_volume for Volume 4 = 0
LIST: mask_list for Volume 4 = []
LIST: masked_by_list for Volume 4 = []
LIST: masked_by_mask_index_list for Volume 4 = []
mask_mode for Volume 4 = 0
List overview for cryostat_wall_vacuum with cylinder shape made of Vacuum
LIST: Children for Volume 5 = [1,2,3]
LIST: Direct_children for Volume 5 = [2,3]
LIST: Intersect_check_list for Volume 5 = [2,3]
LIST: Mask_intersect_list for Volume 5 = []
LIST: Destinations_list for Volume 5 = [4]
LIST: Reduced_destinations_list for Volume 5 = [4]
LIST: Next_volume_list for Volume 5 = [4,2,3]
Is_vacuum for Volume 5 = 1
is_mask_volume for Volume 5 = 0
is_masked_volume for Volume 5 = 0
is_exit_volume for Volume 5 = 0
LIST: mask_list for Volume 5 = []
LIST: masked_by_list for Volume 5 = []
LIST: masked_by_mask_index_list for Volume 5 = []
mask_mode for Volume 5 = 0
List overview for outer_cryostat_wall with cylinder shape made of Al
LIST: Children for Volume 6 = [1,2,3,4,5,7]
LIST: Direct_children for Volume 6 = [7]
LIST: Intersect_check_list for Volume 6 = [7]
LIST: Mask_intersect_list for Volume 6 = []
LIST: Destinations_list for Volume 6 = [0]
LIST: Reduced_destinations_list for Volume 6 = []
LIST: Next_volume_list for Volume 6 = [0,7]
Is_vacuum for Volume 6 = 0
is_mask_volume for Volume 6 = 0
is_masked_volume for Volume 6 = 0
is_exit_volume for Volume 6 = 0
LIST: mask_list for Volume 6 = []
LIST: masked_by_list for Volume 6 = []
LIST: masked_by_mask_index_list for Volume 6 = []
mask_mode for Volume 6 = 0
List overview for outer_cryostat_wall_vacuum with cylinder shape made of Vacuum
LIST: Children for Volume 7 = [1,2,3,4,5]
LIST: Direct_children for Volume 7 = [4]
LIST: Intersect_check_list for Volume 7 = [4]
LIST: Mask_intersect_list for Volume 7 = []
LIST: Destinations_list for Volume 7 = [6]
LIST: Reduced_destinations_list for Volume 7 = [6]
LIST: Next_volume_list for Volume 7 = [6,4]
Is_vacuum for Volume 7 = 1
is_mask_volume for Volume 7 = 0
is_masked_volume for Volume 7 = 0
is_exit_volume for Volume 7 = 0
LIST: mask_list for Volume 7 = []
LIST: masked_by_list for Volume 7 = []
LIST: masked_by_mask_index_list for Volume 7 = []
mask_mode for Volume 7 = 0
Union_master component master initialized sucessfully
Detector: logger_space_zx_I=725.995 logger_space_zx_ERR=5.11291 logger_space_zx_N=39720 "logger_zx.dat"
Detector: logger_space_zy_I=2798.37 logger_space_zy_ERR=16.4439 logger_space_zy_N=139936 "logger_zy.dat"
Detector: logger_1D_I=20536.8 logger_1D_ERR=33.5979 logger_1D_N=1.01065e+06 "logger_1D_time.dat"
Detector: abs_logger_space_zx_I=958.345 abs_logger_space_zx_ERR=1.3705 abs_logger_space_zx_N=1.01065e+06 "abs_logger_zx.dat"
Detector: logger_2DQ_I=20536.8 logger_2DQ_ERR=33.5979 logger_2DQ_N=1.01065e+06 "logger_2DQ.dat"
Detector: logger_2D_kf_I=20536.8 logger_2D_kf_ERR=33.5979 logger_2D_kf_N=1.01065e+06 "logger_2D_kf.dat"
Detector: logger_2D_space_time_I=11.7921 logger_2D_space_time_ERR=0.49868 logger_2D_space_time_N=598 "logger_2D_space_time.dat_0"
Detector: logger_2D_space_time_I=11.0386 logger_2D_space_time_ERR=0.490167 logger_2D_space_time_N=532 "logger_2D_space_time.dat_1"
Detector: logger_2D_space_time_I=200.713 logger_2D_space_time_ERR=2.01193 logger_2D_space_time_N=10409 "logger_2D_space_time.dat_2"
Detector: logger_2D_space_time_I=516.492 logger_2D_space_time_ERR=3.22147 logger_2D_space_time_N=26910 "logger_2D_space_time.dat_3"
Detector: logger_2D_space_time_I=5134.19 logger_2D_space_time_ERR=16.1373 logger_2D_space_time_N=249573 "logger_2D_space_time.dat_4"
Detector: logger_2D_space_time_I=9879.05 logger_2D_space_time_ERR=24.3829 logger_2D_space_time_N=478694 "logger_2D_space_time.dat_5"
Detector: logger_2D_space_time_I=1865.83 logger_2D_space_time_ERR=12.9248 logger_2D_space_time_N=90151 "logger_2D_space_time.dat_6"
Detector: logger_2D_space_time_I=484.641 logger_2D_space_time_ERR=4.49862 logger_2D_space_time_N=25342 "logger_2D_space_time.dat_7"
Detector: logger_2D_space_time_I=81.0748 logger_2D_space_time_ERR=2.03335 logger_2D_space_time_N=4440 "logger_2D_space_time.dat_8"
Detector: logger_2D_space_time_I=73.0366 logger_2D_space_time_ERR=1.99996 logger_2D_space_time_N=3432 "logger_2D_space_time.dat_9"
Detector: logger_2D_space_time_I=75.2637 logger_2D_space_time_ERR=2.00573 logger_2D_space_time_N=3672 "logger_2D_space_time.dat_10"
Detector: logger_2D_space_time_I=496.687 logger_2D_space_time_ERR=3.79769 logger_2D_space_time_N=26071 "logger_2D_space_time.dat_11"
Detector: logger_2D_space_time_I=507.125 logger_2D_space_time_ERR=3.88483 logger_2D_space_time_N=27047 "logger_2D_space_time.dat_12"
Detector: logger_2D_space_time_I=82.1685 logger_2D_space_time_ERR=2.50907 logger_2D_space_time_N=4680 "logger_2D_space_time.dat_13"
Detector: logger_2D_space_time_I=26.9562 logger_2D_space_time_ERR=1.23927 logger_2D_space_time_N=1620 "logger_2D_space_time.dat_14"
Detector: logger_2D_space_time_I=10.1797 logger_2D_space_time_ERR=0.77057 logger_2D_space_time_N=612 "logger_2D_space_time.dat_15"
Detector: logger_2D_space_time_I=4.55247 logger_2D_space_time_ERR=0.308599 logger_2D_space_time_N=325 "logger_2D_space_time.dat_16"
Detector: logger_2D_space_time_I=2.71127 logger_2D_space_time_ERR=0.224145 logger_2D_space_time_N=200 "logger_2D_space_time.dat_17"
Detector: logger_2D_space_time_I=1.07964 logger_2D_space_time_ERR=0.140208 logger_2D_space_time_N=75 "logger_2D_space_time.dat_18"
Detector: logger_2D_space_time_I=0.332187 logger_2D_space_time_ERR=0.0693423 logger_2D_space_time_N=29 "logger_2D_space_time.dat_19"
Detector: logger_2D_space_time_I=0.379245 logger_2D_space_time_ERR=0.0835753 logger_2D_space_time_N=26 "logger_2D_space_time.dat_20"
Detector: logger_2D_space_time_I=0.242421 logger_2D_space_time_ERR=0.0655867 logger_2D_space_time_N=21 "logger_2D_space_time.dat_21"
Detector: logger_2D_space_time_I=0.0826471 logger_2D_space_time_ERR=0.0322384 logger_2D_space_time_N=8 "logger_2D_space_time.dat_22"
Detector: logger_2D_space_time_I=0.131343 logger_2D_space_time_ERR=0.0504646 logger_2D_space_time_N=9 "logger_2D_space_time.dat_23"
Detector: logger_2D_space_time_I=0.0980249 logger_2D_space_time_ERR=0.0435544 logger_2D_space_time_N=6 "logger_2D_space_time.dat_24"
Detector: logger_2D_space_time_I=0.137031 logger_2D_space_time_ERR=0.0555056 logger_2D_space_time_N=10 "logger_2D_space_time.dat_25"
Detector: logger_2D_space_time_I=0.108223 logger_2D_space_time_ERR=0.0420662 logger_2D_space_time_N=10 "logger_2D_space_time.dat_26"
Detector: logger_2D_space_time_I=0.0440577 logger_2D_space_time_ERR=0.0244854 logger_2D_space_time_N=4 "logger_2D_space_time.dat_27"
Detector: logger_2D_space_time_I=0.0202494 logger_2D_space_time_ERR=0.0122366 logger_2D_space_time_N=3 "logger_2D_space_time.dat_28"
Detector: logger_2D_space_time_I=0.0121967 logger_2D_space_time_ERR=0.0142523 logger_2D_space_time_N=2 "logger_2D_space_time.dat_29"
Detector: logger_2D_space_time_I=0 logger_2D_space_time_ERR=0 logger_2D_space_time_N=0 "logger_2D_space_time.dat_30"
Detector: logger_2D_space_time_I=0.00765632 logger_2D_space_time_ERR=0.00765632 logger_2D_space_time_N=1 "logger_2D_space_time.dat_31"
Detector: logger_2D_space_time_I=0 logger_2D_space_time_ERR=0 logger_2D_space_time_N=0 "logger_2D_space_time.dat_32"
Detector: logger_2D_space_time_I=0 logger_2D_space_time_ERR=0 logger_2D_space_time_N=0 "logger_2D_space_time.dat_33"
Detector: logger_2D_space_time_I=0 logger_2D_space_time_ERR=0 logger_2D_space_time_N=0 "logger_2D_space_time.dat_34"
Detector: logger_2D_space_time_I=0 logger_2D_space_time_ERR=0 logger_2D_space_time_N=0 "logger_2D_space_time.dat_35"
INFO: Placing instr file copy python_tutorial.instr in dataset /Users/madsbertelsen/PaNOSC/McStasScript/github/McStasScript/docs/source/tutorial/data_folder/union_loggers_8
loading system configuration
Creating an animation¶
The plotter in McStasScript can create animations when supplied with many McStasData objects. We use name_search to find all the data from the relevant logger, and then a for loop to set plot options for each of them. Then the plotter can make an animation, which is saved as a gif.
ani_data = ms.name_search("logger_2D_space_time", data)
for frame in ani_data:
frame.set_plot_options(log=True, colormap="jet")
ms.make_animation(ani_data, filename="animation_demo", fps=2)
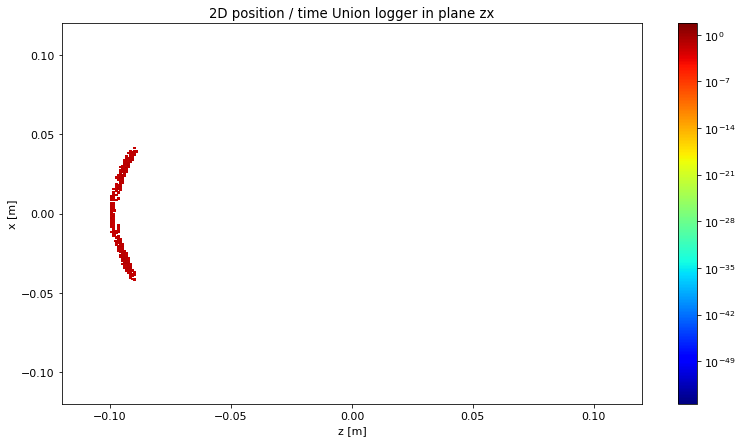
2023-04-25 15:03:41,010 WARNING:MovieWriter imagemagick unavailable; using Pillow instead.
Saving animation with filename : "animation_demo.gif"
Viewing the animation¶
Some problem in the jupyter notebook prevents playing the gif directly, but it can be played from markdown. One has to refresh this cell when a new animation is written. It should be visible that the beam enters the cryostat from the left, scatters of the sample and illuminates the entire cryostat. Running this simulation with a larger ncount and more time_bins in the monitor will reveal more details in what happens. This is available below, but commented out as the simulation can take some time.
Optional: Increasing resolution¶
Running this simulation with a larger ncount and more time_bins in the monitor will reveal more details in what happens. This is available below, but commented out as the simulation can take some time.
log_2D_st.time_bins = 128
instrument.settings(ncount=2E8, mpi=4)
#data = instrument.backengine()
#ani_data = ms.name_search("logger_2D_space_time", data)
#for frame in ani_data:
# ms.set_plot_options(log=True, colormap="jet", orders_of_mag=6)
#
#ms.make_animation(ani_data, filename="animation_demo_long", fps=5)
Viewing the animation¶
In the longer animation it is more evident that scattering from the aluminium hits the top and bottom of the outer cylinder.